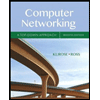
Try pthreads.cpp. Modify it so that they run 3 threads (instead of two) and each thread runs a different function, displaying a different message. Copy-and-paste the source code and the outputs in your report.
/*
pthreads_demo.cpp
A very simple example demonstrating the usage of pthreads.
Compile: g++ -o pthreads_demo pthreads_demo.cpp -lpthread
Execute: ./pthreads_demo
*/
#include <pthread.h>
#include <stdio.h>
using namespace std;
//The thread
void * thread_func (void *data)
{
char *tname = (char *) data;
printf("My thread identifier is %s\n", tname);
pthread_exit (0);
}
int main ()
{
pthread_t id1, id2; //thread identifiers
pthread_attr_t attr1, attr2; //set of thread attributes
char *tnames[2] = { "Thread 1", "Thread 2" }; //names of threads
//get the default attributes
pthread_attr_init (&attr1);
pthread_attr_init (&attr2);
//create the threads
pthread_create (&id1, &attr1, thread_func, tnames[0]);
pthread_create (&id2, &attr2, thread_func, tnames[1]);
//wait for the threads to exit
pthread_join (id1, NULL);
pthread_join (id2, NULL);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Consider the following data that are shared between two threads and are initialised before the threads are executed, as follows: boolean flag = true; int x = 50; Thread 1 is defined as follows: void run_thread1() { while (flag) { } print x; } Thread 2 is defined as follows: void run_thread2() { x = 60; flag = false; } Let us assume that, as there are no data dependencies between the variables flag and x, it is possible that a processor may reorder the instructions for Thread 2. Which of the following statements regarding the possible output of Thread 1 is TRUE? Thread 1 could enter an infinite loop The output could only be 50 The output could either be 50 or 60 None of the mentioned The output could only be 60arrow_forwardWrite a program using pthreads, which calculates the sum of elements in a hard-codedinteger array in parallel using 4 threads. The program must divide the work between 4threads which run simultaneously. For simplicity, you can assume that the size of thearray is 100. Note that the integer array must be declared as a global data structure.Initially code your solution so that the sum of elements is maintained in a global shared variable. Each thread modifies the same shared variable as it sums up elements fromthe array. Use a suitable synchronization primitive (mutex) to ensure safe access to theglobal variable. (A sample code of Mutex is attached for your reference)arrow_forwardWrite a multithreaded Hello World program using OpenMP. Have each thread say “Hello world” along with its thread ID and the number of threads the program is using.Run the program with as many threads as your computer will allow. Then try setting the thread count to a different number. please post:- The source code.- A screenshot of the program running.- A screenshot of the program running with a different thread count.arrow_forward
- Can you help me with this code because i don't know what to do with this code, this code has to be in C. question that I need help with:You need to use the pthread for matrix multiplication. Each threadfrom the threadpool should be responsible for computing only a partof the multiplication (partial product as shown in the above picture –all Ti(S) are called a partical product). Your main thread should splitthe matrices accordingly and create the partial data arrays that areneeded to compute each Ti. You must create a unique task with thedata and submit it to the job queue. You can compute the partialproducts concurrently as long as you have threads available in thethreadpool. You have to remove the task the from queue and submitto a thread in the threadpool. You should define the number ofthreads to be 5 and keep it dynamic so that we can test the samecode with a higher or lower number of threads as needed. When allthe partial products are computed all the threads in the…arrow_forwardPlease answer the follwoing regarding the java code: When looking at the code below, assume that the MyThread and MyRunnable classes are correctly implemented in other files. How many threads are there in the program shown below? (Be careful to consider ALL the threads!)arrow_forwardUSE SIMPLE PYTHON CODE TO COMPLETE Basic version with two levels of threads (master and slaves) One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please program to generate 1000 random integers to populate the array). The number of slave-threads is a parameter which the user can change. For example, if the user chooses 4 slave threads, each slave thread will sum 1000/4 = 250 numbers. If the user chooses 3 slave threads, the first two may each sum 333 numbers and the third slave threadsums the rest 334 numbers. 2) Advanced version with more than two levels of threadsThe master thread creates two slave-threads where each slave-thread is responsible to sum half segment of the array. Each slave thread will fork/spawn two new slave-threads where each new slave-threadsums half of the array segment received by its parent. Each slave thread will return the subtotal to its parent…arrow_forward
- Fill in the blanks:5. The ( 6 ) is used to implement mutual exclusion where it can be decremented by aprocess and incremented by another, but the value must either be 0 or 1.6. If deadlock prevention approach is used to deal with deadlocks in a system, the ( 7 )condition can be prevented using the direct method.7. Two threads may share the memory space, but they cannot share the same ( 8 )8. Consider round-robin (RR) scheduling algorithm is implemented with 2 seconds timeslice and it is now selecting a new process; if we have 3 blocked processes (A, B, and C),and A has been waiting the longest, then A would need to wait a period of ( 9 ) secondsto be selected.9. In real-time systems, if a task appears at random times, then it is considered ( 10 ).arrow_forwardTry pthreads.cpp. Modify it so that they run 3 threads (instead of two) and each thread runs a different function, displaying a different message. Copy-and-paste the source code and the outputs in your report. /* pthreads_demo.cpp A very simple example demonstrating the usage of pthreads. Compile: g++ -o pthreads_demo pthreads_demo.cpp -lpthread Execute: ./pthreads_demo */ #include <pthread.h> #include <stdio.h> using namespace std; //The thread void * thread_func (void *data) { char *tname = (char *) data; printf("My thread identifier is %s\n", tname); pthread_exit (0); } int main () { pthread_t id1, id2; //thread identifiers pthread_attr_t attr1, attr2; //set of thread attributes char *tnames[2] = { "Thread 1", "Thread 2" }; //names of threads //get the default attributes pthread_attr_init (&attr1); pthread_attr_init (&attr2); //create the threads pthread_create (&id1, &attr1, thread_func,…arrow_forwardPlease help with the following In Java:arrow_forward
- Modify this threading example to use, exclusively, multiprocessing, instead of threading. import threadingimport time class BankAccount(): def __init__(self, name, balance): self.name = name self.balance = balance def __str__(self): return self.name # These accounts are our shared resourcesaccount1 = BankAccount("account1", 100)account2 = BankAccount("account2", 0) class BankTransferThread(threading.Thread): def __init__(self, sender, receiver, amount): threading.Thread.__init__(self) self.sender = sender self.receiver = receiver self.amount = amount def run(self): sender_initial_balance = self.sender.balance sender_initial_balance -= self.amount # Inserting delay to allow switch between threads time.sleep(0.001) self.sender.balance = sender_initial_balance receiver_initial_balance = self.receiver.balance receiver_initial_balance += self.amount # Inserting delay to allow switch between threads time.sleep(0.001)…arrow_forwardProblem 0. Write a version of hello.c that creates and reaps (joins) n threads, each of which prints "Hello, world" and its thread id, where n is a command-line argument, and the thread function is passed a pointer to its thread ID as its argument.arrow_forwardExercise 1: Write a thread class TabPrinter that prints the elements of an array of integers (in one line) every 2 seconds 5 times. Use the way of extending the class thread. Write the main method which creates and starts three threads Printer which will print different arrays of integers. After that it prints "Main won’t wait. Main exits". Modify the above thread program so that you implement the interface Runnable. Make the main thread waiting till all other threads finish execution. ____________________________________________________________________________________________ Exercise 2: Write a thread class TextThread that prints a text every 1 second 10 times. Read the following main class. Try to guess what will be its output. class Test { public static void main(String s[]) throws Exception { TextThread x = new TextThread ("I am thread x"); TextThread y= new TextThread ("I am thread y"); System.out.println("I am Main thread"); } } Write it and execute it. Is the…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
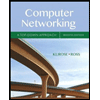
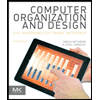
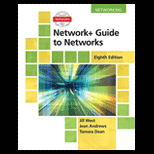
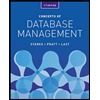
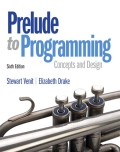
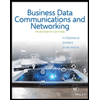