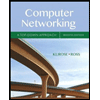
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a class called Dictionary that contains one instance variable representing the number of
definitions in the Dictionary. Write a constructor, setter, getter, and toString method.
Write a driver program named DictionaryTester that test each method in class Dictionary.
Expert Solution

arrow_forward
Step 1
#Class called Dictionary that contains one instance variable representing the number of
definitions in the Dictionary
import java.util.*;
class Dictionary
{
public static void main(String[] args)
{
my_dict.put("01", "Apple");
my_dict.put("10", "orange");
// using get() method
System.out.println("\nValue at key = 10 : " + my_dict.get("10"));
System.out.println("Value at key = 11 : " + my_dict.get("11"));
// using isEmpty() method
System.out.println("\nIs my dictionary empty? : " + my_dict.isEmpty() + "\n");
// using remove() method
// remove value at key 10
my_dict.remove("10");
System.out.println("Checking if the removed value exists: " + my_dict.get("10"));
System.out.println("\nSize of my_dict : " + my_dict.size());
}
}
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- The goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardWrite a method for the farmer class that allows a farmer object to pet all cows on the farm. Do not use arrays.arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- Please answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…arrow_forwardGoal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class The Recipe constructors so far did not specify how many servings the recipe is for. We will now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (see sample output below for an…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forward
- Lab 6 - Exercise 2. Write a test driver that reads data about two surgeons and print the specialization of the doctor with the highest salary using the method that compares the salary of surgeons. A Doctor class has the following private data members, constructor, and public methods: name (String) salary (int) End of document I A constructor without parameters, A constructor with parameters, A set method for the data variables (one method) A get method for each data members. A method to print Doctor information A Surgeon is a subclass of Doctor with the following private data members, constructor, and public methods: specialization (String) degree (string) A constructor without parameters, A constructor with parameters, · A set method for the data variables (one method) · A get method for each data member. · A method to print all Surgeon information. · A method that compares the salary of two Surgeons and return the specialization of the doctor with the higher salary. 1. Implement the…arrow_forwardWrite a class named Library. A library has the following attributes:• name: the name of this library.• employees: a list of LibraryEmployees that work at the library.• books: a dictionary that contains Books as keys. The value paired with each key is either anempty string, or a string representing the name of a person who has checked out the Book.The Library class should have an initializer that MAY accept employees and books as arguments,but doesn’t have to do so. If provided, these values should be assigned to the object’srespective attributes. If not provided, employees and books should begin as an empty list anddictionary, respectively. The value for the name attribute is always provided.The class should also have the following methods:• hire_employee(): takes in a new LibraryEmployee, and adds it to this library’s list ofemployees.• add_book(): takes in a new Book, and adds it to this library’s books (unless the libraryalready has this book). If the library already has the book,…arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward
- Goal 1: Update the Fractions Class Here we will overload two functions that will used by the Recipe class later: multipliedBy, dividedBy Previously, these functions took a Fraction object as a parameter. Now ADD an implementation that takes an integer as a parameter. The functionality remains the same: Instead of multiplying/dividing the current object with another Fraction, it multiplies/divides with an integer (affecting numerator/denominator of the returned object). For example; a fraction 2/3 when multiplied by 4 becomes 8/3. Similarly, a fraction 2/3 when divided by 4 becomes 1/6. Goal 2: Update the Recipe Class Now add a private member variable of type int to denote the serving size and initialize it to 1. This means all recipes are initially constructed for a single serving. Update the overloaded extraction operator (<<) to include the serving size (sample output below for an example of formatting) . New Member functions to the Recipe Class: 1. Add four member functions…arrow_forwardWrite a class named Library. A library has the following attributes:• name: the name of this library.• employees: a list of LibraryEmployees that work at the library.• books: a dictionary that contains Books as keys. The value paired with each key is either anempty string, or a string representing the name of a person who has checked out the Book.The Library class should have an initializer that MAY accept employees and books as arguments,but doesn’t have to do so. If provided, these values should be assigned to the object’srespective attributes. If not provided, employees and books should begin as an empty list anddictionary, respectively. The value for the name attribute is always provided.The class should also have the following methods:• hire_employee(): takes in a new LibraryEmployee, and adds it to this library’s list ofemployees.• add_book(): takes in a new Book, and adds it to this library’s books (unless the libraryalready has this book). If the library already has the book,…arrow_forwardWrite a class definition line and a one line docstring for the class Dog. Write an __init__ method for the class Dog that gives each dog its own name and breed. Test this on a successful creation of a Dog object.>>> import dog>>> sugar = dog.Dog('Sugar', 'border collie')>>> sugar.name'Sugar'>>> sugar.breed'border collie' Add a data attribute tricks of type list to each Dog instance and initialize it in __init__ to the empty list. The user does not have to supply a list of tricks when constructing a Dog instance. Make sure that you test this successfully.>>> sugar.tricks[] Write a method teach as part of the class Dog. The method teach should add a passed string parameter to tricks and print a message that the dog knows the trick.>>> sugar.teach('frisbee')Sugar knows frisbeearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
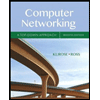
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
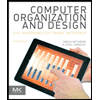
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
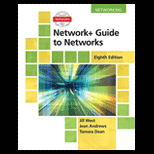
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
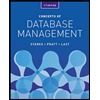
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
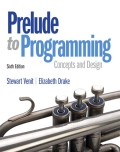
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
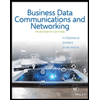
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY