# NumberFun.py # using multiple functions. # copy/paste this program to run it first, you are supposed to use Google Chrome as Internet Browser for this course. # 1. calculate sum of the first n natural numbers, e.g.:1,2,3,... def totalN(n): totalN = 0 for i in range(1,n+1): totalN = totalN + i return totalN # 2. calculate sum of the cube of the first m natural numbers, e.g.:1,8,27,... ### After you define/write/complete the function totalMCube(m), remove the # sign as below to ENABLE next line of code ###def totalMCube(m): ### YOUR TURN TO DEFINE/WRITE/COMPLETE function totalMCube(m) as below, based on totalN(n) # 3. call two functions defined previously within main() function def main(): print("This program computes the total and total of cubes of the first") print("N/M natural numbers.\n") n,m = input("Please enter a value for N and M: ").split(",") print("The total of the first", n, "natural numbers is", totalN(int(n))) ### After you define/write/complete the function totalMCube(m), remove the # sign as below to ENABLE next line of code ###print("The total of the cubes of the first", m, "natural numbers is", (totalMCube(int(m)))) main()
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
# NumberFun.py # using multiple functions. # copy/paste this program to run it first, you are supposed to use Google Chrome as Internet Browser for this course.
# 1. calculate sum of the first n natural numbers, e.g.:1,2,3,... def totalN(n): totalN = 0 for i in range(1,n+1): totalN = totalN + i return totalN
# 2. calculate sum of the cube of the first m natural numbers, e.g.:1,8,27,... ### After you define/write/complete the function totalMCube(m), remove the # sign as below to ENABLE next line of code ###def totalMCube(m):
### YOUR TURN TO DEFINE/WRITE/COMPLETE function totalMCube(m) as below, based on totalN(n)
# 3. call two functions defined previously within main() function def main(): print("This program computes the total and total of cubes of the first") print("N/M natural numbers.\n") n,m = input("Please enter a value for N and M: ").split(",") print("The total of the first", n, "natural numbers is", totalN(int(n)))
### After you define/write/complete the function totalMCube(m), remove the # sign as below to ENABLE next line of code ###print("The total of the cubes of the first", m, "natural numbers is", (totalMCube(int(m)))) main()
-----------------
2. Don't delete the statements/codes/comments for this program, but just add more statements for the second function. (if deleted, points will be deducted.)
3. The purpose of this question is to use multiple functions for

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

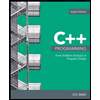
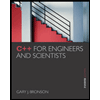
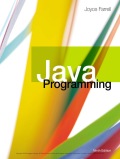
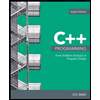
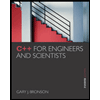
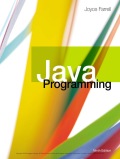