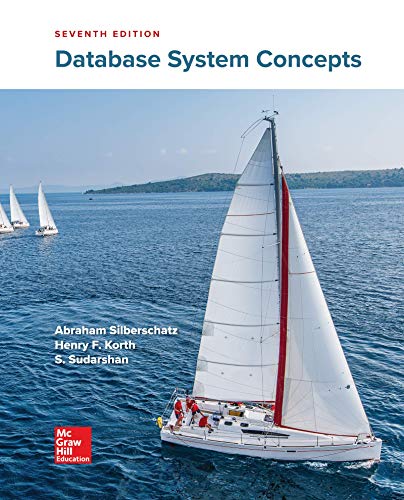
Java Code: How to implement logic for ParseDoWhile, ParseDelete, and ParseReturn where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these three methods.

1. Start
2. Define the 'Token' class to represent lexical tokens with 'type' and 'lexeme' attributes.
3. Define an enumeration 'TokenType' to represent different token types.
4. Define classes for different AST nodes, including 'Node', 'StatementNode', 'DoWhileNode', 'DeleteNode', 'ReturnNode', and 'IdentifierNode'.
5. Create a 'Parser' class to handle parsing logic for the input tokens.
6. In the 'Parser' class:
a. Initialize the parser with a list of tokens.
b. Implement methods to parse 'do-while' statements, 'delete' statements, and 'return' statements, including error handling.
c. Create simplified methods for parsing expressions and blocks (not shown in detail).
d. Implement 'check', 'match', 'advance', and 'isAtEnd' helper methods to manage token consumption.
7. In the 'Main' class:
a. Define a list of sample tokens representing input code.
b. Initialize a 'Parser' with the sample tokens.
c. Parse 'do-while', 'delete', and 'return' statements using the parser, handling potential parse errors.
d. You can further process and execute the parsed statements as needed.
8. End.
Step by stepSolved in 4 steps with 7 images

- Suppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.Code: #include <iostream>#include <queue>#include <string>#include <utility> using namespace std; class Stack{public: Stack(); string top(); void pop(); void push(string element);private: int count; priority_queue<pair<int, string>> pqueue;}; Stack::Stack(){ /* Your code goes here */} string Stack::top(){ /* Your code goes here */} void Stack::pop(){ /* Your code goes here */} void Stack::push(string element){ /* Your code goes here */} int main(){ Stack…arrow_forwardJava Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardIn this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forward
- in Javaarrow_forwardAssume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forwardIm trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } }…arrow_forward
- In Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forwardJava Programming: Make AST Nodes: IfNode, WhileNode, RepeatNode. All will have BooleanCompare for a condition and a collection of StatementNode. ForNode will have a Node for from and a node for to. This will have to be of type Node because it could be any expression. For example: for a from 1+1 to c-6 Make parsing functions for each. Java won't let you create methods called if(), etc. parseIf() is an example of a way around that; use whatever you like but use good sense in your names. Next let's look at function calls. Each function has a name and a collection of parameters. A parameter can be a VAR variable or something that came from booleanCompare (IntegerNode, VariableReferenceNode, etc). It would be reasonable to make 2 objects - ParameterVariableNode and ParameterExpressionNode. But for this very simple and well-defined case, we can do something simple: ParameterNode has a VariableReferenceNode (for VAR IDENTIFIER) and a Node for the case where the parameter is not a VAR.…arrow_forwardWrite all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas…arrow_forward
- Java Code: How to implement logic for ParseBlock and ParseContinue/Break where all of the Node data structure correct, parses correctly, throws exceptions with good error messages. Make sure to write block of codes for these two methods.arrow_forwardPlease use the information in the second screenshot about the BST class and Node class to create a class BSTApp. Please make sure to use the following code as a starting point. import java.util.*; public class BSTTest{public static void main(String[] args) {// perform at least one test on each operation of your BST } private int[] randomArray(int size) {// remove the two linesint[] arr = new int[1];return arr;} // the parameters and return are up to you to define; this method needs to be uncommented// private test() {//// }} Base of class Node public class Node {int key;Node left, right, parent; public Node() {} public Node(int num) {key = num;left = null;right = null;parent = null;}} Base of class BST import java.util.*; class BST {// do not change thisprivate Node root;private ArrayList<Integer> data; // DO NOT MODIFY THIS METHODpublic BST() {root = null;data = new ArrayList<Integer>(0);} // DO NOT MODIFY THIS METHODpublic ArrayList<Integer> getData() {return…arrow_forwardAssume we have an IntBST class, which implements a binary search tree of integers. The field of the class is a Node variable called root that refers to the root element of the tree. 1) Write a recursive method for this class that computes and returns the sum of all integers less than the root element. Assume the tree is not empty and there is at least one element less than the root. 2) Write a recursive method that prints all of the leaves, and only the leaves, of a binary search tree. 3) Write a method, using recursion or a loop, that returns the smallest element in the tree.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
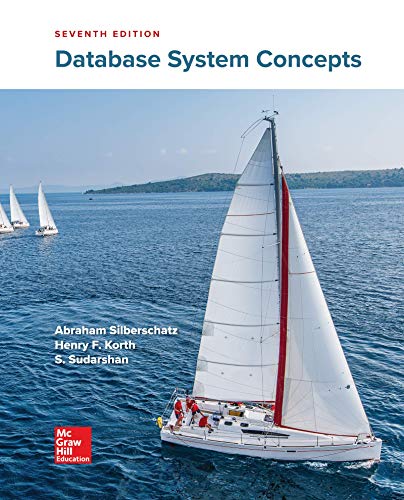
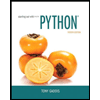
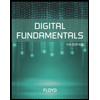
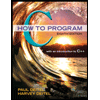
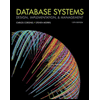
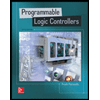