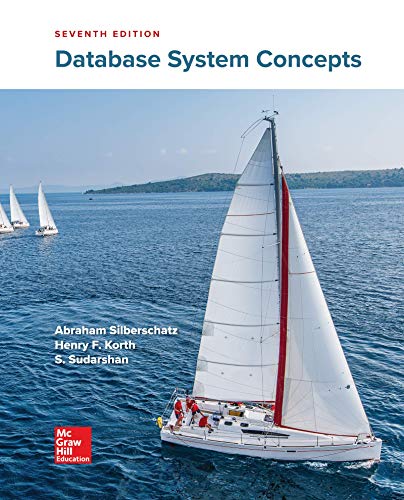
Write a Fraction class whose objects will represent fractions.
#include <iostream>
using namespace std;
class Fraction
{
public:
int numerator;
int denominator;
void set(int n ,int d);
void print();
Fraction multipliedBy(Fraction f);
Fraction dividedBy(Fraction f);
Fraction addedTo(Fraction f);
Fraction subtract(Fraction f);
bool isEqualTo(Fraction f);
};
void Fraction::set(int n, int d)
{
numerator = n;
denominator = d;
}
void Fraction::print()
{
cout << numerator << "/" << denominator;
}
Fraction Fraction::multipliedBy(Fraction f)
{
Fraction results;
results.numerator = (this->numerator *f.denominator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::dividedBy(Fraction f)
{
Fraction results;
results.numerator =(this->numerator * f.denominator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::addedTo(Fraction f)
{
Fraction results;
results.numerator =(this->numerator * f.denominator) + (this->denominator * f.numerator);
results.denominator=this->denominator * f.numerator;
return results;
}
Fraction Fraction::subtract(Fraction f)
{
Fraction results;
results.numerator = (this->numerator * f.denominator)- (this->denominator * f.numerator);
results.denominator=this->denominator * f.numerator;
return results;
}
bool Fraction::isEqualTo(Fraction f)
{
if ( this->numerator * f.denominator == this->denominator * f.numerator)
return true;
else
return false;
}
int main()
{
Fraction f1;
Fraction f2;
Fraction result;
f1.set(9, 8);
f2.set(2, 3);
cout << "The product of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.multipliedBy(f2);
result.print();
cout << endl;
cout << "The quotient of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.dividedBy(f2);
result.print();
cout << endl;
cout << "The sum of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.addedTo(f2);
result.print();
cout << endl;
cout << "The difference of ";
f1.print();
cout << " and ";
f2.print();
cout << " is ";
result = f1.subtract(f2);
result.print();
cout << endl;
if (f1.isEqualTo(f2)){
cout << "The two Fractions are equal." << endl;
} else {
cout << "The two Fractions are not equal." << endl;
}
}
current output
The product of 9/8 and 2/3 is 27/16
The quotient of 9/8 and 2/3 is 27/16
The sum of 9/8 and 2/3 is 43/16
The difference of 9/8 and 2/3 is 11/16
The two Fractions are not equal.
Output is suppose to look like:
The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal.
The product of 9/8 and 2/3 is 18/24 The quotient of 9/8 and 2/3 is 27/16 The sum of 9/8 and 2/3 is 43/24 The difference of 9/8 and 2/3 is 11/24 The two Fractions are not equal.

Step by stepSolved in 2 steps with 1 images

- class Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardJava:arrow_forward2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forward
- Java Programming Please do not change anything in Student class or Course class class John_Smith extends Student{ public John_Smith() { setFirstName("John"); setLastName("Smith"); setEmail("jsmith@jaguar.tamu.edu"); setGender("Male"); setPhoneNumber("(200)00-0000"); setJNumber("J0101459"); } class MyCourse extends Course { public MyCourse { class Course { private String courseNumber; private String courseName; private int creditHrs; public Course (String number, String name, int creditHrs){ this.courseNumber = number; this.courseName = name; this.creditHrs = creditHrs; } public String getNumber() { return courseNumber; } public String getName() { return courseName; } public int getCreditHrs() { return creditHrs; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseName(String…arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardPublic classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forward
- Fill out the starter codearrow_forwardinterface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forwardC# Solve this error using System; namespace RahmanA3P1BasePlusCEmployee { public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; // gross weekly sales private decimal commissionRate; // commission percentage private decimal baseSalary; // base salary per week // six-parameter constructor public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { // implicit call to object constructor occurs here FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; // validates gross sales CommissionRate = commissionRate; // validates commission rate BaseSalary = baseSalary; // validates base…arrow_forward
- C++arrow_forwardclass implementation file -- Rectangle.cpp class Rectangle { #include #include "Rectangle.h" using namespace std; private: double width; double length; public: void setWidth (double); void setLength (double) ; double getWidth() const; double getLength() const; double getArea () const; } ; // set the width of the rectangle void Rectangle::setWidth (double w) { width = w; } // set the length of the rectangle void Rectangle::setLength (double l) { length l; //get the width of the rectangle double Rectangle::getWidth() const { return width; // more member functions herearrow_forward/* (name header)*/public class BatteryTester{ public static void main(String[] args) { //=========================battery1============================ //Create a Battery object called battery1 using the default constructor System.out.println("=====Battery1====="); //Print the max capacity and the current capacity of battery1 //Check if the battery is full and print the result. You need to call the method isFull() //Drain the battery by 25.5 mAh //Print the current capacity of battery1 after draining //=========================battery2============================ //Create a Battery object called battery2 using the overloaded constructor and set the current capacity and //max capacity to 2000 mAh. System.out.println("\n=====Battery2====="); //Print the max capacity and the current capacity of battery2 //Drain the battery by 2200 mAh //Print…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
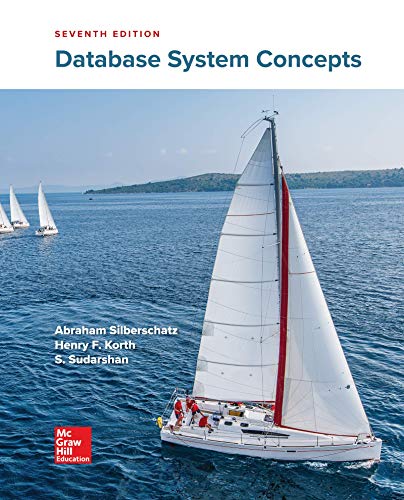
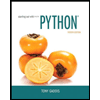
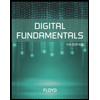
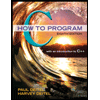
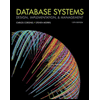
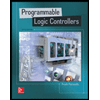