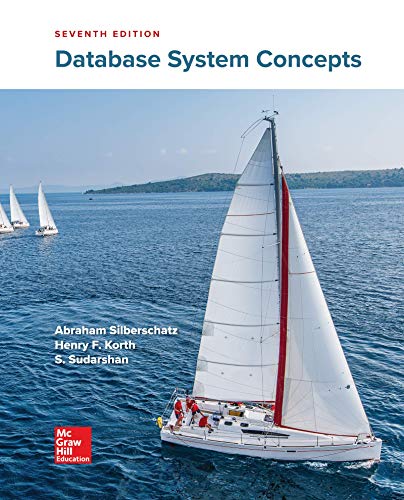
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![/**
removeDuplicates returns a new array containing the unique values in the
* array. There should not be any extra space in the array --- there should
* be exactly one space for each unique element (Hint: numUnique tells you
* how big the array should be). You may assume that the list is sorted, as
* you did for numUnique.
*
*
* Your solution may call numUnique, but should not call any other
* functions. After the call to numUnique, you must go through the array
* exactly one more time. Here are some examples (using "==" informally):
*
*
*
*
*
*
*
*
*
<pre>
}
new double[] { }
== removeDuplicates (new double[] { })
new double[] {11}
removeDuplicates (new double[] {11})
== removeDuplicates (new double[] { 11, 11, 11, 11 })
new double[] { 11, 22, 33, 44, 55, 66, 77, 88 }
== removeDuplicates (new double[] { 11, 11, 11, 11, 22, 33, 44, 44, 44, 44, 44, 55, 55, 66, 77, 88, 88 })
removeDuplicates (new double[] { 11, 22, 33, 44, 44, 44, 44, 44, 55, 55, 66, 77, 88 })
==
==
* </pre>
*/
public static double [] removeDuplicates (double[] list) {
double [] result = new double[numUnique (list)];
// ..
return result; // TODO: fix this](https://content.bartleby.com/qna-images/question/7d2da000-1b5a-4e3b-a44e-4b528fa64829/c5c80fa8-b4c1-4449-9b30-ec7ffb316dc2/093wb8h_thumbnail.png)
Transcribed Image Text:/**
removeDuplicates returns a new array containing the unique values in the
* array. There should not be any extra space in the array --- there should
* be exactly one space for each unique element (Hint: numUnique tells you
* how big the array should be). You may assume that the list is sorted, as
* you did for numUnique.
*
*
* Your solution may call numUnique, but should not call any other
* functions. After the call to numUnique, you must go through the array
* exactly one more time. Here are some examples (using "==" informally):
*
*
*
*
*
*
*
*
*
<pre>
}
new double[] { }
== removeDuplicates (new double[] { })
new double[] {11}
removeDuplicates (new double[] {11})
== removeDuplicates (new double[] { 11, 11, 11, 11 })
new double[] { 11, 22, 33, 44, 55, 66, 77, 88 }
== removeDuplicates (new double[] { 11, 11, 11, 11, 22, 33, 44, 44, 44, 44, 44, 55, 55, 66, 77, 88, 88 })
removeDuplicates (new double[] { 11, 22, 33, 44, 44, 44, 44, 44, 55, 55, 66, 77, 88 })
==
==
* </pre>
*/
public static double [] removeDuplicates (double[] list) {
double [] result = new double[numUnique (list)];
// ..
return result; // TODO: fix this
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- ز٢arrow_forwardExercise - Collection Functions Using the code below, use the map function to create an array of Int values, whose values are equal to the original integer value, plus 1. Use $0 as you iterate through the values of the array. Print the resulting collection. 5 let testScores = [65, 80, 88, 90, 47] Using the code below, use the filter function to create a new array of String values. The new array should only include Strings longer than four characters. Use $0 as you iterate through the values of the array. Print the resulting collection. Olet schoolSubjects = ["Math" , "Computer Science", "Gym", "English", "Biology"] Using the code below, use the reduce function to subtract all of the values within the array from the starting value 100. Print the resulting value. let damageTaken = [25, 10, 15, 30, 20]arrow_forwardStep 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function. 0 Pay attention to the print() function. How many elements is it printing? main.cpp -- client code to test your staticarray class. Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to…arrow_forward
- C++ Write a function named “checkInvalidHours” that accepts an array of PaySubobjects and its size. It will go through the array and check for invalid hours(negative values). If it is negative, it will reset the hourly payrate to 0. It willreturn how many PayStub objects that it has reset the payrate to 0.Please show you would call and test this function.arrow_forwardLinks Bing Remaining Time: 1 hour, 24 minutes, 17 seconds. * Question Completion Status: Р QUESTION 8 bbhosted.cuny.edu/webapps/assessment/take/launch.jsp?course_assessment_id=_2271631_1&course_id=_2 Implement the following: 1) Define a function evenList() with an arbitrary parameter a. P 2) This function accepts any number of integer arguments. 3) evenList() stores all even numbers into a list and returns the list. 4) Call the function with 5, 6, 7, 8, 9, 10 as arguments. 5) Print the result of the function call. Make sure to precisely match the output format below. Write your code in the ANSWER area provided below (must include comments if using code is not covered in the course). Example Output [6, 8, 10] For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). BIUS Paragraph Arial QUESTION 9 Programs s 10pt Click Save and Submit to save and submit. Click Save All Answers to save all answers. く Ev Avarrow_forwardIn the following code both myList and yourList are dynamic arrays of the same size. The code initializes the array myList to certain values. The value in each element of yourList should be twice the value in the corresponding element in myList. However, the output of this code would show that is not the case. Provide the correct code to accomplish the desired results. int *myList;int *yourList;myList = new int[5];myList[0] = 8;for (int i = 1; i < 5; i++)myList[i] = i * myList[i - 1];yourList = myList;for (int i = 0; i < 5; i++)yourList[i] = 2 * myList[i];arrow_forward
- Write a findSpelling Function -PHP Write a function findSpellings($word, $allWords) that takes a string and an array of dictionary words as parameters. The function should return an array of possible spellings for a misspelled $word. One way to approach this is to use the soundex() Words that have the same soundex are spelled similarly. Return an array of words from $allWords that match the soundex for $word. I have most of the code. How do I append the $sound to the $mathcing array?arrow_forward6. the grade is under 20 which is outlier, remove it from the array list. 7. Print array list using System.out.println() 8. Use indexOf to print index of 80. 9. Use get function. 10. What is the difference between get and index of? 11. Print the values of the array list using Iterator class. 12.. Delete all the values of the array list using clear function. 13. Print all the values of the array after you execute clear using System.out.println(). what is the result of using clear function? 14. What is the shortcoming of using array List?arrow_forward8arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
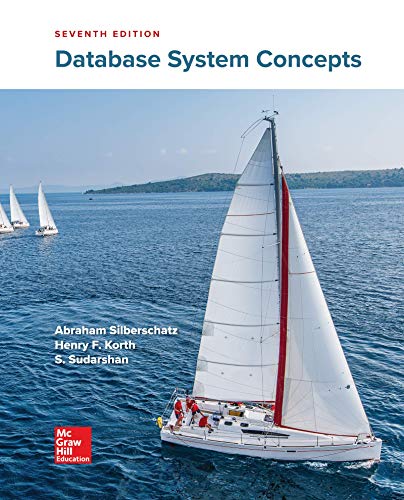
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
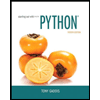
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
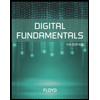
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
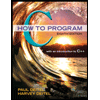
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
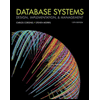
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
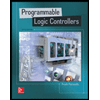
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education