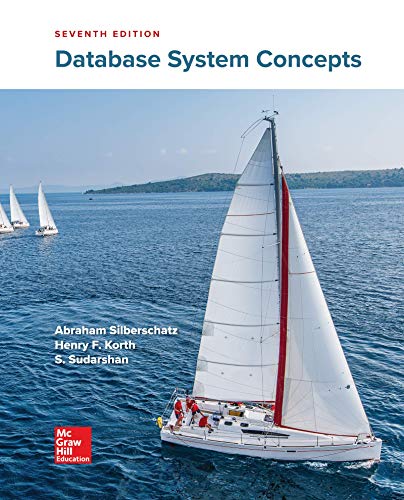
Concept explainers
LAB 5.3 Working with the for Loop
Bring in
the Pre-lab Reading Assignment). This program has the user input a number n and
then finds the mean of the first n positive integers. The code is shown below:
// This program has the user input a number n and then finds the
// mean of the first n positive integers
// PLACE YOUR NAME HERE
#include <iostream>
using namespace std;
int main()
{
int value; // value is some positive number n
int total = 0; // total holds the sum of the first n positive numbers
int number; // the amount of numbers
float mean; // the average of the first n positive numbers
cout << "Please enter a positive integer" << endl;
cin >> value;
if (value > 0)
{
for (number = 1; number <= value; number++)
{
total = total + number;
}// curly braces are optional since there is only one statement
mean = static_cast<float>(total) / value; // note the use of the typecast
// operator here
cout << "The mean average of the first " << value
<< " positive integers is " << mean << endl;
}
else
cout << "Invalid input - integer must be positive" << endl;
return 0;
}
Exercise 1: Why is the typecast operator needed to compute the mean in the
statement mean = static_cast(float)(total)/value;? What do you think
will happen if it is removed? Modify the code and try it. Record what happens.
Make sure that you try both even and odd cases. Now put static_cast<float>
total back in the program.
Exercise 2: What happens if you enter a float such as 2.99 instead of an integer
for value? Try it and record the results.
Exercise 3: Modify the code so that it computes the mean of the consecutive
positive integers n, n+1, n+2, . . . , m, where the user chooses n and m.
For example, if the user picks 3 and 9, then the program should find the
mean of 3, 4, 5, 6, 7, 8, and 9, which is 6.

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 14 images

- PrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardCan this be completed using a while loop at least once or twicearrow_forwardThis program in C++ obtains a series of letter grades as input from a user. The program should calculate and output the total grade points and the grade point average (note: you may have to track the total number of inputs and the grade points to calculate the grade point average). The algorithm should execute 3 times for testing purposes.Use the appropriate loop for the main structure of the program that will stop accepting letter grades when the user inputs X. Also, choose the appropriate selection statement to convert the letter grade to the correct grade points.Additional Requirements:• Use appropriate data types and variable names throughout the code.• Use constants appropriately. Output needs to look like the image below.arrow_forward
- How do I write the while loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop? Also including the calculations to compute the average rating.arrow_forwardin phython langage Write a while loop that will print all the numbers between 200 and 300 (200 and 300 included) that are divisible by 3 but not divisible by 6. Your output should include the following numbers: 201 207 213 219 225 231 237 243 249 255 261 267 273 279 285 291 297arrow_forward12. Write a loop of the specified type that sums up the even integers between 1 and 21. a. a while loop b. a for looparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
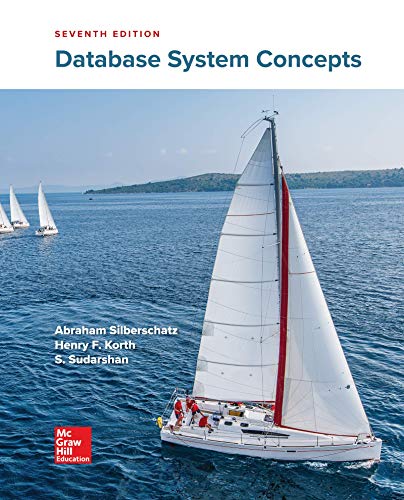
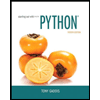
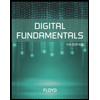
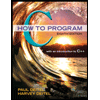
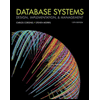
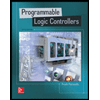