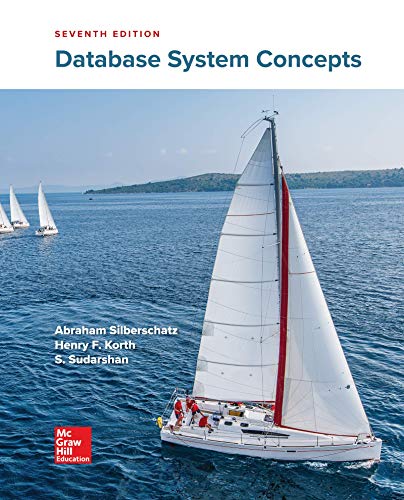
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a function removeDuplicates -PHP(
arr) that takes an associative array as a parameter and returns the same associative array except with all duplicate values removed. You may not use the function array_unique for this problem. For example:$arr = array( 'a' => "one", 'b' => "two", 'c' => "three", 'd' => "two", 'e' => "four", 'f' => "five", 'g' => "three", 'h' => "two" ); Expand (10 lines) print_r(removeDuplicates(
arr));
Would print:
Array ( [a] => one [e] => four [f] => five )
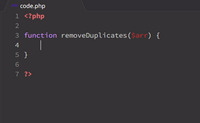
Transcribed Image Text:```php
<?php
function removeDuplicates($arr) {
}
?>
```
This is a basic PHP code snippet intended to define a function named `removeDuplicates`. This function takes one parameter, `$arr`, which is expected to be an array. The function currently has no implementation in its body, indicated by the empty block between the curly braces. To complete this function, you would need to add logic that processes the input array and removes duplicate values.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Similar questions
- Write in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the arrayarrow_forwardWrite a function named "SumPositive" that takes the following arguments: an array of integer values referred to as AArray; an integer Size that indicates the number of elements of AArray The function scans each position of the AArray[] and computes the sum of only the positive numbers. For example, if the array passed to the function looks like this: int AArray[] {1,-4,-2,2}; %3D SumPositive (AArray, 4); // the result returned is 3 int SumPositive(int AArray[], int Size) {arrow_forwardWrite a function that takes two string parameters and their common length. It fills out the second array with the capital form of the first parameter. Test your function in the main program.arrow_forward
- Create a function using Java: Number of Rows = 3-6 Number of Columns = 3-6 Function Name: winInDiagonalFSParameters: board: 2D integer array, piece: integerReturn: booleanAssume board is valid 2D int array, piece is X==1/O==2.Look at all forward slash / diagonals in the board. If any forward slash / diagonals has at least 3consecutive entries with given type of piece (X/O) (3 in a row XXX, OOO), then return true,otherwise falsearrow_forwardWrite a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forwardJAVASCRIPT Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console.arrow_forward
- Create a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forwardWrite a function named swapFrontback that takes as input an array ofintegers and an integer that specifies how many entries are in the array. Thefunction should swap the first element in the array with the last elements inthe array. The function should check if the array is empty to prevent errors.The header file for the swapFrontback() is:swapFrontback.h:double swapFrontback (int [] arr,int size);Write a driver (main.c) to test your function with arrays of different lengthand with varrying front and back numbers. Print the array elements beforeand after the swap in the main.c.Sample Run: Original Array: 1 2 3 4 5 6 FrontBack Array: 6 2 3 4 5 1arrow_forwardWrite 5 JUnit test cases to test a function designed to show the smallest and largest integers in an array. The function name is SmallLargeInt and the return type is int. JUnit test cases include 1. Array is null 2. Array is empty 3. Array has a single value 4. Array has multiple values 5. Array has positive and negative valuesarrow_forward
- C++ Write a function named “checkInvalidHours” that accepts an array of PaySubobjects and its size. It will go through the array and check for invalid hours(negative values). If it is negative, it will reset the hourly payrate to 0. It willreturn how many PayStub objects that it has reset the payrate to 0.Please show you would call and test this function.arrow_forwardWrite a function called remove_punct() that accepts an array of characters and the number of items in the array as parameters, removes the punctuation (',', '!', '.') characters from the array, and returns the number of punctuation characters removed. For example, if the array contains ['C', 'p', 't', 'S', ',', '1', '2', '1', '.', 'i', 's', 'f', 'u', 'n', '!'], then the function should remove the punctuation characters. The function must remove the characters by shifting all characters to the right of each punctuation character, left by one spot in the array. This will overwrite the punctuation characters, resulting in: ['C', 'p', 't', 'S', '1', '2', '1', 'i', 's', 'f', 'u', 'n']. In this case, the function returns 3. Note: if the array does not contain any punctuation characters, then the array is unchanged and the function returns 0.arrow_forwardDefine a function named “getExamListInfo” that accepts an array of Exam objects and its size. It will return the following information to the caller: - The number of exams with perfect score - The number of exams with “Pass” status - The index of the Exam object in the array that has the largest score. For example, if you get the exam info for this array of Exam object Exam examList[] = { {"Midterm1 Exam", 90}, {"Midterm2 Exam", 80}, {"Final Exam", 50}, {"Extra Credit", 100}, {"Initial Test", 0}, {"Homework1", 69} } ; You will get Perfect Count: 1 Pass Count: 3 Index of the largest: 3 C++arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
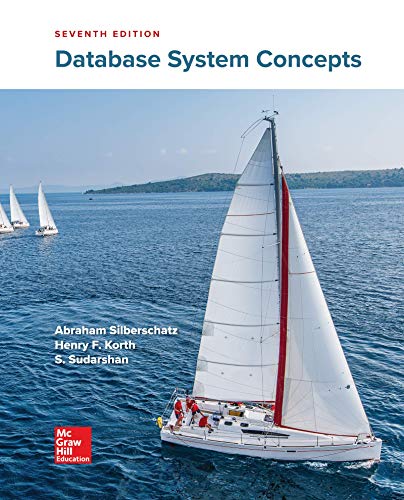
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
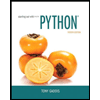
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
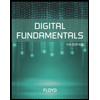
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
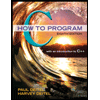
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
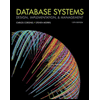
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
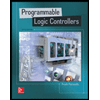
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education