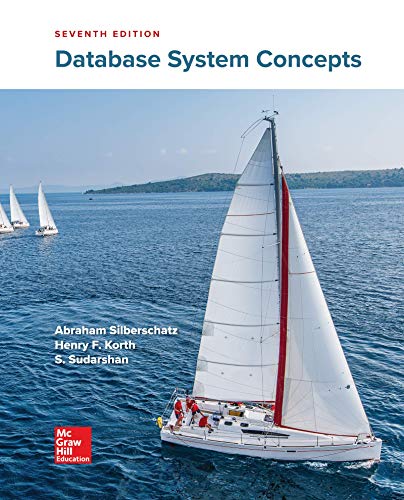
Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary class) and the other implemented using the built-in
Complete main() by inserting a new book into each list using the respective LinkedListLibrary and VectorLibrary InsertSorted() functions and outputting the number of book copy operations the computer must perform to insert the new book. Each InsertSorted() returns the number of book copy operations the computer performs.
Ex: If the input is:
Their Eyes Were Watching God 9780060838676 Zora Neale Hurston
the output is:
Number of linked list book copy operations: 1 Number of vector book copy operations: 95
Which list do you think will require the most operations? Why?
Main.cpp
#include "LinkedListLibrary.h"
#include "VectorLibrary.h"
#include "BookNode.h"
#include "Book.h"
#include <fstream>
#include <iostream>
using namespace std;
void FillLibraries(LinkedListLibrary &linkedListLibrary, VectorLibrary &vectorLibrary) {
ifstream inputFS; // File input stream
int linkedListOperations = 0;
int vectorOperations = 0;
BookNode* currNode;
Book tempBook;
string bookTitle;
string bookAuthor;
long long bookISBN;
// Try to open file
inputFS.open("books.txt");
while(getline(inputFS, bookTitle)) {
inputFS >> bookISBN;
inputFS.ignore(1, '\n');
getline(inputFS, bookAuthor);
// Insert into linked list
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
linkedListOperations = linkedListLibrary.InsertSorted(currNode, linkedListOperations);
// Insert into vector
tempBook = Book(bookTitle, bookAuthor, bookISBN);
vectorOperations = vectorLibrary.InsertSorted(tempBook, vectorOperations);
}
inputFS.close(); // close() may throw ios_base::failure if fails
}
int main () {
int linkedListOperations = 0;
int vectorOperations = 0;
// Create libraries
LinkedListLibrary linkedListLibrary = LinkedListLibrary();
VectorLibrary vectorLibrary;
// Fill libraries with 100 books
FillLibraries(linkedListLibrary, vectorLibrary);
// Create new book to insert into libraries
BookNode* currNode;
Book tempBook;
string bookTitle;
string bookAuthor;
long bookISBN;
getline(cin, bookTitle);
cin >> bookISBN;
cin.ignore();
getline(cin, bookAuthor);
// Insert into linked list
// No need to delete currNode, deleted by LinkedListLibrary destructor
currNode = new BookNode(bookTitle, bookAuthor, bookISBN);
// TODO: Call LL_Library's InsertSorted() method to insert currNode and return
// the number of operations performed
// Insert into VectorList
tempBook = Book(bookTitle, bookAuthor, bookISBN);
// TODO: Call VectorLibrary's InsertSorted() method to insert tempBook and return
// the number of operations performed
// TODO: Print number of operations for linked list
// TODO: Print number of operations for vector
}
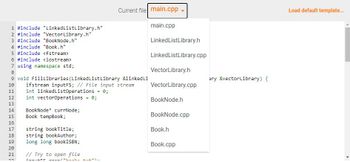

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- What is the biggest advantage of linked list over array? Group of answer choices Unlike array, linked list can dynamically grow and shrink With linked list, it is faster to access a specific element than with array Linked list is easier to implement than array Unlike array, linked list can only hold a fixed number of elementsarrow_forwardImplement a list of employees using a dynamic array of strings.Your solution should include functions for printing list, inserting and removingemployees, and sorting lists (use Bubble sort in C++ language).Keep list of employees sorted within the list after insertion.See the following example:How many employees do you expect to have?5 (Carriage Return)Menu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit2 (Carriage Return)Input name of new employee : MikeMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit1(Carriage Return)1 . MaryMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exitarrow_forwardC++ program for the following please: Create a generic function print(ls, n) that prints to standardoutput the first n elements of list ls. The elements are printed on separatelines. If ls has less than n elements, then the entire list is printedarrow_forward
- You are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forwardWhat would be a way in implementing the Josephus problem in C++ using a vector or a list? That takes in three parameters, one the number of knights for the knight parameter. The second parameter, "skip", is the number of knights to skip and to execute the next night. Then the third parameter "start", is the index of the knight to execute first.arrow_forwardProvide an explanation for the primary distinction that exists here between collection and an arraylist.arrow_forward
- Modify following delete() function so that it takes only two arguments, first and x without trail. void delete(listPointer *first, listPointer trail, listPointer x) { if (trail) trail -> link = x -> link; else *first = (*first) -> link; free(x); }arrow_forwardYou may do this assignment using either Java or C++. Do *not use the JDK LinkedList class or any linked list library! A linked list consists of zero or more nodes, which each contain one item of data and a link (Java reference, C++ pointer or reference) to the next node, if there is one. Linked List code is usually generic, so that you can use it to create a list of Strings, Students, Doubles, etc. For this assignment, you will write a simplified version of linked list that only can be used to create and use a list of ints and that has only a few of the functions typical of linked lists. The data element can be just an int, and the data type of the link to the next node can be just Node. You will need a reference (Java) or either a reference or a pointer (C++) to the first node, as well as one to the last node. Write a method/function that takes an in, creates a node with that int as its data value, and adds the node to the end of the list. This function will need to update the…arrow_forwardImplement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this. Use C++! The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to create an empty unsorted list add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. putItem () the numbers in the third line of the data file to the corresponding location in The list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one line…arrow_forward
- } (1 of 3 parts) Consider the function below that is given the head and tail pointers to a double linked list. bool patronum (Node ✶h, Node * t) { bool c = true; if (t nullptr) return true; Node* p = t; while (p != h) { Node* j = p; Node* r = p->prev; EXAM for (Node* r = p->prev; r != nullptr; r = r->prev) { if (j->value > r->value) { c = false; j = r; } swap(p->value, j->value); p = p->prev; return c;arrow_forwardWrite a template function that takes as parameter a vector of a generic type and reverses the order of elements in the vector, and then add the function to the program you wrote for Programming Challenge 5. Modify the driver program to test the new function by reversing and outputting vectors whose element types are char, int, double, and string.arrow_forwardTwo sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in ArrayList class (ArrayListLibrary arrayListLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and ArrayListLibrary insertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each insertSorted() returns the number of operations the computer performs. Ex: If the input is: The Catcher in the Rye 9787543321724 J.D. Salinger the output is: Number of linked list operations: 1 Number of ArrayList operations: 1 import java.util.Scanner;import java.io.FileInputStream;import java.io.IOException; public class Library { public static void fillLibraries(LinkedListLibrary linkedListLibrary, ArrayListLibrary arrayListLibrary) throws…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
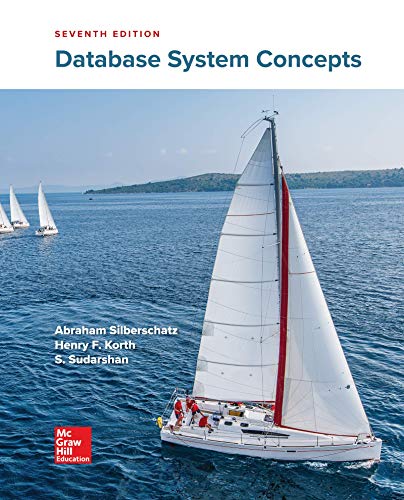
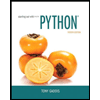
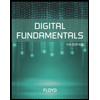
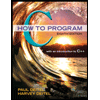
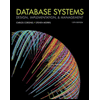
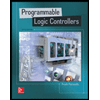