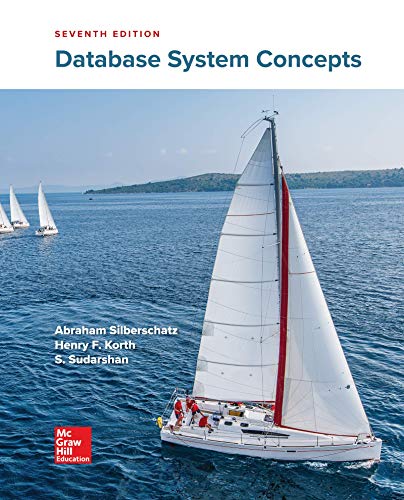
TASK 2
Time Complexity: Build a table and record the time complexity taken by linear and binary search to look for an element with the following array sizes: 10, 50, 100, 200, 500, 700, 1000. Compare the performance of both algorithms highlighting the time complexity for both algorithms when run on small input size and when run on large input size.
To accomplish this, load a list with the file data and then create a program that selects at random X amount of elements from the original list (where X is a value that the user selects) and place them in an array, then select at random an element in the original list to search in the sampled array using both algorithms. Measure the time it takes for both algorithms on an array of a given input size.
For this part just create a class called Task2Exercise in your project, reuse any code you did for the first part.
TASK 3
Using one of the sorting methods, sort the Car ArrayList based on their ‘City mpg’ feature. Justify your choice of the
HINTS:
A report on the second exercise showing a graph of running time vs. input size of both algorithms and your conclusions.

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 2 images

- The script has four steps: Read a list of integers (no duplicates). Output the numbers in the list. Perform an insertion sort on the list. Output the number of comparisons and swaps performed during the insertion sort. Steps 1 and 2 are provided in the script. Implement step 3 based on the insertion sort algorithm in the book. Modify insertion_sort() to: Count the number of comparisons performed. Count the number of swaps performed. Output the list during each iteration of the outside loop. Implement step 4 at the end of the script. Hints: In order to count comparisons and swaps, modify the while loop in insertion_sort(). Use global variables for comparisons and swaps. The script includes three helper functions: read_nums() # Read and return a list of integers. print_nums(nums) # Output the numbers in nums swap(nums, n, m) # Exchange nums[n] and nums[m] Ex: When the input is: 3 2 1 5 9 8 the output is: 3 2 1 5 9 8 2 3 1 5 9 8 1 2 3 5 9 8 1 2 3 5 9 8 1 2 3 5 9 8 1 2 3 5 8 9…arrow_forwardQuestion in image Please explain how to do with the answer. Thank you.arrow_forwardWrite a recursive function to recursively print the file list returned by os.listdir().arrow_forward
- 3 2 5 6 4 1 In each blank, enter the list after the given iteration. Put one space between each pair of numbers. Do not put commas or any other character in your response besides the numbers and spaces. Do not put a space at the beginning or end of your response. What will the list be after the first iteration of selection sort? incorrect What will the list be after the second iteration of selection sort? incorrect What will the list be after the third iteration of selection sort? incorrect What will the list be after the fourth iteration of selection sort?arrow_forwardPut the following code in a main.py file and a module.py file. Define the names sort, list_max and sum_of_list after putting the code into a main file and a module file #predefined modules import random import math #function to sort the list in ascending order def sort(x): #predefined function sort() x.sort() #print the sorted list print("\nSorted list is: ",str(x)) #function to find the sum of list elements def sum_of_list(x): #predefined function sum() Sum=sum(x) #return the sum of list elements return Sum #function to list the maximum from the list def list_max(x): #predefined function max() maximum=max(x) #return maximum return maximum #function to test the above three function def main(): #set a flag variable flag=True #create a list list1=list() #initialize the list element by using randrange() predefined function of random module list1=[random.randrange(1, 50, 1) for i in range(0,7)] #print the…arrow_forwardComputer Science Using Java, write a simple Insertion Sort program that can read in integers from a text file (line by line) and sort them into another text file. Use inFile and outFile for the input and output files, respectively. Also make sure that the algorithm keeps track of the comparisons and exchanges performed by the sort so that they may be printed out in the console after the sort is completedarrow_forward
- For the list shown below, demonstrate the following sort: 10, 1, 5, 2, 6, 8, 4, 10, 6, 6, 2, 4, 1, 8, 7, 3 External sort. Use a run size of 4. Note: continue until the final list is sorted!arrow_forwardPYTHON QUESTION This assignment requires you to create a dictionary by reading the text file created for the Chapter 6 assignment. The dictionary should have player names for the keys. The value for each key must be a two-element list holding the player's goals and assists, respectively. See page 472! Start with an empty dictionary. Then, use a loop to cycle through the text file and add key-value pairs to the dictionary. Close the text file and process the dictionary to print the stats and determine the top scorer as before. In fact, much of the code used in program6_2.py can be copied and used for this program. Printing the stats for each player is the most challenging part of this program. To master this, refer to the examples in the zip file that can be downloaded from the "Dictionary values can be lists" link in the "Learn Here" part of this module. NOTE: you do not need to submit the text file. Submit just this program. The required output should be the same well-formatted table…arrow_forwardIn Java, Apply replacement selection sort to the following list assuming array size M = 3; 27 47 35 7 67 21 32 18 24 20 12 8 Show the content of each sorted file.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
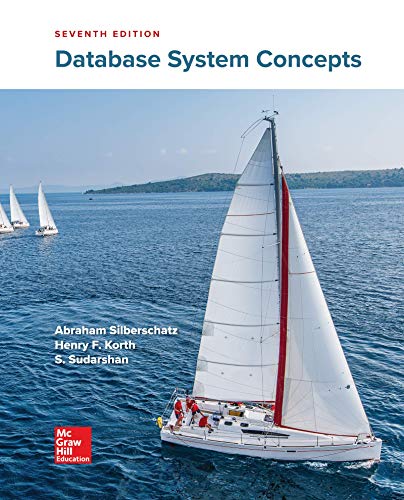
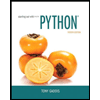
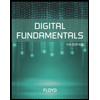
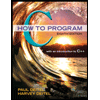
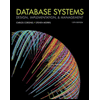
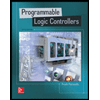