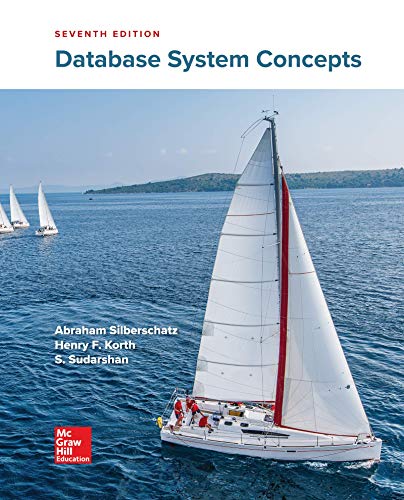
Concept explainers
Please create a Java class that has the following data attributes and methods:
private int count - number of customers in the array
private customer Record[] data - array of customerRecord objects
public customer List() constructor that should initialize memory for data array and count value
public void getCustomerList(String fileName) - reads a file call fileName which is a text file containing lines (records) of customer data. This method fills the data array with the records from the file. The file will not have more than 100 records and will have the following format (where customer Number is an integer, firstName and lastName are Strings, and balance is a float:
customerNumber firstName lastName balance
public customerRecord getCustomer(int customerNumber) - returns the object corresponding to the customer with customerNumber. If the customer number is not in the array, return null.
public void enter CustomerRecord(customerRecord new_record) - store the customer record into data array
public void saveCustermerList(String filename) - save the information stored in the data array to the file called filename

Step by stepSolved in 2 steps

- In C++ Write a code snippet to automatically increase the size of the dynamic array prayerBox[10] if it becomes full while reading data in from a file.arrow_forwardWorkArea 1st object 2nd object 3rd object 1- Write the code that declares an array of Rectangle objects, called rectangles, and stores in it three objects with the following data: length width 20 25 30 10 15 30 Area of object 1: 200 Area of object 2: 375 Area of object 3: 900 Interactive Session ? 2- Write the code of a for loop that displays the areas of n objects from the rectangles array you declared in the previous task. The output of the for loop. when n = 3, should look like this: Note: Make sure to use the integer n in your for loop. Workbench Additional Notes: Regarding your code's standard output, CodeLab will ignore case errors and will ignore whitespace (tabs, spaces, newlines) altogether. Visible newlines 0 00 0? 0! 0x 1- Visible whitespace MacBook Air Display stdin ✔Display stdout Millarrow_forwardPythonarrow_forward
- Design an application that declares an array of 20 AutomobileLoan objects. Prompt the user for data for each object, and then display all the values.arrow_forwardJAVA PROGRAM Lab #2. Chapter 7. PC #11. Array Operations (Page 491) Write a program that accepts a file name from command line, then initializes an array with test data using that text file as an input. The file should contain floating point numbers (use double data type). The program should also have the following methods: * getTotal. This method should accept a one-dimensional array as its argument and return the total of the values in the array. * getAverage. This method should accept a one-dimensional array as its argument and return the average of the values in the array. * getHighest. This method should accept a one-dimensional array as its argument and return the highest value in the array. * getLowest. This method should accept a one-dimensional array as its argument and return the lowest value in the array. This part of the program is not correct. There should be no Scanner. You should read the file name from the command line.Scanner scanner = new…arrow_forwardjavaarrow_forward
- JAVA PROGRAM Chapter 7. PC #16. 2D Array Operations with Additional Requirements Write a program that creates an ArrayList of ArrayList of Doubles. The program should ask the user to enter the filename from the keyboard and validate the file for existence. Once the file is verified, the program should load the two-dimensional ArrayList with test data from the file. Be careful, as some of the files will contain rows with different number of elements. The program should work regardless whether the input data is perfectly rectangular or ragged. The program should have the following methods: • main. Main entry point for the program. • getRowSubtotal. This method should accept a two-dimensional ArrayList as its first argument and an integer as its second argument. The second argument should be the index of the row in the ArrayList. The method should return the subtotal of the values in the specified row. • getColSubtotal. This method should accept a two-dimensional ArrayList as…arrow_forwardJAVA PROGRAM Lab #2. Chapter 7. PC #11. Array Operations (Page 491) Write a program that accepts a file name from command line, then initializes an array with test data using that text file as an input. The file should contain floating point numbers (use double data type). The program should also have the following methods: * getTotal. This method should accept a one-dimensional array as its argument and return the total of the values in the array. * getAverage. This method should accept a one-dimensional array as its argument and return the average of the values in the array. * getHighest. This method should accept a one-dimensional array as its argument and return the highest value in the array. * getLowest. This method should accept a one-dimensional array as its argument and return the lowest value in the array. double_input1.txt double_input2.txt WHEN I UPLOAD THE PROGRAM. TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. PLEASE MODIFY THIS CODE, SO WHEN I…arrow_forwardJAVA PROGRAM Lab #2. Chapter 7. PC #11. Array Operations (Page 491) Write a program that accepts a file name from command line, then initializes an array with test data using that text file as an input. The file should contain floating point numbers (use double data type). The program should also have the following methods: * getTotal. This method should accept a one-dimensional array as its argument and return the total of the values in the array. * getAverage. This method should accept a one-dimensional array as its argument and return the average of the values in the array. * getHighest. This method should accept a one-dimensional array as its argument and return the highest value in the array. * getLowest. This method should accept a one-dimensional array as its argument and return the lowest value in the array. PLEASE MOFDIFY THIS PROGRAM SO THERE ARE ONLY THREE DIGITS AFTER THE DECIMAL POINT FOR LOWEST, HIGHTEST, AVERAGE AND TOTAL. BECAUSE WHEN I UPLOAD IT TO…arrow_forward
- Can you fix the code please on the first picture shows the error output. // Corrected code #define _CRT_SECURE_NO_WARNINGS #include "LibraryManagement.h" #include "Books.h" #include "DigitalMedia.h" #include "LibraryConfig.h" #include #include #include #include // Include the necessary header for boolean data type // Comparison function for qsort to sort Digital Media by ID int compareDigitalMedia(const void* a, const void* b) { return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id; } // initializing library struct Library initializeLibrary() { struct Library lib; lib.bookCount = 0; lib.ebookCount = 0; lib.digitalMediaCount = 0; // Initialize book array for (int i = 0; i < MAX_BOOK_COUNT; i++) { lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot } // Initialize ebook array for (int i = 0; i < MAX_EBOOK_COUNT; i++) { lib.ebooks[i].commonAttributes.id = -1; }…arrow_forwardGiven the previous Car class, the following members have been added for you: Private: string * parts; //string array of part names int num_parts; //number of parts Public: void setParts(int numpart, string newparts[]) //set the numparts and parts int getNumParts() string * getParts() string getPart(int index) //return the string in parts at index num Please implement the following for the Car class: Add the copy constructor. (deep copy!) Add the copy assignment operator. (deep copy!) Add the destructorarrow_forwardIn Java please,arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
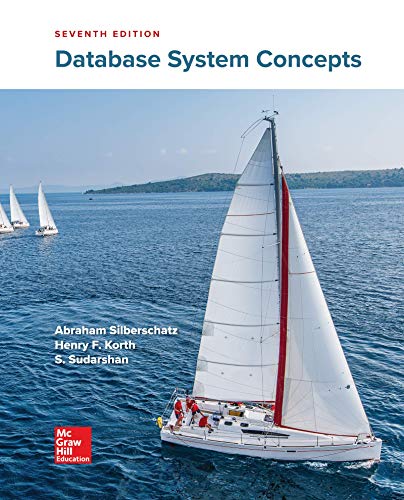
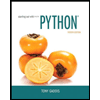
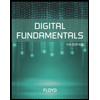
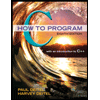
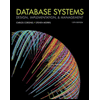
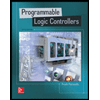