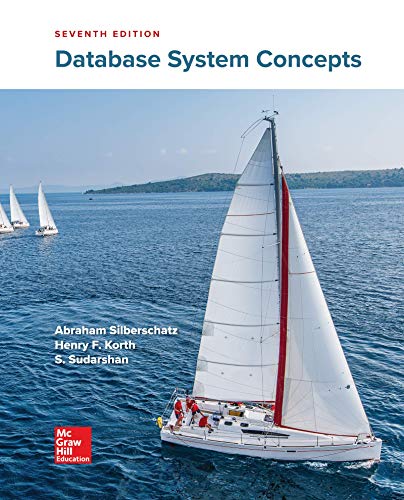
Concept explainers
use Advanced C++ techniques, containers and features to refactor the C/C++
replace appropriate declarations and code segments with Advanced C++ declarations and code.
* Replace all arrays with appropriate STL containers.
* Use STL algorithms to REPLACE existing logic where appropriate.
* Use smart and move pointers where pointers are needed.
* Use lambda expressions where appropriate.
* Use C++ style casting when needed.
* Look for opportunities where tuples could be used.
* The code listing is in C and C++.
If there are logic or syntax problems, please fix.
#define BIND(A,L,H) ((L)<(A)?(A)<(H)?(A):(H):(L))
char
dih[50],dah[50],medium[30],word[30],*dd[2] = {dih,dah};
const char
*ascii = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789.,?'!/()&:;=+-_\"$@",
*itu[] = {"13","3111","3131","311","1","1131","331","1111","11","1333","313","1311","33","31","333","1331","3313","131","111","3","113","1113","133","3113","3133","3311","33333","13333","11333","11133","11113","11111","31111","33111","33311","33331","131313","331133","113311","133331","313133","31131","31331","313313","13111","333111","313131","31113","13131","311113","113313","131131","1113113","133131"
};
void algorithm1(char* s,const char* m)
{
for (; *m; ++m)
strcat(s,dd['3'==*m]);
strcat(s,medium);
}
char* algorithm2(const char*i,char*o)
{
const char* pc;
sprintf(o,"beep");
for (; *i; ++i)
if (NULL == (pc = strchr(ascii,toupper(*i))))
strcat(o,word);
else
algorithm1(o,itu[pc-ascii]);
strcat(o,word);
return o;
}
/*
BIND(-1,0,9) is 0
BIND( 7,0,9) is 7
BIND(77,0,9) is 9
*/

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- Every data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardHow can I quickly copy a group of shared references from one array into another in C++? Create a list of potential responses to the challenge at hand. Does copying shared pointers also copy the objects they manage? Explainarrow_forwarduse Advanced C++ techniques, containers and features to refactor the C/C++ algorithm. The purpose is toreplace appropriate declarations and code segments with Advanced C++ declarations and code. * Replace all arrays with appropriate STL containers. * Use STL algorithms to REPLACE existing logic where appropriate. * Use smart and move pointers where pointers are needed. * Use lambda expressions where appropriate. * Use C++ style casting when needed. * Look for opportunities where tuples could be used. * The code listing is in C and C++. If you don't understand someof the C code or C functions, please google. If there are logic or syntax problems, please fix. int Algorithm(int a[], int x, int y) { int p, i, j = x; p = y; for(int i=x; i < y; i++) { if(a[i] < a[p]) { swap(&a[i], &a[j]); j++; } } swap(&a[p], &a[j]); return j;}arrow_forward
- Write some C++ code to sort an array of predefined data types using a template function.arrow_forwardneed help in C++ Problem: You are asked to create a program for storing the catalog of movies at a DVD store using functions, files, and user-defined structures. The program should let the user read the movie through the file, add, remove, and output movies to the file. For this assignment, you must store the information about the movies in the catalog using a single vector. The vector's data type is a user-defined structure that you must define on functions.h following these rules: Identifier for the user-define structure: movie. Member variables of the structure "movie": name (string), year (int), and genre (string). Note: you must use the identifiers presented before when defining the user-defined structure. Your solution will NOT pass the unit test cases if you do not follow the instructions presented above. The main function is provided (you need to modify the code of the main function to call the user-defined functions described below). The following user-defined functions are…arrow_forwardC++ Attached is the questionarrow_forward
- In C++, how can one easily duplicate a set of shared pointers into another array? Make a list of various solutions to the situation you've been given. Is it true that copying shared pointers also copies the objects they manage? Explainarrow_forwardHow can I quickly copy a group of shared references from one array into another in C++? Create a list of potential responses to the challenge at hand. Does copying shared pointers also copy the objects they manage? Explainarrow_forwardDevelop an algorithm and write a C++ program that counts the letter occurrence in the C-string; make sure you use call by reference to the array of a class with char and count as private member attributes when return from parse function. For the class member methods, provide the public accessor and modifier as well as print functions for the private member attributes. Write a test program that reads a C-string and displays the number of spaces, letters [a-z] [A-Z] and number of numbers [0-9] in the string. In addition to that, you need to output the histogram of the char and count array sorted by the ascii char ascending order. Here is a sample run of the program: <Output; double quote enclosure on string is required in the output> Enter a string: 2024 is coming The number of spaces in "2024 is coming" is 2 The number of letters in "2024 is coming" is 8 The number of numbers in "2024 is coming" is 4 ---- Histogram ---- Char Count space 2 0…arrow_forward
- use Advanced C++ techniques, containers and features to refactor the C/C++ algorithm. The purpose is toreplace appropriate declarations and code segments with Advanced C++ declarations and code. * Replace all arrays with appropriate STL containers. * Use STL algorithms to REPLACE existing logic where appropriate. * Use smart and move pointers where pointers are needed. * Use lambda expressions where appropriate. * Use C++ style casting when needed. * Look for opportunities where tuples could be used. * The code listing is in C and C++. If there are logic or syntax problems, please fix. char algorithm1(char c,int s){ if (isalpha(c)) { c = toupper(c); c = (((c - 65) + s) % 26) + 65; } return c;} int algorithm2(string input){ do { string output = ""; int shift = rand() % 26; for (int x = 0; x < input.length(); x++) output += algorithm1(input[x],shift); cout << output << endl; } while…arrow_forwardWrite a program which has a class called Character and data member which is a pointer to a character. Use appropriate data members to get input from the user and to perform linear search to locate an element in array pls code in c++arrow_forwardHow can C++ simply clone a collection of shared pointers into another array? List your options for the scenario. Do shared pointers transfer their managed objects? Explainarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
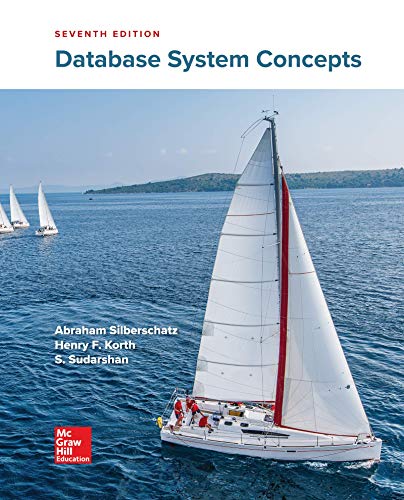
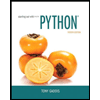
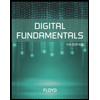
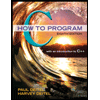
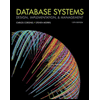
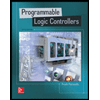