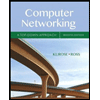
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
import java.util.*;
public class Budgeter {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
please use java and start the code the way provided and do NOT use \n or printf in the code please.
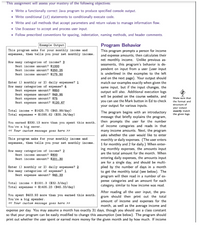
Transcribed Image Text:This assignment will assess your mastery of the following objectives:
• Write a functionally correct Java program to produce specified console output.
• Write conditional (if) statements to conditionally execute code.
• Write and call methods that accept parameters and return values to manage information flow.
• Use Scanner to accept and process user input.
• Follow prescribed conventions for spacing, indentation, naming methods, and header comments.
Example Output
This program asks for your monthly income and
Program Behavior
This program prompts a person for income
expenses, then tells you your net monthly income. and expense amounts, then calculates their
net monthly income. Unlike previous as-
sessments, this program's behavior is de-
pendent on input from a user (user input
is underlined in the examples to the left
and on the next page). Your output should
match our examples exactly when given the
same input, but if the input changes, the
output will also. Additional execution logs
will be posted on the course website, and
you can use the Mark button in Ed to check
your output for various inputs.
How many categories of income? 3
Next income amount? $1000
Next income amount? $250.25
Next income amount? $175.50
Enter 1) monthly or 2) daily expenses? 1
How many categories of expense? 4
Next expense amount? $850
Next expense amount? $49.95
Next expense amount? $75
Next expense amount? $120.67
Make sure that
the format and
Total income - $1425.75 ($45.99/day)
Total expenses = $1095.62 ($35.34/day)
structure of
your output
exactly match
the given logs.
The program begins with an introductory
message that briefly explains the program,
You earned $330.13 more than you apent this month. then prompts the user for the number
You're a big saver.
« Your custom message goes here >>
of income categories and reads in that
many income amounts. Next, the program
asks whether the user would like to enter
This program asks for your monthly income and
expenses, then tells you your net monthly income.
monthly or daily expenses. (The user enters
1 for monthly and 2 for daily.) When enter-
ing monthly expenses, the amounts input
are the total amount for the month. When
Hov many categories of income? 2
Next income amount? $800
entering daily expenses, the amounts input
are for a single day, and should be multi-
plied by the number of days in a month
to get the monthly total (see below). The
program will then read in a number of ex-
pense categories and an amount for each
category, similar to how income was read.
Next income amount? $201.30
Enter 1) monthly or 2) daily expenses? 2
How many categories of expense? 1
Next expense amount? $45.33
Total income - $1001.3 ($32.3/day)
Total expenses = $1405.23 ($45.33/day)
After reading all the user input, the pro-
You spent $403.93 more than you earned this month. gram should then print out the total
You're a big spender.
« Your custom message goes here >»
amount of income and expenses for the
month, as well as the average income and
expense per day. You may assume a month has exactly 31 days, though you should use a class constant
so that your program can be easily modified to change this assumption (see below). The program should
print out whether the user spent or earned more money for the given month and by how much. If income
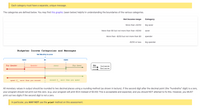
Transcribed Image Text:Each category must have a separate, unique message.
The categories are defined below. You may find this graphic (seen below) helpful in understanding the boundaries of the various categories.
Net income range
Category
More than +$250
big saver
More than $0 but not more than than +$250
saver
More than -$250 but not more than $0
spender
-$250 or less
big spender
Budgeter Income Categories and Messages
Net Monthly Income
-$250
$0
+$250
Big Spender
Spender
Saver
Big Saver
Key
Inclusive
Exclusive
spent $ more than you earned
earned $ more than you spent
All monetary values in output should be rounded to two decimal places using a rounding method (as shown in lecture). If the second digit after the decimal point (the "hundreths" digit) is a zero,
your program should not print out this zero. (e.g. your program will print $3.5 instead of $3.50) This is acceptable and expected, and you should NOT attempt to fix this. However, you MUST
print out two digits if the second digit is not a zero.
In particular, you MAY NOT use the printf method on this assessment.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- T/F 1. Java methods can only return primitive typesarrow_forwardHow do I fix the Java code errors? Code: //import java.util.Arrays;//Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() {…arrow_forwardimport java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forward
- PROBLEM STATEMENT: Use string concatenation to append the "dogs" string to the parameter str. public class StringConcatAppend{public static String solution(String str){// ↓↓↓↓ your code goes here ↓↓↓↓return null; Can you help me with this question The language is Javaarrow_forwardusing System; using System.Linq; class main { publicstaticvoid Main (string[] args) { Console.WriteLine("Hi, my name is Stevie. I am thinking of an Animal.\nGuess which animal I am thinking of> "); string[] words = newstring[7]{"sheep","goat","lion","horse","dog","cat","lizard"}; Random rnd = new Random(); int random = rnd.Next(0, 5); string secretWord = words[random]; secretWord = secretWord.ToUpper(); int lives = 5; int counter = -1; int wordLength = secretWord.Length; char[] secretArray = secretWord.ToCharArray(); char[] printArray = newchar[wordLength]; char[] guessedLetters = newchar[26]; int numberStore = 0; bool victory = false; foreach(char letter in printArray) { counter++; printArray[counter] = '-'; } while(lives > 0) { counter = -1; string printProgress = String.Concat(printArray); bool letterFound = false; int multiples = 0; if (printProgress == secretWord) { victory = true; break; } if (lives > 1) { Console.WriteLine("You have {0} lives!", lives); } else {…arrow_forwardCorrect Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forward
- 1. Insert the missing part of the code below to output the string. public class MyClass { public static void main(String[] args) { ("Hello, I am a Computer Engineer");arrow_forwardA technique that allows a researcherto determine the greatest amount of material that can move through anetwork is called __________. maximal-flowmaximal-spanningshortest-routemaximal-treearrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forward
- public class Test { } public static void main(String[] args) { String str = "Salom"; System.out.println(str.indexOf('t)); }arrow_forwardpublic class Animal { public static int population; private int age; } public Animal (int age) { this.age = age; population++; } + msg); public void say (String msg) { System.out.print("Saying: " System.out.print(" for "); System.out.print(getHuman Years()); System.out.println(" years."); } public int getHuman Years() { return age; } public class Mammal extends Animal { private String species; public Mammal(String species, int age) { super (age); this. species species; } } } = public int getHuman Years() { if (species.equals("dog")) 1 else return super.getHumanYears() * 7; return super.getHumanYears(); €arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { Console.WriteLine(number); number = number + 2; } Random r = new Random(); int randNo= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo); } } how would this program be modified to choose three random numbers instead of just one number between 1-88?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
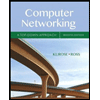
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
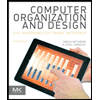
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
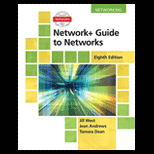
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
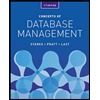
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
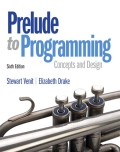
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
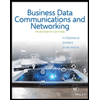
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY