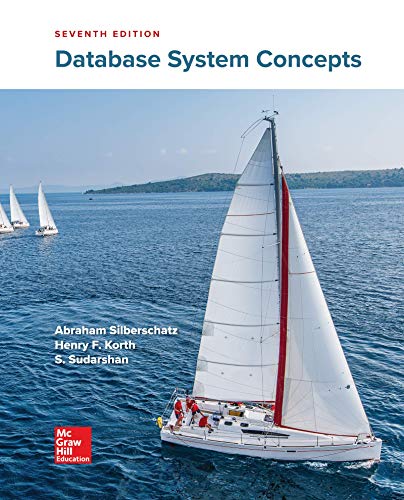
OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below:
-Push integers, strings, and names on the stack
-Push booleans
-Pushing an error literal or unit literal will push :error: or :unit:
onto the stack, respectively
-Command pop removes the top value from the stack
-The command add refers to integer addition. Since this is a binary operator, it consumes the top
two values in the stack, calculates the sum and pushes the result back to the stack
- Command sub refers to integer subtraction
-Command mul refers to integer multiplication
-Command div refers to integer division
-Command rem refers to the remainder of integer division
-Command neg is to calculate the negation of an integer
-Command swap interchanges the top two elements in the stack, meaning that the first element
becomes the second and the second becomes the first
-Command toString removes the top element of the stack and converts it to a string
-println command pops a string off the top of the stack and writes it, followed by a newline,
to the output file that is specified as the second argument to the interpreter function
-Command quit causes the interpreter to stop
Below are the test cases:
input1.txt:
push 1
toString
println
quit
input2.txt:
push 5
push :true:
push :false:
push "str1"
push str1
push :error:
push :unit:
toString
println
toString
println
toString
println
toString
println
toString
println
toString
println
toString
println
quit
input3.txt:
push 10
push 2
push 8
pop
add
toString
println
quit
input4.txt:
push 6
push 2
push 3
sub
add
toString
println
quit
input5.txt:
push :true:
push 7
push 8
push :false:
pop
mul
toString
println
toString
println
quit
output1.txt:
1
output2.txt:
:unit:
:error:
str1
str1
:false:
:true:
5
output3.txt:
12
output4.txt:
5
output5.txt:
56
:true:
![File "interpreter.ml", line 1:
Error: The implementation interpreter.ml
does not match the interface interpreter.cmi:
Values do not match:
val interpreter : instruction list -> unit
is not included in
val interpreter : string * string -> unit
File "interpreter.ml", line 23, characters 4-15: Actual declaration
Makefile:3: recipe for target 'all' failed
make:
[all] Error 2
***](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/9178def9-e1c7-47ef-a44f-5c9f07f4284e/rer5m2_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- *in java* A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Assume that the list will always contain less than 20 word pairs. Ex: If the input is: 3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank the output is: 867-5309 Your program must define and call the following method. The return value of getPhoneNumber() is the phone number associated with the specific contact name.public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int arraySize) Hint: Use two arrays: One for the string names, and the other for the string phone numbers.…arrow_forwardUse C++ language Can you please give me a code that runs properly this time Given a 2D board containing 'X' and 'O', capture all regions surrounded by 'X'.A region is captured by flipping all 'O's into 'X's in that surrounded region.For example, for the following board,X X X XX O O XX X O XX O X XAfter capturing all regions, the board becomesX X X XX X X XX X X XX O X XThe algorithm should take an input file, named “input.txt”, and output the resulting board in the console.Sample input.txt6X X X X X OX O X O O XX X O O O XX X X O X XX O X X O OX X X X X XThe first line is a number indicating the size of the board; in the above example, 6 means the board is .Output for in console:X X X X X OX X X X X XX X X X X XX X X X X XX X X X O OX X X X X Xarrow_forwardJava Program ASAP Please modify Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.HashMap;import java.util.Map;import java.util.Scanner;public class MorseCodeConverter { public static void main(String[] args) { Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text =…arrow_forward
- Complete the function that computes the length of a path that starts with the given Point. The Point structure = point on the plane that is a part of a path. The structure includes a pointer to the next point on the path, or nullptr if it's the last point. I'm not sure how to fill in the '?'; especially the while loop I'm not sure how to implement the condition for that.arrow_forwardImplement the"paint fill"function that one might see on many image editing programs. That is, given a screen (represented by a two-dimensional array of colors), a point, and a new color, fill in the surrounding area until the color changes from the original color.arrow_forwardTHIS NEEDS TO BE DONE IN C#!! The Tourtise and The Hair In this lab, we will be simulating the classic race of the tourtise and the hare. The race will take place on two paths of 70 tiles, which spans from left to right. The path will have two lanes, one for each animal. The path will be represented as an array of 70 characters, where each character will be the following: · A dash(i.e, “-“), which represents an empty tile. · An “h”, which represents the hare on the hare lane. · A “t”, which represents the tourtise on the tortise lane. All tiles will be “empty” (i.e. set to the dash) with the exception of the tiles that are occupied by the animals. As the animals move, the previous tile the animal was on will be set to empty, and the new tile will be changed to either “h” or “t”. Tile 70 will be the finishing tile, and the first animal to that tile will be declared the winner of the race. We will use randomization to determine how far each animal will move. Since this race will be…arrow_forward
- convert this code to JAVA location = [] size = [] rover = 0 def displayInitialList(location, size): global rover print("FSB# location Size") for i in range(len(location)): print(i," ",location[i]," ",size[i]) if rover<len(size)-1: print("Rover is at ",location[rover+1]) else: print("Rover is at ",location[rover]) def allocateMemory(location,size,blockSize): global rover if rover<len(size): while size[rover]<blockSize: rover+=1 if i==len(size): return False location[rover] += blockSize size[rover] -= blockSize rover+=1 return True else: return False def deallocateMemory(location,size,delLocation,delSize): i=0 while delLocation>location[i]: i+=1 location[i]-=delSize size[i]+=delSize while True: print("1. Define Initital memory\n2. Display initial FSB list\n3. Allocate memory\n4. Deallocate memory\n5. Exit") print("Enter choice: ",end="") choice = int(input()) if…arrow_forwardWrite code in assembly language Question 1: Construct a program using 2D array. Define a list of strings in the 2D arrays, the list should be only a string. Get a string from a user as input Search the user's string in the list of strings. If string is found print the string, and a message "String is Found". If string is not found print only a message "String is Not Found".arrow_forwardI need to write a Java ArrayList program called Search.java that counts the names of people that were interviewed from a standard input stream. The program reads people's names from the standard input stream and from time to time prints a sorted portion of the list of names. All the names in the input are given in the order in which they were found and interviewed. You can imagine a unique timestamp associated with each interview. The names are not necessarily unique (many of the people have the same name). Interspersed in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of names to be printed to the standard output stream. The list depends only on the names up to that point in the input stream and does include any people's names that appear in the input stream after the query. The list does not contain all the names of the previous interviews, but only a selection of them. A query provides the names of two people,…arrow_forward
- Write code in assembly language Question 1: Construct a program using 2D array. Define a list of strings in the 2D arrays, the list should be only a string. Get a string from a user as input Search the user's string in the list of strings. If string is found print the string, and a message "String is Found". If string is not found print only a message "String is Not Found".arrow_forwardpreLabC.java 1 import java.util.Random; 2 import java.util.StringJoiner; 3 4- public class preLabC { 5 6- 7 8 9 10 11 12 13 14 15 16 17 18 19 20 } public static String myMethod(MathVector inputVector) { String vectorStringValue = new String(); // empty String object System.out.println("here is the contents object, inputVector: + inputVector); // get a String out of that MathVector object. Do it here. On the next line. // return the double value here. return vectorStringValue; "1 ▸ Compilation Description A MathVector object will be passed to your method. Return its contents as a String. If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector object as a String. This makes it useful for displaying to the user. /** * Returns a String representation of this vector. The String should be in the format "[1, 2, 3]" * * @return a String representation of this vector * @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method. */…arrow_forwardLanguage Pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
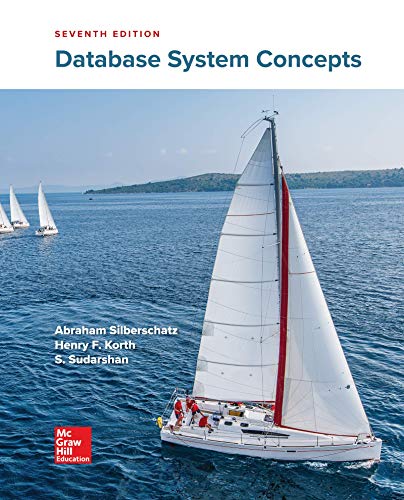
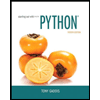
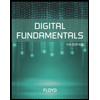
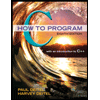
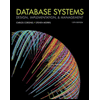
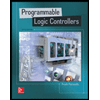