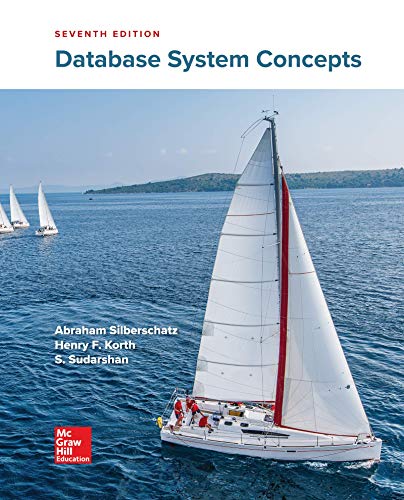
Concept explainers
My Python code has an attribute error wherein 'Car' has no attribute 'accelerate' attached to it, but it is clearly defined in my code. Can you help please? My code is below.
class Car:
def __init__(self, model_year, make):
self.__model_year = model_year
self.__make = make
self.__speed = 0
def accelerate(self):
self.__speed += 3
def brake(self):
self.__speed -= 3
def get_speed(self):
return self.__speed
# Make Car object
my_subaru = Car(2015, "Subaru Forester")
# Accelerate car 5x and show speed after each acceleration
for _ in range(5):
my_subaru.accelerate()
the_speed = my_subaru.get_speed()
print(f"Current Speed is: {the_speed} mph.")
# Brake the car 5x and show speed after each brake
for _ in range(5):
my_subaru.brake()
the_speed = my_subaru.get_speed()
print(f"Current Speed is: {the_speed} mph.")

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- fast in pyth pleasearrow_forward1 Java onlyarrow_forwardwrite a python code Create the Testing class that does the following: a-Use the constructor to create an instance of Cylinder class cy with radius of 5 and height of 10. use the following code: class Circular_Shape_3D: radius = 0.0 #double value #Constructor def __init(self,radius): self.radius = radius #Get function def getRadius(self): return self.radius #Set function def setRadius(self, radius): self.radius = radius #Print function def Print(self): print("Circular_Shape_3D") print("Radius:", self.radius) class Cylinder(Circular_Shape_3D): #Radius value is already present in super class Circular_Shape_3D height = 0.0 #double value #Constructor def __init(self,radius,height): super().__init__(radius) self.height = height #Get function def getHeight(self): return self.height #Set function def setHeight(self, height): self.height = height…arrow_forward
- python: class Student:def __init__(self, first, last, gpa):self.first = first # first nameself.last = last # last nameself.gpa = gpa # grade point average def get_gpa(self):return self.gpa def get_last(self):return self.last class Course:def __init__(self):self.roster = [] # list of Student objects def add_student(self, student):self.roster.append(student) def course_size(self):return len(self.roster) # Type your code here if __name__ == "__main__":course = Course()course.add_student(Student('Henry', 'Nguyen', 3.5))course.add_student(Student('Brenda', 'Stern', 2.0))course.add_student(Student('Lynda', 'Robison', 3.2))course.add_student(Student('Sonya', 'King', 3.9)) student = course.find_student_highest_gpa()print('Top student:', student.first, student.last, '( GPA:', student.gpa,')')arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forwardIn C++ Create a new project named lab9_1 . You will need to implement a Course class. Here is its UML diagram: Course - department : string- course_num : string- section : int- num_students : int- is_full : bool + Course()+ Course(string, string, int, int)+ setDepartment(string) : void+ setNumber(string) : void+ setSection(int) : void+ setStudents(int) : void+ getDepartment() const : string+ getNumber() const : string+ getSection() const : int+ getStudents() const : int+ print() const : void Create a sample file to read from: CSS 2A 1111 35 Additional information: The Course class has two constructors. Make sure you have default values for your default constructor. Each course maxes out at 40 students. Therefore, you need to make sure that there aren’t more than 40 students in a Course. You can choose how you handle situations where more than 40 students are added. Additionally, you should automatically set is_full to false or true, based on the number of…arrow_forward
- In C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forwardcreate a class in python with a constructor To create instances of the user defined class and practice applying them. Practice implementing loops. Part 1 Instructions:Create a class called BankAccount with the following variables: balancenameaccount_id Create 1 constructor for the class BankAccount (with or without parameters, your choice, but only 1): def __init_(self, name, account_id, balance)def __init_(self) Create the following methods in the BankAccount class: def viewAccountInfo() //prints all account information def withdraw(self, amount) //withdraws from the balance def deposit(self, amount) //deposits to the balance Your task after your class has been created: Create two objects, "personal" and "joint_account"with initial balances of:personal = $1000joint_account = $3000Use the constructor to create the objects. Create a program that prompts the user which account they would like to access personal or jointWhich account would you like to access? 1. Personal2. Joint…arrow_forwardHello, I have written my random bear generator but for some reason the code isn't running in my command terminal. Please have a look at it and let me know what I am missing. I followed my teachers instructors and lectures, but it doesn't run on another compiler either. class Bear { constructor(type, color, weight, favoriteFood) { this.type = type; this.color = color; this.weight = weight; this.favoriteFood = favoriteFood; if (this.weight === 700 || this.type === "Little Bear" || this.type === "Baby Bear") { this.isAggressive = false; } else if (this.weight === 1200 || this.type === "Papa Bear" || this.type === "Black Bear") { this.isAggressive = true; } else { this.isAggressive = Math.random() < 0.5; } if (this.type === "Winnie the Pooh Bear" && this.favoriteFood !== "Honey") { throw new Error("Winnie the Pooh Bear must have Honey as his favorite food."); } }} class BearGenerator { get types() { return [ "Winnie…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
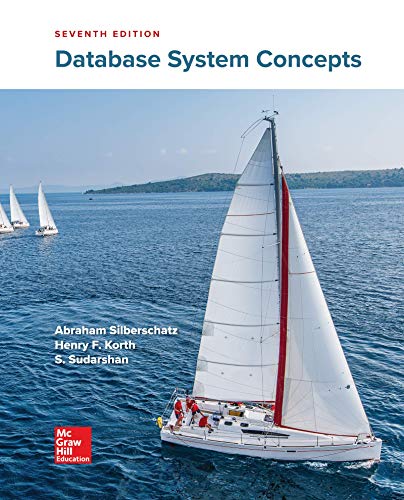
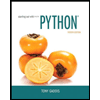
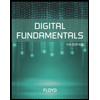
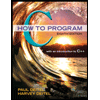
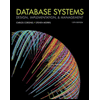
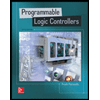