Implement the provided Queue interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in a test harness. Add to your ‘StagBusClient’ the following functionality using your Queue. -Create (enqueue) 6 riders by name. Iterate over the queue, print all riders -Peek at the queue / print the result -Remove (dequeue) the head of the queue. Iterate over the queue, print all riders -Add two more riders to the queue -Peek at the queue & print the result -Remove the head & print the result. Iterate over the queue, print all riders StagBusClient.java package app; import queue.Queue; import queue.QueueImpl; public class StagBusClient { public static void main(String[] args) { // create implementation, then //QueueRunTestMethod... System.out.println("----Q U E U E T E S T-------"); //StackRunTestMethod... } } Queue.java package queue; public interface Queue { boolean isFull() ; boolean isEmpty(); // insert elements to the queue void enQueue(String element); // delete element from the queue String deQueue(); // display element of the queue void display(); //display 'first' element public String peek(); } QueueImpl.java package queue; public class QueueImpl implements Queue { } QueueTester package queue; public class QueueTester { public static void main(String[] args) { } }
Implement the provided Queue interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in a test harness. Add to your ‘StagBusClient’ the following functionality using your Queue.
-Create (enqueue) 6 riders by name. Iterate over the queue, print all riders
-Peek at the queue / print the result
-Remove (dequeue) the head of the queue. Iterate over the queue, print all riders
-Add two more riders to the queue
-Peek at the queue & print the result
-Remove the head & print the result. Iterate over the queue, print all riders
StagBusClient.java
package app;
import queue.Queue;
import queue.QueueImpl;
public class StagBusClient {
public static void main(String[] args) {
// create implementation, then
//QueueRunTestMethod...
System.out.println("----Q U E U E T E S T-------");
//StackRunTestMethod...
}
}
Queue.java
package queue;
public interface Queue {
boolean isFull() ;
boolean isEmpty();
// insert elements to the queue
void enQueue(String element);
// delete element from the queue
String deQueue();
// display element of the queue
void display();
//display 'first' element
public String peek();
}
QueueImpl.java
package queue;
public class QueueImpl implements Queue {
}
QueueTester
package queue;
public class QueueTester {
public static void main(String[] args) {
}
}

Step by step
Solved in 2 steps

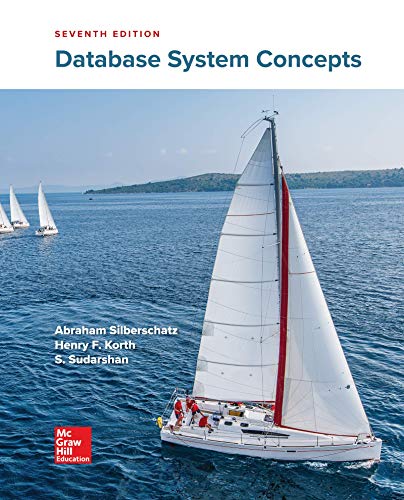
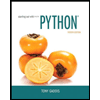
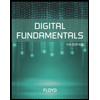
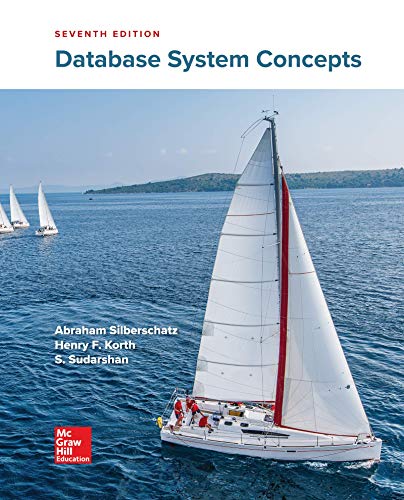
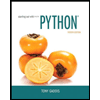
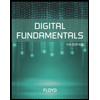
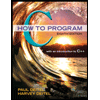
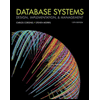
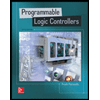