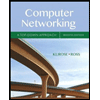
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
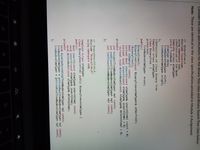
Transcribed Image Text:Consider the class specifications for the Binary Tree class and Binary Search Tree class below:
Note: These are identical to the class specifications provided in Module 6 Assignment
// BinaryTree.h
#include <iostream>
using namespace std;
//Definition of the Node
template <class elemType>
struct TreeNode {
elemType data;
TreeNode<elemType> *left;
TreeNode<elemType> *right;
};
//Definition of class Binary Tree
template <class elemType>
class BinaryTree {
protected:
TreeNode<elemType> *root;
public:
BinaryTree();
BinaryTree(const BinaryTree<elemType>& otherTree);
~BinaryTree(0;
bool isEmpty() const;
virtual bool search(const elemType& searchItem) const = 0;
virtual void insert(const elemType& insertItem) = 0;
virtual void deleteNode(const elemType& deleteItem) = 0;
private
int height(TreeNode<elemType> *p) const;
int nodeCount(TreeNode<elemType> *p) const;
int leafCount(TreeNode<elemType> *p) const;
};
%3D
%3D
// BinarySearchTree.h
#include "BinaryTree.h"
using names pace std;
template <class elemType>
class BinarySearchTree: public BinaryTree<elemType> {
public:
bool search(const elemTy pe& searchItem) const;
void insert(const elemType& insertItem);
void deleteNode(const elemType& deleteItem);
private
void displayAscending(TreeNode<elemType> *p) const;
TreeNode<elemType> * getTreeMax(TreeNode<elemType> *p) const;
TreeNode<elemType> * getTreeMin(TreeNode<elemType> *p) const;
};
#
$
%
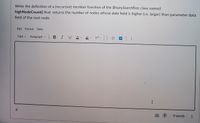
Transcribed Image Text:Write the definition of a (recursive) member function of the BinarySearchTree class named
highNodeCount() that returns the number of nodes whose data field is higher (i.e. larger) than parameter data
field of the root node.
Edit Format Table
12pt v Paragraph v
| BIU Av 2v T?v|
WP
O words
:::
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- public class HeapPriQ<T> implements PriQueueInterface<T>{ protected ArrayList<T> elements; // priority queue elements protected int lastIndex; // index of last element in priority queue protected int maxIndex; // index of last position in ArrayList protected Comparator<T> comp; public HeapPriQ(int maxSize) // Precondition: T implements Comparable { elements = new ArrayList<T>(maxSize); lastIndex = -1; maxIndex = maxSize - 1; comp = new Comparator<T>() { public int compare(T element1, T element2) { return ((Comparable)element1).compareTo(element2); } }; } public HeapPriQ(int maxSize, Comparator<T> comp) // Precondition: T implements Comparable { elements = new ArrayList<T>(maxSize); lastIndex = -1; maxIndex = maxSize - 1; this.comp = comp; } public boolean isEmpty() // Returns true if this priority queue is empty; otherwise, returns false. {…arrow_forwardPlease help with C++ question in image. Thank you.arrow_forwardplease write code both in java an pythonarrow_forward
- Tree.java: This is a simple binary tree class that should be used to represent the data in your Huffman tree. You only need to implement two of the methods (_printTree and compareTo). Huffman.java: This file contains all of the logic required to implement a Huffman tree. The code in this file relies on the Tree.java file. You must provide the implementation for all of the methods in this class. public class Main { /* * tree.txt should produce this tree: * 16 * / \ * 6 Z * / \ * A D * * A (1), D (5), Z (10) * * Codes: * A: 00 * D: 01 * Z: 1 * * Preorder Traversal: * 16, 6, 1 (A), 5 (D), 10 (Z) */ public static void main(String[] args) throws Exception { Huffman huff = new Huffman(); huff.buildTreeFromFile("tree.txt"); // Print the tree: System.out.println("printTree tests:"); huff.printTree(); // Expected output: [16: , 6: , 1:A, 5:D, 10:Z] // Get some codes:…arrow_forwardCreate a function that uses Node * pointing to root of AVL Tree as an input and returns the height of the AVL tree in O(log(n)).arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
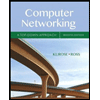
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
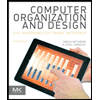
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
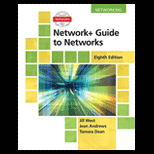
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
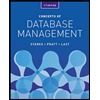
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
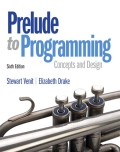
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
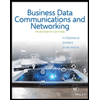
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY