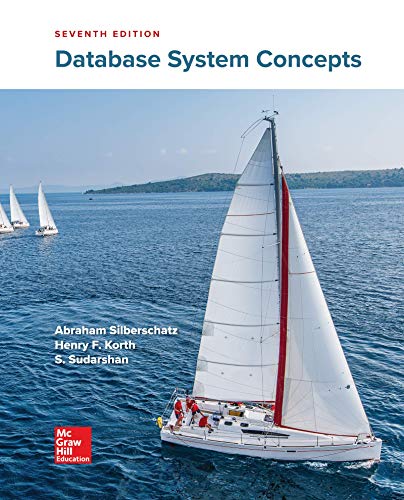
Concept explainers
Please help me with my Python code Im still getting errors like missing input data and more.
def read_data(filename):
try:
with open(filename, "r") as file:
return file.read()
except Exception as exception:
print("File is empty" if not str(exception) else str(exception))
return None
def extract_data(tags, strings):
data = []
start_tag = f"<{tags}>"
end_tag = f"</{tags}>"
while start_tag in strings:
try:
start_index = strings.find(start_tag) + len(start_tag)
end_index = strings.find(end_tag)
if end_index == -1:
break
value = strings[start_index:end_index]
if value:
data.append(value)
strings = strings[end_index + len(end_tag):]
except Exception as exception:
print(exception)
return data
def get_names(string):
names = extract_data("name", string)
return names
def get_descriptions(string):
descriptions = extract_data("description", string)
return descriptions
def get_calories(string):
calories = extract_data("calories", string)
return calories
def get_prices(string):
prices = extract_data("price", string)
return prices
def display_menu(names, descriptions, calories, prices):
for i in range(len(names)):
print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}")
def display_stats(calories, prices):
total_items = len(calories)
average_calories = sum(map(int, calories)) / total_items
correct_price = []
for price in prices:
try:
if price:
correct_price.append(float(price[1:]))
else:
correct_price.append(0.0)
except ValueError:
print("Error: Missing or bad data")
return
average_price = sum(correct_price) / total_items
print(f"{total_items} items - {average_calories:.1f} average calories - ${average_price:.2f} average price")
def main():
filename = "simple.xml"
string = read_data(filename)
if string:
names = get_names(string)
descriptions = get_descriptions(string)
calories = get_calories(string)
prices = get_prices(string)
if names and prices and descriptions and calories:
display_menu(names, descriptions, calories, prices)
display_stats(calories, prices)
main()
![<
X Test with pytest
5 items 800.0 average calories $6.86 average price
448 E
449
450 .github/workflows/test.py: 1393: AssertionError
451
452
short test summary info
FAILED .github/workflows/test_final_project.py::test_final_project_source_code_formatting
formatting:
453 Final project.py:82:80: E501 line too long (112 > 79 characters)
454
FAILED .github/workflows/test_final_project.py::test_final_project_missing_file
incorrect. Expected 'File is missing'.
AssertionError: Final Project Final Project Python source code
455 Input:
456 Missing file
457 Output:
458 [Errno 2] No such file or directory: 'simple.xml'
459 FAILED .github/workflows/test_final_project.py::test_final_project_empty_file - AssertionError: Final Project Final Project Error message is missing or
incorrect. Expected 'File is empty'.
Input:
Empty file
464 Input:
465 No records
466 Output:
467 FAILED .github/workflows/test_final_project.py::test_final_project_missing_fields
incorrect. Expected 'Error: Missing or bad data'.
AssertionError: Final Project Final Project Error message is missing or
460
461
462 Output:
463 FAILED .github/workflows/test_final_project.py::test_final_project_no_records AssertionError: Final Project Final Project Error message is missing or
incorrect. Expected 'File is empty' or 'No data'.
-
AssertionError: Final Project Final Project Error message is missing or
468 Input:
469 Missing fields
470
Output:
471
Belgian Waffles Two of our famous Belgian Waffles with plenty of real maple syrup 650 - $5.95
472 Strawberry Belgian Waffles Light Belgian waffles covered with strawberries and whipped cream 900 - $7.95
473
Berry-Berry Belgian Waffles - Light Belgian waffles covered with an assortment of fresh berries and whipped cream 900 $8.95
Homestyle Breakfast Thick slices made from our homemade sourdough bread 600 $4.50
474
475 5 items
800.0 average calories
$6.86 average price
476
========= 5 failed, 46 passed, 35 skipped in 1.26s
477 Error: Process completed with exit code 1.
1s](https://content.bartleby.com/qna-images/question/df788c22-83be-4a9a-a241-87bf44363afe/8f476349-a518-4810-bec7-a89b46f7ef7e/lpm2t3k_thumbnail.png)

Step by stepSolved in 4 steps with 2 images

- FilelO 01: Write a Message Write a FileI001 class with a method named writeMessage() to takes message as a String and a filename (as a String) (in that order). Have the method, write the message to the filename. Your code will either need to 1) catch all checked exceptions or 2) use the throws keyword. public class FileI001{ public void writeMessage( String message, String filename ){arrow_forwardJava : Create a .txt file with 3 rows of various movies data of your choice in the following table format: Movie ID number of viewers rating release year Movie name 0000012211 174 8.4 2017 "Star Wars: The Last Jedi" 0000122110 369 7.9 2017 "Thor: Ragnarok" Create a class Movie which instantiates variables corresponds to the table columns. Implement getters, setters, constructors and toString. Implement 2 readData() methods : the first one will return an array of Movies and the second one will return a List of movies . Test your methods.arrow_forwardFilename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forward
- -Add a method to the tractor class to write all of atractors attributes to a text file. Name it saveData(Stringfilename) It should throw Exceptions.-Add a method to the tractor class to read all of atractors attributes from a text file. Name itloadData(String filename) It should throw Exceptions.-Test these methods by calling them from your testdrivers main() method public class Tractor { private String ID; private double rentalRate; private int rentalDays; Tractor() { this.ID = "00"; this.rentalDays = 0; this.rentalRate = 0; } } Tractor(String ID, double rentalRate, int rentalDays) { this.setID(ID); this.setRentalDays(rentalDays); this.setRentalRate(rentalRate); } /** * @return the iD */ public String getID() { return ID; } /** * @return the rentalDays */ public int getRentalDays() { return rentalDays; } /** * @return the rentalRate */…arrow_forwardWhat is the best way to implement a dynamic array if you don't know how many elements will be added? Create a new array with double the size any time an add operation would exceed the current array size. Create a new array with 1000 more elements any time an add operator would exceed the current size. Create an initial array with a size that uses all available memory Block (or throw an exception for) an add operation that would exceed the current maximum array size.arrow_forwardmsleep**** For this assignment, you must name your R file msleep.R (using Rstudio) For all questions you should load tidyverse. You should not need to use any other libraries. Load tidyverse with suppressPackageStartupMessages(library(tidyverse)) The actual data set is called msleep Round all float/dbl values to two decimal places. If your rounding does not work the way you expect, convert the tibble to a dataframe by using as.data.frame() All statistics should be run with variables in the order I state E.g., “Run a regression predicting mileage from mpg, make, and type” would be: lm(mileage ~ mpg + make + type...) Before attempting to answer these, or if you lose points upon an attempt, please review all CodeGrade information provided in the CodeGrade overview submodule - if you do not you are likely to lose points. What is the variance in total sleep for carni vores and those whose conservation status is lc? Your answer should be in a 1 X 1 data frame with a value…arrow_forward
- Evaluate the following functions: 1. prune [[[3],[1,2,4]],[[1,3],[3,4]]] 2. fix prune [[[5],[6,8]],[[7],[7,8]]] 3. blocked [[[3,4],[],[3]],[[1],[1,2],[1]]] 4. expand [[[1,2],[3]],[[4),[1,2]]arrow_forwardMilestone #1 - Ask Quiz Type In a file called main.py, implement a program that asks the user for a quiz type and prints the file name for that quiz type. The program should have one input prompt: the integer value of the personality quiz number. Your program should print the welcome message and the list of the personality quizzes, ask for the test number, and then print the file name. You are required to use the provided dictionary with the personality quizzes names. The list of the personality quizzes should be printed in alphabetical order. See example below. Welcome to the Personality Quiz! What type of Personality Quiz do you want to run? 1 BabyAnimals 2 Brooklyn99 3 Disney 4 Hogwarts 5 MyersBriggs 6 Sesame Street 7 - StarWars 8 - Vegetablesarrow_forward1-Write a JAVA program that reads an array from input file and invokes twodifferent methods Sort and Max ,that sorts the elements of the array and findsthe Max element and writes out the resulted array in to output file . Usetwo interfaces for methods and throw Exception Handling for Out Of Boundindex for the arrayarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
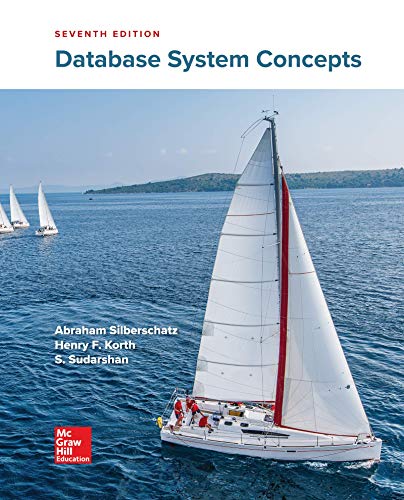
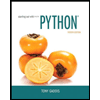
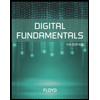
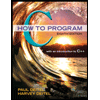
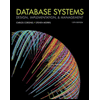
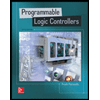