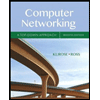
public int insert(int value);
/* Creates a node with the parameter as its value
* and inserts the node into the tree in the appropriate position.
* This method should call upon the recursive insert_r() helper method
* you write.
*
* we will make the assumption for this assignment (and implement it so)
* that the values in a BST are unique... no duplicates
*
* If we insert a value and it succeeded... adds it to the tree
* return the element that was added
*
* If we try to add an element and it is a duplicate, return the element
* but make no changes to the tree... no new nodes added
*
* @param int value to be inserted in tree
* @return integer that was insrted after insertion is done.
*
* Example 1: Suppose we have the tree below:
*
* (27)
* / \
* (4) (29)
* / \
* (1) (8)
* After calling insert(5), we'd obtain the following tree:
*
* (27)
* / \
* (4) (29)
* / \
* (1) (8)
* /
* (5)
*
* Example 2: Suppose we have the tree below:
*
* (87)
* / \
* (42) (128)
* \ \
* (46) (145)
* /
* (44)
*
* After calling insert(147), we'd obtain the following tree:
*
* (87)
* / \
* (42) (128)
* \ \
* (46) (145)
* / \
* (44) (147)
*
**/
DESCRIPTIONS AND CODE IMPLEMENTATION IN PICTURES
FUNCTION SHOULD INSERT A NODE WITH A VALUE INTO A BST INTO ITS DESIGNATED SPOT
DO THIS RECURSIVELY
PLS AND THANK YOU!!!!!!!!!!!!!
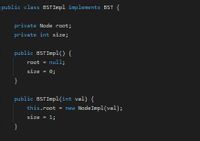
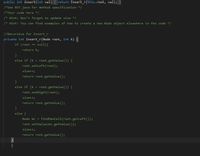

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write a method isBST() that takes a Node as argument and returns true if the argument node is the root of a binary search tree, false otherwise.Hint : This task is also more difficult than it might seem, because the order in which youcall the methods in the previous three exercises is important.Write a method isBST() that takes a Node as argument and returns true if the argument node is the root of a binary search tree, false otherwise.Hint : This task is also more difficult than it might seem, because the order in which youcall the methods in the previous three exercises is important.arrow_forwardIn python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardAdd more methods to the singly linked list class then test them• search(e) // Return one node with 3 values (stuID, stuName, stuScore) which matches agiven key e (studentID).• addAfter(e, stuID, stuName, stuScore) //Add a new node with 3 values (stuID,stuName, stuScore) after the node with the key e (studentID).• removeAt(e) //Remove a node which matches a given key e (studentID)• count() //Return a number of nodes of list.• update(stuID, stuName, stuScore) //Update the values of one node three codes below two in pictures one,typed out. public class SlinkedList<A,B,C> { private Node head; private Node tail; private int size; public SlinkedList(){ head=null; tail=null; size=0; } public int getSize(){ return size; } public boolean isEmpty(){ return size == 0; } public A getFirstStuId(){ if(isEmpty()) return null; return (A) head.getStuID();}public B getFirstStuName(){ if(isEmpty())…arrow_forward
- 24. Add the following new method in the BST class. /** Returns the number of nodes in this binary tree */ public int getNumberofNodes () Requirements: a. Don't use return size; b.write a recursive method that returns the number of nodes starting from www the root.arrow_forward8. Write down the insertBefore method which inserts a new element in the list before the node containing the given element. The method takes as parameters a dummy headed doubly linked circular list, the element existing in the list and new element to be added. public void insertBefore (Node head, Object elem, Object newElement) { //to do OR def insertBefore (head, elem, newElement): pass insertBefore (head, 3, 50) Sample Input Sample Output Ox21 2 223 240 O x21 2 22 50 ² 3 2 4 0arrow_forwardAdd a method in the BST class to return the number of thenonleaves as follows:/** Return the number of nonleaf nodes */public int getNumberofNonLeaves()arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
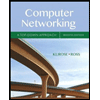
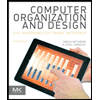
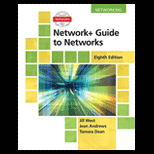
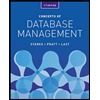
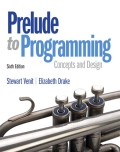
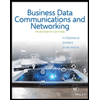