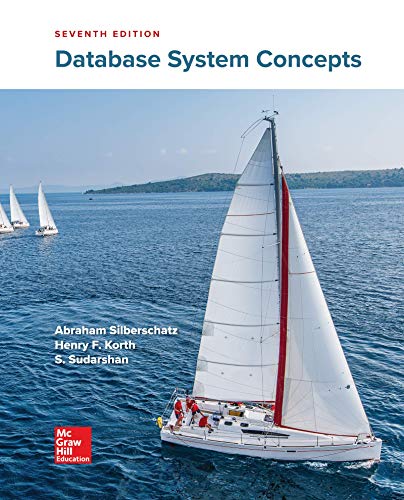
Concept explainers
Write a Pep/9 assembly language program that reads an integer value from the keyboard, and displays a table that shows the quotient when the input value is divided by successive powers of 2, between 2 and 32. In other words, your program should produce exactly the same output as the following C program:
#include <stdio.h> int inpVal; int divis; int quotient; int main() { printf("? "); scanf("%d", &inpVal); divis = 2; quotient = inpVal / divis; printf("%d / %d = %d\n", inpVal, divis, quotient); divis *= 2; quotient /= 2; printf("%d / %d = %d\n", inpVal, divis, quotient); divis *= 2; quotient /= 2; printf("%d / %d = %d\n", inpVal, divis, quotient); divis *= 2; quotient /= 2; printf("%d / %d = %d\n", inpVal, divis, quotient); divis *= 2; quotient /= 2; printf("%d / %d = %d\n", inpVal, divis, quotient); } // end of main
Example of Input and Output format requirements:
For this example, assume that the keyboard input given to the program is:
8209
For this input data, the output report must look like this:
? 8209
8209 / 2 = 4104
8209 / 4 = 2052
8209 / 8 = 1026
8209 / 16 = 513
8209 / 32 = 256
**************************** IMP I need in this below example in Assembly Language format please don't answer in other ways********************************************
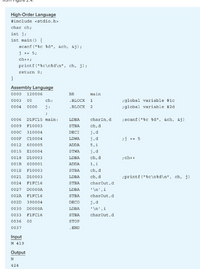

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
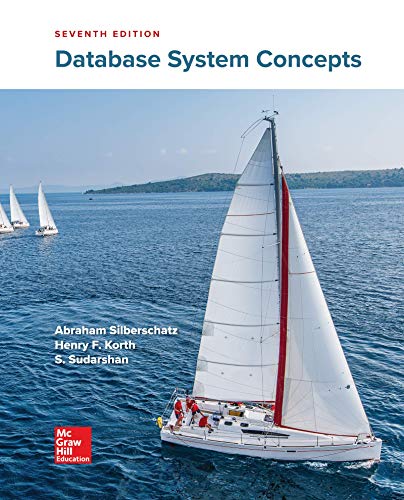
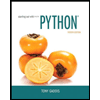
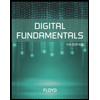
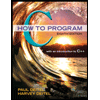
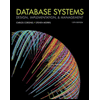
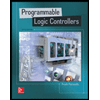