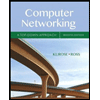
please see image for instructions
starter code for Main.java
import java.io.*;
import java.util.*;;
public class Main {
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.println(countConnectedComponents("data.txt"));
}
public static int countConnectedComponents(String fileName)
{
try
{
//the file to be opened for reading
FileInputStream fis=new FileInputStream(fileName);
Scanner sc=new Scanner(fis); //file to be scanned
//returns true if there is another line to read
ArrayList<int []> edge = new ArrayList<int[]>();
Set<String> set = new HashSet<String>();
while(sc.hasNextLine())
{
int temp [] = new int[2];
int index = 0;
for(String s : sc.nextLine().split(" "))
{
temp[index] = Integer.parseInt(s);
set.add(s);
index++;
}
edge.add(temp);
}
sc.close(); //closes the scanner
int n = set.size();
int[] root = new int[n];
// initialize each node is an island
for(int i=0; i<n; i++){
root[i]=i;
}
int count = n;
for(int i = 0; i < edge.size(); i++)
{
int x = edge.get(i)[0];
int y = edge.get(i)[1];
int xRoot = root[x - 1];
int yRoot = root[y - 1];
if(xRoot!=yRoot){
count--;
root[xRoot]=yRoot;
}
}
return count;
}
catch(IOException e)
{
e.printStackTrace();
}
return 0;
}
}
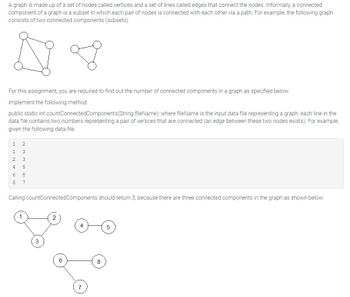

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Compliant/Noncompliant Solutions The following code segment was provided in Secure Coding Guidelines for Java SE: void readData() throws IOException{ BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("file"))); // Read from the file String data = br.readLine();} The code is presented as a noncompliant coding example. For this assignment, identify two compliant solutions that can be utilized to ensure the protection of sensitive data. Provide a detailed explanation of factors, which influence noncompliant code and specifically how your solutions are now compliant. Your explanation should be 2-3 pages in length. Submit the following components: Word document with appropriate program analysis for your compliant solutions and source code in the Word file. Submit your .java source code file(s). If more than 1 file, submit a zip file.arrow_forwardWrite a class FileConverter with a constructor that accepts a String file name as its argument. It should store filename as its attribute (with a accessor getFilename(). The class should have the following methods: • createUpperCaseFile(): Accepts a String parameter targetFilename. It will open up both the file specified in class attribute filename and the method parameter targetFilename. Then it will convert all lines of the class attribute filename to upper case and write it to target file, line by line. • createRowCharCountFile(): Accepts a String parameter targetFilename. It will open up both the file specified in class attribute filename and the method parameter targetFilename. Then it will count the number of chars in each line of the class attribute filename and write it to target file line by line. The class must come with a main() method, which will create an object instance and generate two target files based on the source file, one all upper case, and another row char count.arrow_forwardJava programming 1. Write the code to print out an individual customer’s information. Your code must read the customer from a file, populate the object, and display the data from the object. Customer customer = new Customer (); 2. Write the signature line for this method 3, Write the line of code to read a line from the file Assume the object has been populated with the proper values. Write the line of code that will print one of the properties in the customer object. Be sure to include a textual description, e.g. “Customer Name: “arrow_forward
- a java assignment about 4 used defined exception using array loop based on real life scenario. (use only java)arrow_forwardExplain the term relocation. Explain the structure of Typical ELF executable object file. What would be the output on the terminal when a user tries to compile and execute the following files. Write the steps to compile and run as well. #include <stdio.h> int buf[]={1,3}; int main() { swap(); printf("x= %d , y=%d\n", buf[0], buf[1]); return 0; } #include <stdio.h> extern int buf[]; int *bufp0 = &buf[0]; static int *bufp1; void swap() { int temp; bufp1 = &buf[1]; temp = *bufp0; *bufp0 = *bufp1; *bufp1 = temp; }arrow_forwardFilelO 01: Write a Message Write a FileI001 class with a method named writeMessage() to takes message as a String and a filename (as a String) (in that order). Have the method, write the message to the filename. Your code will either need to 1) catch all checked exceptions or 2) use the throws keyword. public class FileI001{ public void writeMessage( String message, String filename ){arrow_forward
- createDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forwardChallenge 3: Encryption.java and Decryption.java Write an Encryption class that encrypts the contents of a binary file. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded content out to a second file. The second file will be a version of the first file, but written in a secret code. Use a simple encryption technique, such as reading one character at a time, and add 10 to the character code of each character before it is written to the second file. Come up with your own encryption method. Also write a Decryption class that reads the file produced by the Encryption program. The decryption file will use the method that you used and restore the data to its original state, and write it to a new file.arrow_forwardC# also I have a error that states cannot open assembly 'debugone4.exe' no such file or directoryarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
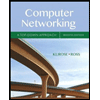
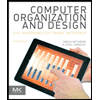
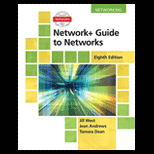
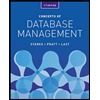
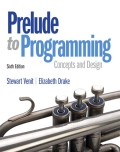
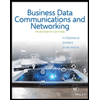