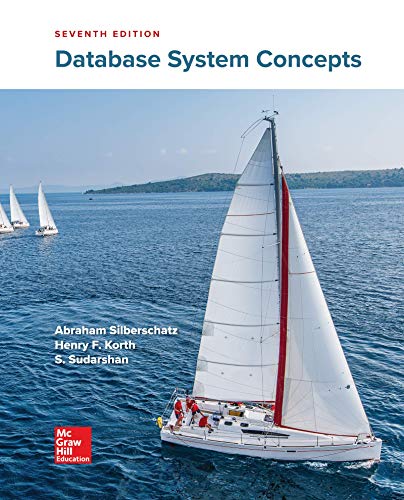
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Java Code: How to implement logic for ParseIf ParseFor, ParseWhile, ParseDoWhile, ParseDelete, ParseReturn, and ParseFunctionCall where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these methods.
Expert Solution

arrow_forward
Step 1: Some Overviews
To parse a node data structure in Java using the ParseIf and ParseSelf methods, you must design the data structure, create the parsing logic, and handle errors with sensible error messages.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using java code Problem: Suppose we want to write a program for the class BinaryTree that counts the number of times an object occurs in the tree. We need to use a method with the following header, public int count (O anObject) 1. Name your class objectCounter 2. Define main method, test the program 3. Write a method using one of the iterators of the binary tree. 4. Write another method using a private recursive method of the same name NB: Can you add main method to test the code please for 2. Define main method, test the programarrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardSuppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.Code: #include <iostream>#include <queue>#include <string>#include <utility> using namespace std; class Stack{public: Stack(); string top(); void pop(); void push(string element);private: int count; priority_queue<pair<int, string>> pqueue;}; Stack::Stack(){ /* Your code goes here */} string Stack::top(){ /* Your code goes here */} void Stack::pop(){ /* Your code goes here */} void Stack::push(string element){ /* Your code goes here */} int main(){ Stack…arrow_forward
- Java Code: How to implement logic for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.arrow_forward!!! JAVA ONLY!! Create a class Tree to model a binary tree. This class should contain a single Node which is theroot node of the tree, and should have appropriate getters/setters and toString().Again, test out your class by creating a tree and nodes objects; and by calling various methodson the them in the main method.Create the following tree using instances of your classes: HoareJonesFitzgerald AstarteRoscoeBroadfoot CreeseThis tree captures PhD supervision hierarchy: Jones was supervised by Hoare, etc.Write a method traceAndPrint for your Tree class. It should take as a parameter a stringof L and R characters, and, starting at the root, trace a path through the tree, taking a leftchild when encountering L and a right child when encountering R. Each node passed, startingwith the root, should be printed to the console. If directions given are wrong, i.e. a null isencountered, or any other character is passed to the method, an exception should be thrown.The exception should indicate…arrow_forwardJava Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forward
- Implementation of largest-cover difference #23@title Implementation of largest-cover difference def rectangle_difference(r, t): "Computes the rectangle difference rt, and outputs the result as a list of rectangles." assert len(r) == len(t), "Rectangles have different dimensions" ### YOUR SOLUTION HERE In the above code, we notice how it paid off to encapsulate intervals in their own abstraction. Now that we need to reason about rectangles, we do not need to get bogged down into complicated considerations of how to perform difference; the comparisons between endpoints are all done in the context of intervals, where it is easier to reason about them. It is often the case that problems become easy, once you think at them in the appropriate context. It is much easier to develop the code for the difference of intervals, as we have done, and then move on to difference of rectangles, rather than trying to write code for rectangle difference directly. The result is also far more elegant. # Let…arrow_forwardIt's a very nice application of stacks. Consider that a freight train has n railroad cars. Each to be left at different station. They're numbered 1 through n & freight train visits these stations in order n through 1. Obviously, the railroad cars are labeled by their destination. To facilitate removal of the cars from the train, we must rearrange them in ascending order of their number (i.e. 1 through n). When cars are in this order, they can be detached at each station. We rearrange cars at a shunting yard that has input track, output track & k holding tracks between input & output tracks (i.e. holding track). The figure shows a shunting yard with three holding tracks H1, H2 & H3, also n = 9. The n cars of freight train begin in the input track & are to end up in the output track in order 1 through n from right to left. The cars initially are in the order 5,8,1,7,4,2,9,6,3 from back to front. Later cars are rearranged in desired order. To rearrange cars, we…arrow_forwardPlease answer the question in the screenshot. The language used here is Java. Please use the starting code.arrow_forward
- I ran the code and got an error. Please fix the error and provide me the correct code for all parts. Make sure to give the screenshot of the output as well.arrow_forwardIn python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardIm trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } }…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
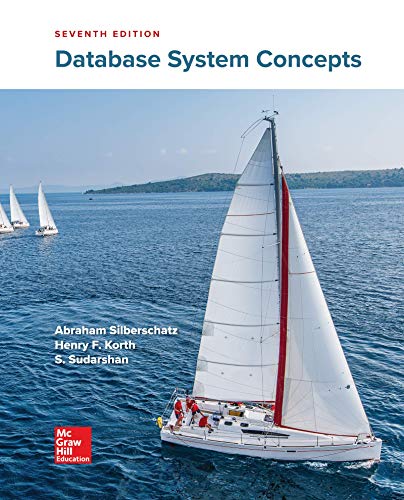
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
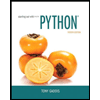
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
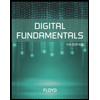
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
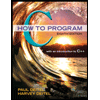
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
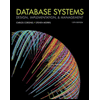
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
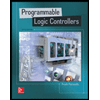
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education