Write a test program named TestApartment that: • Creates a CanadianAddress object for the following address: "1030 South Park St., Halifax, Nova Scotia B3H 2W3". • Creates an Apartment object with the name "Somerset Place Apartment", the above CanadianAddress object, 100,000 for square footage and 100 for the total of units. • Print the information about the Apartment object, using the format in the sample run. Use the getter methods to obtain the information. • Use the proper setter method to set the square footage of the Apartment object to 120,000. • Use the proper setter method to set the total units of the Apartment object to 120. • Print the updated information about the Apartment object, using the format in the sample run. Use the getter methods to obtain the information. Here is the sample run: The apartment is created with the following information: Name: Somerset Place Apartment Address: 1030 South Park St., Halifax, Nova Scotia B3H 2W3, Canada Square footage: 100000 Total units: 100 The apartment is updated to the following information: Name: Somerset Place Apartment Address: 1030 South Park St., Halifax, Nova Scotia B3H 2W3, Canada Square footage: 120000 Total units: 120 Additional requirements: Use separate files for each class. You should have the following four source files: CanadianAddress.java, Building.java, Apartment.java, TestApartment.java. Use proper visibility modifiers for your classes.
Write a test program named TestApartment that: • Creates a CanadianAddress object for the following address: "1030 South Park St., Halifax, Nova Scotia B3H 2W3". • Creates an Apartment object with the name "Somerset Place Apartment", the above CanadianAddress object, 100,000 for square footage and 100 for the total of units. • Print the information about the Apartment object, using the format in the sample run. Use the getter methods to obtain the information. • Use the proper setter method to set the square footage of the Apartment object to 120,000. • Use the proper setter method to set the total units of the Apartment object to 120. • Print the updated information about the Apartment object, using the format in the sample run. Use the getter methods to obtain the information. Here is the sample run: The apartment is created with the following information: Name: Somerset Place Apartment Address: 1030 South Park St., Halifax, Nova Scotia B3H 2W3, Canada Square footage: 100000 Total units: 100 The apartment is updated to the following information: Name: Somerset Place Apartment Address: 1030 South Park St., Halifax, Nova Scotia B3H 2W3, Canada Square footage: 120000 Total units: 120 Additional requirements: Use separate files for each class. You should have the following four source files: CanadianAddress.java, Building.java, Apartment.java, TestApartment.java. Use proper visibility modifiers for your classes.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 17RQ
Related questions
Question
Write the code in java and here is the lab 12 code read the question why I gave you lab 12 code :
public class lab12daneshelahi
{
public static void main (String[]args)
{
CanadianAddress testAddress = new CanadianAddress ();
CanadianAddress SMUAddress =
new CanadianAddress ("923 Robie St.", "Halifax", "Nova Scotia",
"B3H 3C3");
System.out.println ("The testAddress is created as:");
testAddress.printAddress ();
System.out.println ();
System.out.println ("The SMUAddress is created as:");
SMUAddress.printAddress ();
System.out.println ();
System.out.println ("The testAddress is equal to SMUAddress? :( " +
testAddress.equalAddress (SMUAddress));
System.out.println ();
testAddress.setStreetAddress ("923 Robie St.");
testAddress.setCity ("Halifax");
testAddress.setProvince ("Nova Scotia");
testAddress.setPostalCode ("B3H 3C3");
System.out.println ("The testAddress is updated as: :D ");
testAddress.printAddress ();
System.out.println ();
System.out.println ("The testAddress is equal to SMUAddress? :) " +
testAddress.equalAddress (SMUAddress));
}
}
class CanadianAddress
{
private String streetAddress;
private String city;
private String province;
private String postalCode;
public CanadianAddress ()
{
}
public CanadianAddress (String streetAddress, String city, String province,
String postalCode)
{
this.streetAddress = streetAddress;
this.city = city;
this.province = province;
this.postalCode = postalCode;
}
public String getStreetAddress ()
{
return streetAddress;
}
public void setStreetAddress (String streetAddress)
{
this.streetAddress = streetAddress;
}
public String getCity ()
{
return city;
}
public void setCity (String city)
{
this.city = city;
}
public String getProvince ()
{
return province;
}
public void setProvince (String province)
{
this.province = province;
}
public String getPostalCode ()
{
return postalCode;
}
public void setPostalCode (String postalCode)
{
this.postalCode = postalCode;
}
public void printAddress ()
{
System.out.println ((streetAddress != null ? streetAddress : "null") +
", " + (city != null ? city : "null") + ",");
System.out.println ((province != null ? province : "null") + " " +
(postalCode !=
null ? postalCode : "null") + ", Canada");
}
public boolean equalAddress (CanadianAddress address)
{
return (this.streetAddress == null ? address.streetAddress ==
null : this.streetAddress.equals (address.streetAddress))
&& (this.city == null ? address.city ==
null : this.city.equals (address.city))
&& (this.province == null ? address.province ==
null : this.province.equals (address.province))
&& (this.postalCode == null ? address.postalCode ==
null : this.postalCode.equals (address.postalCode));
}
}
Please dont plagarise or copy from other sources do what in the question says and follow what in the question says
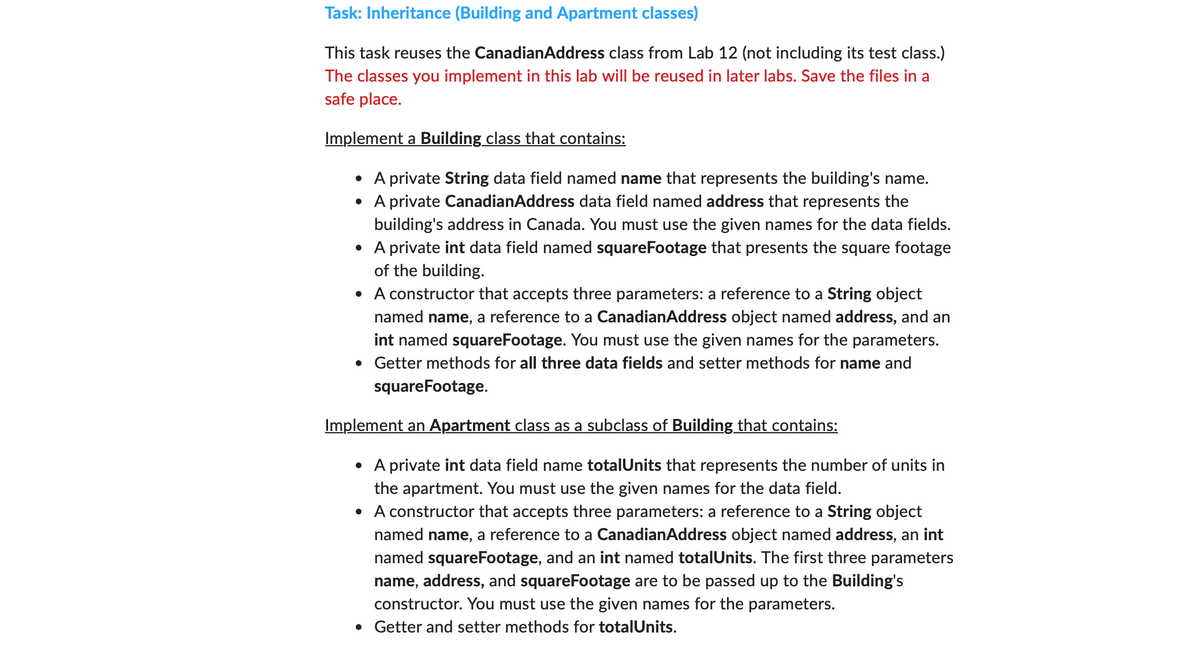
Transcribed Image Text:Task: Inheritance (Building and Apartment classes)
This task reuses the CanadianAddress class from Lab 12 (not including its test class.)
The classes you implement in this lab will be reused in later labs. Save the files in a
safe place.
Implement a Building class that contains:
• A private String data field named name that represents the building's name.
• A private CanadianAddress data field named address that represents the
building's address in Canada. You must use the given names for the data fields.
• A private int data field named squareFootage that presents the square footage
of the building.
• A constructor that accepts three parameters: a reference to a String object
named name, a reference to a CanadianAddress object named address, and an
int named squareFootage. You must use the given names for the parameters.
• Getter methods for all three data fields and setter methods for name and
squareFootage.
Implement an Apartment class as a subclass of Building that contains:
• A private int data field name totalUnits that represents the number of units in
the apartment. You must use the given names for the data field.
• A constructor that accepts three parameters: a reference to a String object
named name, a reference to a CanadianAddress object named address, an int
named squareFootage, and an int named totalUnits. The first three parameters
name, address, and squareFootage are to be passed up to the Building's
constructor. You must use the given names for the parameters.
• Getter and setter methods for totalUnits.
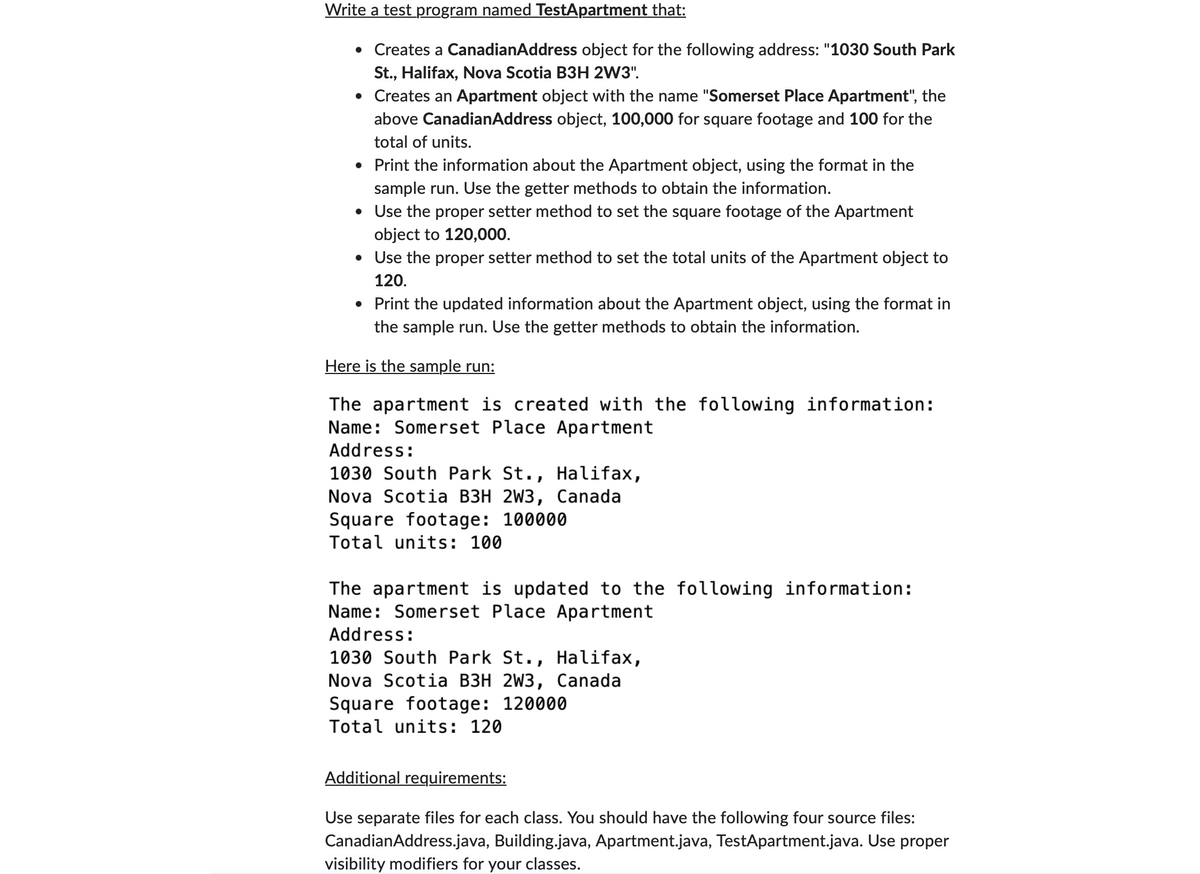
Transcribed Image Text:Write a test program named TestApartment that:
.
Creates a CanadianAddress object for the following address: "1030 South Park
St., Halifax, Nova Scotia B3H 2W3".
• Creates an Apartment object with the name "Somerset Place Apartment", the
above CanadianAddress object, 100,000 for square footage and 100 for the
total of units.
• Print the information about the Apartment object, using the format in the
sample run. Use the getter methods to obtain the information.
• Use the proper setter method to set the square footage of the Apartment
object to 120,000.
• Use the proper setter method to set the total units of the Apartment object to
120.
• Print the updated information about the Apartment object, using the format in
the sample run. Use the getter methods to obtain the information.
Here is the sample run:
The apartment is created with the following information:
Name: Somerset Place Apartment
Address:
1030 South Park St., Halifax,
Nova Scotia B3H 2W3, Canada
Square footage: 100000
Total units: 100
The apartment is updated to the following information:
Name: Somerset Place Apartment
Address:
1030 South Park St., Halifax,
Nova Scotia B3H 2W3, Canada
Square footage: 120000
Total units: 120
Additional requirements:
Use separate files for each class. You should have the following four source files:
CanadianAddress.java, Building.java, Apartment.java, TestApartment.java. Use proper
visibility modifiers for your classes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step 1: Algorithm
VIEWStep 2: CanadianAddress.java Class - Program
VIEWStep 3: CanadianAddress.java Class - Screenshot
VIEWStep 4: Building.java class - Program
VIEWStep 5: Building.java class - Screenshot
VIEWStep 6: Apartment.java class - Program
VIEWStep 7: Apartment.java class - Screenshot
VIEWStep 8: TestApartment.java Class - Program
VIEWStep 9: TestApartment.java Class - Screenshot
VIEWSolution
VIEWStep by step
Solved in 10 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
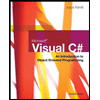
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
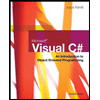
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,