java] Write a two-dimensional transformation library by implementing the following API: public class PolygonTransform { // Returns a new array object that is an exact copy of the given array. // The given array is not mutated. public static double[] copy(double[] array) // Scales the polygon by the factor alpha. public static void scale(double[] x, double[] y, double alpha) // Translates the polygon by (dx, dy). public static void translate(double[] x, double[] y, double dx, double dy) // Rotates the polygon theta degrees counterclockwise, about the origin. public static void rotate(double[] x, double[] y, double theta) // Tests each of the API methods by directly calling them. public static void main(String[] args)
java] Write a two-dimensional transformation library by implementing the following API: public class PolygonTransform { // Returns a new array object that is an exact copy of the given array. // The given array is not mutated. public static double[] copy(double[] array) // Scales the polygon by the factor alpha. public static void scale(double[] x, double[] y, double alpha) // Translates the polygon by (dx, dy). public static void translate(double[] x, double[] y, double dx, double dy) // Rotates the polygon theta degrees counterclockwise, about the origin. public static void rotate(double[] x, double[] y, double theta) // Tests each of the API methods by directly calling them. public static void main(String[] args)
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
100%
[java] Write a two-dimensional transformation library by implementing the following API:
public class PolygonTransform { // Returns a new array object that is an exact copy of the given array. // The given array is not mutated. public static double[] copy(double[] array) // Scales the polygon by the factor alpha. public static void scale(double[] x, double[] y, double alpha) // Translates the polygon by (dx, dy). public static void translate(double[] x, double[] y, double dx, double dy) // Rotates the polygon theta degrees counterclockwise, about the origin. public static void rotate(double[] x, double[] y, double theta) // Tests each of the API methods by directly calling them. public static void main(String[] args) }
![1. Polygon transform
polygon is defiıned by its sequence of vertices (xo, y o), (x 1, y 1), (x 2, Y 2), .... In Java, we will represent a polygon by storing the x- and y-
Write a library of static methods that performs various geometric transforms on polygons. Mathematically, a
coordinates of the vertices in two parallel arrays x[] and y[].
(1, 2)
(0, 1)
// Represents the polygon with vertices (0, 0), (1, 0), (1, 2), (0, 1).
double x[ ]
double y[]
{0, 1, 1, о };
{0, о, 2, 1 };
%3D
(0, 0)
(1, 0)
Three useful geometric transforms are scale, translate and rotate.
o Scale the coordinates of each vertex (x i, Y i) by a factor a.
Xi = a X¡
yi = a y
o Translate each vertex (x , Y ) by a given offset (dx, dy).
X; = X¡ + dx
Yi = Yi + dy
o Rotate each vertex (x ;, y ) by 0 degrees counterclockwise, around the origin.
X¡ = Xj Cos 0– y sin 0
Yi = Y cos e + x¡ sin 0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F801e7fe1-493b-4a36-a14c-4533a3782dfd%2Fc599bff4-06ab-41a1-b6f6-a51f40b05fba%2Fxf7j2oi_processed.png&w=3840&q=75)
Transcribed Image Text:1. Polygon transform
polygon is defiıned by its sequence of vertices (xo, y o), (x 1, y 1), (x 2, Y 2), .... In Java, we will represent a polygon by storing the x- and y-
Write a library of static methods that performs various geometric transforms on polygons. Mathematically, a
coordinates of the vertices in two parallel arrays x[] and y[].
(1, 2)
(0, 1)
// Represents the polygon with vertices (0, 0), (1, 0), (1, 2), (0, 1).
double x[ ]
double y[]
{0, 1, 1, о };
{0, о, 2, 1 };
%3D
(0, 0)
(1, 0)
Three useful geometric transforms are scale, translate and rotate.
o Scale the coordinates of each vertex (x i, Y i) by a factor a.
Xi = a X¡
yi = a y
o Translate each vertex (x , Y ) by a given offset (dx, dy).
X; = X¡ + dx
Yi = Yi + dy
o Rotate each vertex (x ;, y ) by 0 degrees counterclockwise, around the origin.
X¡ = Xj Cos 0– y sin 0
Yi = Y cos e + x¡ sin 0
![Note that the transformation methods scale(), translate() and rotate() mutate the polygons. Here are some example test cases (tests
for copy () are not shown):
// Scales polygon by the factor 2.
StdDraw.setScale(-5.0, +5.0);
double[] x = { 0, 1, 1, 0 };
double[] y = { 0, 0, 2, 1 };
double alpha
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
scale (x, y, alpha);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
|// Translates polygon by (2, 1).
StdDraw.setScale(-5.0, +5.0);
double[] x =
double[] y = { 0, 0, 2, 1 };
double dx = 2.0, dy
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
translate(x, y, dx, dy);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
|// Rotates polygon 45 degrees.
StdDraw.setScale(-5.0, +5.0);
double[] x = { 0, 1, 1, 0 };
double[] y = { 0, 0, 2, 1 };
double theta = 45. 0;
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
rotate(x, y, theta);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
{ 0, 1, 1, 0 };
= 2.0;
= 1.0;
(0, 0)
(0, 0)
(0, 0)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F801e7fe1-493b-4a36-a14c-4533a3782dfd%2Fc599bff4-06ab-41a1-b6f6-a51f40b05fba%2Fnznolim_processed.png&w=3840&q=75)
Transcribed Image Text:Note that the transformation methods scale(), translate() and rotate() mutate the polygons. Here are some example test cases (tests
for copy () are not shown):
// Scales polygon by the factor 2.
StdDraw.setScale(-5.0, +5.0);
double[] x = { 0, 1, 1, 0 };
double[] y = { 0, 0, 2, 1 };
double alpha
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
scale (x, y, alpha);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
|// Translates polygon by (2, 1).
StdDraw.setScale(-5.0, +5.0);
double[] x =
double[] y = { 0, 0, 2, 1 };
double dx = 2.0, dy
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
translate(x, y, dx, dy);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
|// Rotates polygon 45 degrees.
StdDraw.setScale(-5.0, +5.0);
double[] x = { 0, 1, 1, 0 };
double[] y = { 0, 0, 2, 1 };
double theta = 45. 0;
StdDraw.setPenColor(StdDraw.RED);
StdDraw.polygon(x, y);
rotate(x, y, theta);
StdDraw.setPenColor(StdDraw.BLUE);
StdDraw.polygon(x, y);
{ 0, 1, 1, 0 };
= 2.0;
= 1.0;
(0, 0)
(0, 0)
(0, 0)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
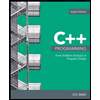
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
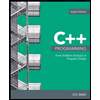
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning