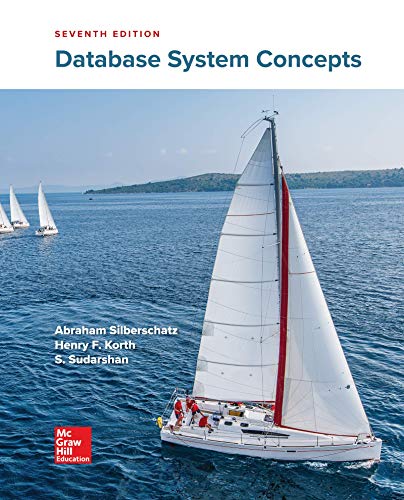
Write (define) a public static method named countInRange, that takes an ArrayList of Integer, and two additional intarguments and returns (as an int) a count of the number values in the ArrayList that are between the second and third argument values (inclusive). You can safely assume that the third argument value will always be greater than or equal to the second argument value.
For example:
given an ArrayList<Integer> named myList that contains this list of values: {1, 2, 1, 3, 2, 5, 6, 2, 4}
countInRange(myList, 1, 6) should return 9
countInRange(myList, 2, 4) should return 5
countInRange(myList, 10, 20) should return 0
--------------------------------------------------------------------
public class Main {
public static void main(String[] args) {
// you may wish to write some code in this main method
// to test your method.
}
<your method definition here>
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- // Only few methods are mentioned here //Do remaining methods to pass the test methods /*** Removes the specified (num) of the given element from the internal ArrayList of elements. * If num <= 0, don't remove anything. * If num is too large, remove all instances of the given element from the internal ArrayList of elements.* Example:* - For a defined CustomIntegerArrayList containing the integers: 1, 2, 1, 2, 1* - Calling remove(2, 1) would remove the first 2 instances of 1 * - The CustomIntegerArrayList will then contain the integers: 2, 2, 1* - For a defined CustomIntegerArrayList containing the integers: 100, 100, 100 * - Calling remove(4, 100) would remove all instances of 100 * - The CustomIntegerArrayList will then be empty* - For a defined CustomIntegerArrayList containing the integers: 5, 5, 5, 5, 5 * - Calling remove(0, 5) would remove nothing* * @param num number of instances of element to remove * @param element to remove*/ public void remove(int num, int element) {//…arrow_forwardin java programming answer the following: Given an array called myArr (created as ArrayList<T>) and contains the {1,2,3,4,5,6,7}. (a) what would be the contents of this array after myArr.add(10). b) write the content of myArr after myArr.add(4, 11);arrow_forwardJava Complete a method calledRedundantCharacterMatch(ArrayList<Character> YourFirstName): the parameter of this method is an ArrayList<Character> whose elements are the characters in your first name (they should be in the order appear in your first name, e.g., if your first name is bob, then the ArrayList<Char> includes ‘b’, ‘o’, ‘b’.). The method will check whether there exists duplicate characters in your name and return the index of those duplicate characters. For example, when using bob as first name, it will return b: 0, 2. 2. Create an ArrayList<Character> NameExample. All the characters of your first name will appear twice in this ArrayList. For example, if your first name is bob, then NameExample will include the following element {b,o,b,b,o,b}. Then, please use NameExample as parameter for the method RedundantCharacterMatch(). If your first name is bob, the results that print in the console will be b: 0, 2, 3, 5 o: 1, 4arrow_forward
- How do you do this? JAVAarrow_forwardjava Create a static method that: is called repeatAll returns ArrayList of Booleans takes in a single parameter - an ArrayList of Booleans This method should modify its ArrayList parameter by repeating its ArrayList values. For example, if the parameter is (true, false, false) The modified ArrayList should be (true, false, false, true, false, false) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Boolean> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextBoolean()); } System.out.println(repeatAll(list)); } }arrow_forwardjava Create a method that: is called timesTwo returns an ArrayList of Integers takes in a single parameter - an ArrayList of Integers called nums This method should take the ArrayList parameter and multiply every value by two. public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(timesTwo(list)); } }arrow_forward
- Write a public static method named allLessThanMean that will take an ArrayList<Integer> as an argument. This method will return an ArrayList<Integer>. When called and passed anArrayList, this method will return an ArrayList containing all the elements in the argument ArrayList that are less than the average of all the values in the argument ArrayList. The values in the returned ArrayList must be in the same order as they are in the argument ArrayList. Here are some examples: Example 1 Given: ArrayList<Integer> myList = new ArrayList<Integer>(); with these values {1, 2, 3, 4, 5}; allLessThanMean(myList) should return an ArrayList<Integer> with these values {1, 2} Example 2 Given: ArrayList<Integer> myList = new ArrayList<Integer>(); with these values {3, 7, 6, 2, 9, 0, 4, 8}; allLessThanMean(myList) should return an ArrayList<Integer> with these values {3, 2, 0, 4} Example 3 Given: ArrayList<Integer> myList = new…arrow_forwardjava Create a static method that: is called appendPosSum returns an ArrayList of Integers takes one parameter: an ArrayList of Integers This method should: Create a new ArrayList of Integers Add only the positive Integers to the new ArrayList Sum the positive Integers in the new ArrayList and add the Sum as the last element For example, if the incoming ArrayList contains the Integers (4,-6,3,-8,0,4,3), the ArrayList that gets returned should be (4,3,4,3,14), with 14 being the sum of (4,3,4,3). The original ArrayList should remain unchanged. public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(appendPosSum(list));arrow_forwardWrite a class ArrayClass, where the main method asks the user to enter the four numbers. Create a method printArray that prints the array whenever required (use Array.toString method). Make the program run as shown in the Sample Run below. Use Array.sort, Arrach.bianrySearch, Array.equalsarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
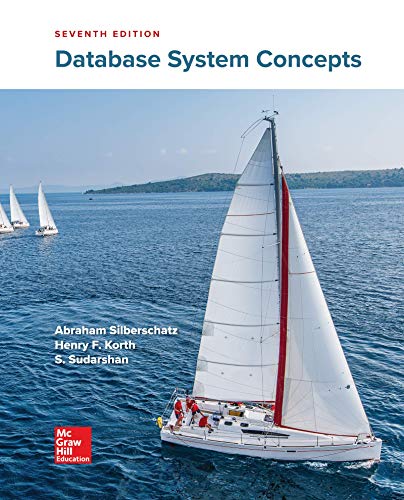
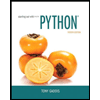
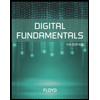
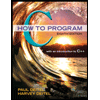
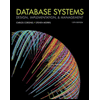
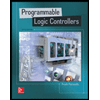