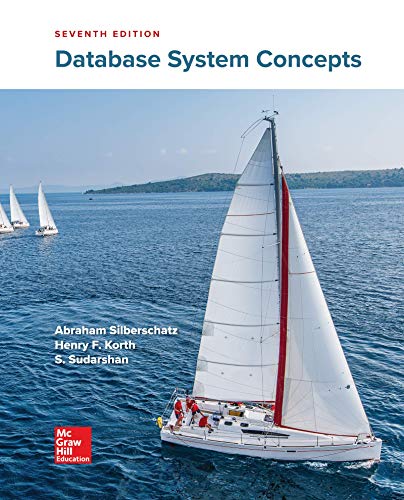
import java.util.Scanner;
public class CircleAndSphereWhileLoop
{
public static final double MAX_RADIUS = 500.0;
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
// Step 2: Read a double value as radius using prompt
// "Enter the radius (between 0.0 and 500.0, exclusive): "
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
// Display a message on one line (ssuming input value -1)
// "The input number -1.00 is out of range."
// Read a double value as radius using the same promt
double circumference = 2 * Math.PI * radius;
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3);
// Step 4: Display the radius, circle circumference, circle area,
// sphere surface area, and sphere volume on separate
// lines with 6 decimal digits
// Sample output
// The radius : 499.990000.
// The circle circumference: 3141.529822.
// The circle area : 785366.747785.
// The sphere surface area : 3141466.991100.
// The sphere volume : 523567360.300077.
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Java Programming Language The math to calculate the area for scalene, isoceles, and equlateral triangles are apparently wrong. Only the calculations for the right triange are correct. I need the correct calculations for the area of the other triangles. This is my program: import java.util.Scanner;public class TriangleClassifier{public static void main(String[] args){Scanner Keyboard = new Scanner(System.in);int side1, side2, side3;boolean valid = true;System.out.println("Welcome to the Triangle Classifier Program");System.out.println("Enter the lengths of the three (3) triangle sides"+ " in ascending order >>");side1 = Keyboard.nextInt(); // I don't know why, but you must enter the values a second timeside2 = Keyboard.nextInt(); // for the program to start.side3 = Keyboard.nextInt();if (Keyboard.hasNextInt()){side1 = Keyboard.nextInt();}else{Keyboard.next();valid = false;}if (!valid){System.out.println("Error - At least one of your sides is not an integer");return;}if…arrow_forwardimport java.util.*;import java.io.FileWriter;import java.io.IOException;public class Main { public static void main(String[] args) throws IOException { //For taking inputs Scanner in = new Scanner(System.in); ArrayList<Integer> daysEntered = new ArrayList<Integer>(); // For storing dangerous reading value int dangerous; // Ask and input the reading that is considered dangerous System.out.print("Enter dangerous reading: "); dangerous = in.nextInt(); // To write in file FileWriter myWriter = new FileWriter("report.txt"); // To store day of the month int day = 1; // To store the three readings in a day int first_reading, second_reading, third_reading; // Loops until user input 0 as day while(day…arrow_forwardimport java.util.*; public class Main{ public static void main(String[] args) { Scanner sc= new Scanner(System.in); int a1= sc.nextInt(); int b1=sc.nextInt() ; int c1=b1/2; int i =1 ; while(i<=c1){ for( int j=1 ; j<=c1-i ; j++){ System.out.print(" "); } for (int k=1; k<=a1; k++){ System.out.print("="); } System.out.print("\n"); a1=a1+2; i++; } } } a) provide commented code for the following codearrow_forward
- Write a for loop that prints: 1 2 … countNum Ex: If the input is: 4 the output is: 1 2 3 4arrow_forwardWrite a statement that outputs variable userAge. End with a newline. Program will be tested with different input values. import java.util.Scanner; public class VariableOutput {public static void main (String [] args) {int userAge; Scanner scnr = new Scanner(System.in);userAge = scnr.nextInt(); // Program will be tested with values: 15, 40.arrow_forwardWrite a for loop that prints from startNumber to lastNumber. Ex: If the input is: -3 1 the output is: -3 -2 -1 0 1 1 import java.util.Scanner; 2 3 public class ForLoops { public static void main (String [] args) { int startNumber; int lastNumber; int i; 5 6 7 Scanner input new Scanner(System.in); input.nextInt(); input.nextInt(); 9. %3D 10 startNumber 11 lastNumber %3D 12 for (* Your code goes here */) { System.out.print(i + } 13 14 "); 15 16arrow_forward
- import java.util.Scanner;import java.util.NoSuchElementException; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int val1; int val2; int val3; int max; val1 = 0; val2 = 0; val3 = 0; /* Type your code here. */ }}arrow_forwardFind the internal representation of the integer: 450 (in 32-bit memory location). Please, do not insert any blanks in your answer.arrow_forwardimport java.util.*; public class Main{ public static void main(String[] args) { Scanner sc= new Scanner(System.in); int a1= sc.nextInt(); int b1=sc.nextInt() ; int c1=b1/2; int i =1 ; while(i<=c1){ for( int j=1 ; j<=c1-i ; j++){ System.out.print(" "); } for (int k=1; k<=a1; k++){ System.out.print("="); } System.out.print("\n"); a1=a1+2; i++; } } } Explain in your own words how one important method of your program above works.arrow_forward
- Please write in Java import java.util.Scanner; public class ConcertPromoter { public static void main(String[] args) { Scanner key = new Scanner(System.in); Concert concert = new Concert(); System.out.println("Welcome to the Concert Promotion tool!"); String input = ""; while(input.equalsIgnoreCase("quit")!= true) { System.out.println("Currently the concert featuring the band: "+concert.getBandName()); System.out.println("Has sold "+concert.getNumTicketsSoldByPhone()+" tickets by phone"); System.out.println("Has sold "+concert.getNumTicketsSoldAtVenue()+" tickets at the venue"); System.out.println("And has grossed $"+concert.totalSales()); System.out.println("What would you like to do?\n" + "Enter 1: To change name\n" + "Enter 2: To change ticket by phone price\n" + "Enter 3: To change ticket at venue price\n" + "Enter 4: To add tickets by phone\n" + "Enter 5: To add tickets at the venue\n" + "Enter…arrow_forwardI have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) {…arrow_forwardPython language Write a initializer method for the class Test. It has 2 parameters/field variables: correct and totalarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
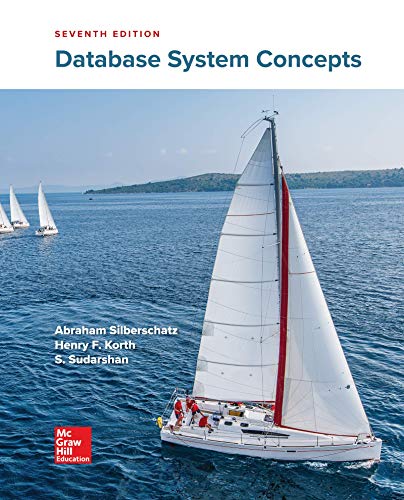
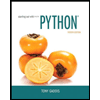
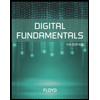
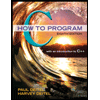
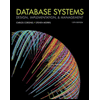
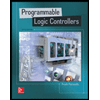