package Q2; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; public class Question2 { public static void main(String[] args) throws FileNotFoundException { /** * Part a * Finish creating an ArrayList called NameList that stores the names in the file Names.txt. */ ArrayList NameList = new ArrayList(); /** * Part b * Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream * so that it is initialized correctly to the Names.txt file located in the folder specified in the question description */ FileInputStream inputStream = new FileInputStream("Names.txt"); Scanner scnr = new Scanner(inputStream); //Do not modify this line of code /** * Part c * Using a loop and the Scanner object provided, read the names from Names.txt * and store them in NameList created in Part a. */ while (scnr.hasNext()) { //reading each string from text file and adding to NameList NameList.add(scnr.next()); } /** * Part d * Reorder the names in the ArrayList so that they appear in reverse alphabetical order. */ for (int i = 0; i < NameList.size(); i++) { //Using bubble sort algorithm for (int j = 0; j < NameList.size() - i - 1; j++) { //comparing NameList[i] and NameList[i+1] if (NameList.get(j).compareTo(NameList.get(j + 1)) > 0) { //swapping NameList[i] and NameList[i+1] String temp = NameList.get(j); NameList.set(j, NameList.get(j + 1)); NameList.set(j + 1, temp); } } // System.out.println("NameList after correct ordering: " + NameList); //Uncomment when you are ready to test Part d /** * Part e * Change the spelling of the name "Petor" to "Peter" in *NameList*. */ for (int i = 0; i < NameList.size(); i++) { //Incase Petor is found if (NameList.get(i).compareTo("Petor") == 0) NameList.set(i, "Peter"); } System.out.println("NameList after corrected spelling: " + NameList); //Uncomment when you are ready to test Part e } } } INPUT FILE: Petor Winston Laura Bonnie
package Q2;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
public class Question2 {
public static void main(String[] args) throws FileNotFoundException {
/**
* Part a
* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.
*/
ArrayList<String> NameList = new ArrayList<String>();
/**
* Part b
* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream
* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description
*/
FileInputStream inputStream = new FileInputStream("Names.txt");
Scanner scnr = new Scanner(inputStream); //Do not modify this line of code
/**
* Part c
* Using a loop and the Scanner object provided, read the names from Names.txt
* and store them in NameList created in Part a.
*/
while (scnr.hasNext()) {
//reading each string from text file and adding to NameList
NameList.add(scnr.next());
}
/**
* Part d
* Reorder the names in the ArrayList so that they appear in reverse alphabetical order.
*/
for (int i = 0; i < NameList.size(); i++) {
//Using bubble sort
for (int j = 0; j < NameList.size() - i - 1; j++) {
//comparing NameList[i] and NameList[i+1]
if (NameList.get(j).compareTo(NameList.get(j + 1)) > 0) {
//swapping NameList[i] and NameList[i+1]
String temp = NameList.get(j);
NameList.set(j, NameList.get(j + 1));
NameList.set(j + 1, temp);
}
}
// System.out.println("NameList after correct ordering: " + NameList); //Uncomment when you are ready to test Part d
/**
* Part e
* Change the spelling of the name "Petor" to "Peter" in *NameList*.
*/
for (int i = 0; i < NameList.size(); i++) {
//Incase Petor is found
if (NameList.get(i).compareTo("Petor") == 0)
NameList.set(i, "Peter");
}
System.out.println("NameList after corrected spelling: " + NameList); //Uncomment when you are ready to test Part e
}
}
}
INPUT FILE:
Petor
Winston
Laura
Bonnie

Step by step
Solved in 2 steps

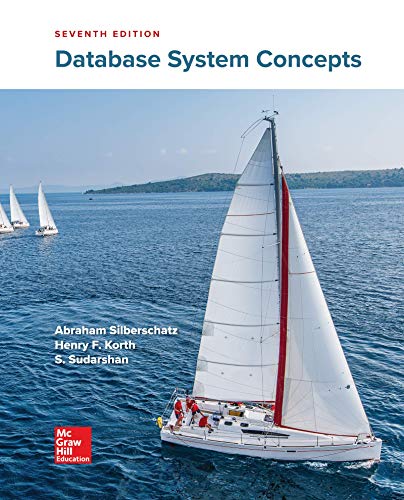
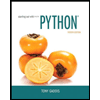
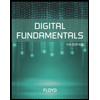
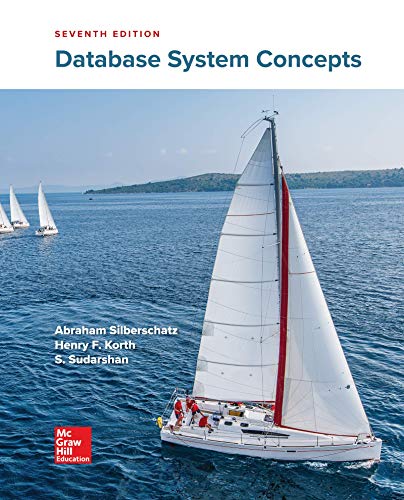
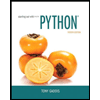
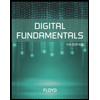
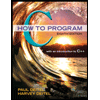
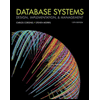
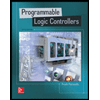