s of printf statements to display the algorithm name and the output header d. Declare a one-dimensional array, data type integer, to store the page requests (i.e., pageRequests) initialized to data set 4, 1, 2, 4, 2, 5, 1, 3, 6 e. Declare a variable, data type integer, to store the page faults, initialize to 0 (i.e., pageFaults) f. Declare a one-dimensional array, data type integer, to store the memory frame a page is allocated to (i.e., allocation), size is parameter FRAMES g. Declare a variable, data type integer, to st
s of printf statements to display the algorithm name and the output header d. Declare a one-dimensional array, data type integer, to store the page requests (i.e., pageRequests) initialized to data set 4, 1, 2, 4, 2, 5, 1, 3, 6 e. Declare a variable, data type integer, to store the page faults, initialize to 0 (i.e., pageFaults) f. Declare a one-dimensional array, data type integer, to store the memory frame a page is allocated to (i.e., allocation), size is parameter FRAMES g. Declare a variable, data type integer, to st
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 1TF: Mark the following statements as true or false. A double type is an example of a simple data type....
Related questions
Question
Write function LRU using C to do the following.
a. Return type void
b. Empty parameter list
c. Write a series of printf statements to display the algorithm
name and the output header
d. Declare a one-dimensional array, data type integer, to store the
page requests (i.e., pageRequests) initialized to data set 4, 1,
2, 4, 2, 5, 1, 3, 6
e. Declare a variable, data type integer, to store the page faults,
initialize to 0 (i.e., pageFaults)
f. Declare a one-dimensional array, data type integer, to store the
memory frame a page is allocated to (i.e., allocation), size is
parameter FRAMES
g. Declare a variable, data type integer, to store number of pages
(i.e., pages) initialized to the number of page requests
h. Declare a variable, data type integer, to store page hits (i.e.,
counter)
i. Declare a one-dimensional array, data type integer, to store
when a page is allocated to (i.e., time), size is parameter 10
j. Declare a variable, data type integer, to track page hits/misses
(i.e., flag1)
k. Declare a variable, data type integer, to track page hits/misses
(i.e., flag2)
l. Declare a variable, data type integer, to store the position of
the most recent request, initialize to 0 (i.e., position)
m. Initialize the allocation array by calling function memset,
passing arguments
i. Array allocation
ii. -1 (i.e., INVALID)
iii. sizeof(allocation)
n. Using a looping construct, iterate through the number of pages
i. Set variable flag1 equal to 0
ii. Set variable flag2 equal to 0
iii. Using a looping construct, iterate through the number
of FRAMES
1. If the current page request is equal to the
current frame allocation
a. Increment the counter variable by 1
b. Update array time, index of the loop
control variable, set it equal to variable
counter
c. Set variable flag1 equal to 1
d. Set variable flag2 equal to 1
e. Break out of the loop
iv. If variable flag1 is equal to 0
1. Using a looping construct, iterate through the
number of FRAMES
a. If the current element of array
allocation is equal to a -1 (i.e.,
INVALID)
i. Increment the counter variable
by 1
ii. Increment the pageFaults
variable by 1
initialize to 0 (i.e., pageFaults)
f. Declare a one-dimensional array, data type integer, to store the
memory frame a page is allocated to (i.e., allocation), size is
parameter FRAMES
g. Declare a variable, data type integer, to store number of pages
(i.e., pages) initialized to the number of page requests
h. Declare a variable, data type integer, to store page hits (i.e.,
counter)
i. Declare a one-dimensional array, data type integer, to store
when a page is allocated to (i.e., time), size is parameter 10
j. Declare a variable, data type integer, to track page hits/misses
(i.e., flag1)
k. Declare a variable, data type integer, to track page hits/misses
(i.e., flag2)
l. Declare a variable, data type integer, to store the position of
the most recent request, initialize to 0 (i.e., position)
m. Initialize the allocation array by calling function memset,
passing arguments
i. Array allocation
ii. -1 (i.e., INVALID)
iii. sizeof(allocation)
n. Using a looping construct, iterate through the number of pages
i. Set variable flag1 equal to 0
ii. Set variable flag2 equal to 0
iii. Using a looping construct, iterate through the number
of FRAMES
1. If the current page request is equal to the
current frame allocation
a. Increment the counter variable by 1
b. Update array time, index of the loop
control variable, set it equal to variable
counter
c. Set variable flag1 equal to 1
d. Set variable flag2 equal to 1
e. Break out of the loop
iv. If variable flag1 is equal to 0
1. Using a looping construct, iterate through the
number of FRAMES
a. If the current element of array
allocation is equal to a -1 (i.e.,
INVALID)
i. Increment the counter variable
by 1
ii. Increment the pageFaults
variable by 1
iii. Update the allocation array for
the current frame by setting it
equal to the current
pageRequest
iv. Update array time for the
current frame by setting it equal
to variable counter
v. Set variable flag2 equal to 1
vi. Break out of the loop
v. If variable flag2 is equal to 0
1. Set variable position equal to function call
findLRU passing array time as an argument
2. Increment the counter variable by 1
3. Increment the pageFaults variable by 1
4. Update the allocation array at index position
by setting it equal to the current pageRequest
5. Update array time at index position by setting
it equal to variable counter
vi. Call function displayPages passing arguments
1. the current pageRequest
2. allocation array
o. Display to the console the total number of page faults
the current frame by setting it
equal to the current
pageRequest
iv. Update array time for the
current frame by setting it equal
to variable counter
v. Set variable flag2 equal to 1
vi. Break out of the loop
v. If variable flag2 is equal to 0
1. Set variable position equal to function call
findLRU passing array time as an argument
2. Increment the counter variable by 1
3. Increment the pageFaults variable by 1
4. Update the allocation array at index position
by setting it equal to the current pageRequest
5. Update array time at index position by setting
it equal to variable counter
vi. Call function displayPages passing arguments
1. the current pageRequest
2. allocation array
o. Display to the console the total number of page faults
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
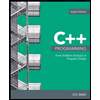
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
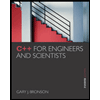
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
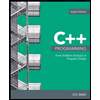
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
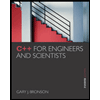
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr