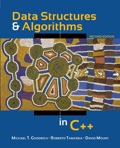
Explanation of Solution
//include the required header files
#include<iostream>
#include<cstdlib>
//use std namespace
using namespace std;
//function that takes an array
//it will generate random integers
void Random_Order(int arr[],int n)
{
//variable for counting random integers between 1 to 52
int count=0;
//while loop will iterate till count will equal to 52
while(count<n)
{
//flag to check random number is already generated or not
int flag=0;
//built in function to generate random number
int random_number=(rand()%n)+1;
//for loop for checking if random number is exist in the array or not
for(int i=0;i<count;i++)
{
//if number is exist in the array, make flag as 1
if(random_number==arr[i])
{
//set flag as 1
flag=1;
}
}
//if flag is 0, add into array
if(flag==0)
{
//add number to the array
arr[count]=random_number;
//increment the count by 1
count++;
}
}
//create a variable c
int c=0;
//print the statement
cout<<"52 Random Numbers:\n\n";
//displaying numbers
//10 integers in each row
for(int i=0;i<n;i++)
{
//print the values
cout<<arr[i]<<"\t";
//increment c by 1
c++;
//if the value of c is 10
if(c==10)
{
//print new line
cout<<"\n";
//set the value c is 0
c=0;
}
}
//print new line
cout<<"\n";
}
//main method
int main()
{
//create an integer variable n
int n=52;
//create an integer array arr
int arr[n];
//call the method Random_Order()
Random_Order(arr,n);
}
Explanation:
The above snippet of code is used print 52 random numbers using “rand()” function. In the code,
- Include the required header files.
- Use the “std” namespace.
- Define “Random_Order()” function.
- Declare the required variable “count”.
- Iterate a “while” loop.
- Declare an integer variable “flag”.
- Call built in function “rand()” to get the random number and save it to the variable “random_number”.
- Iterate a “for” loop to check the number is already exist in the array.
- If the value of “random_number” is equal to value at “arr[i]”.
- Set the value of “flag” as “1”.
- If the value of “random_number” is equal to value at “arr[i]”.
- If the value of “flag” is “0”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
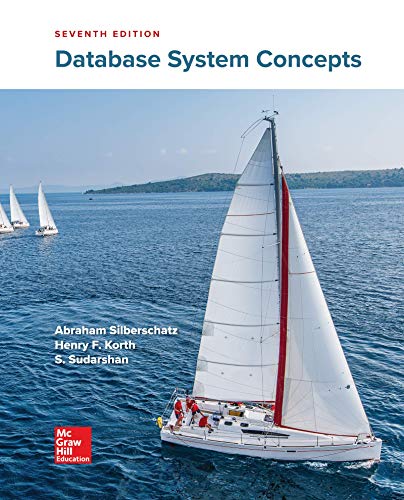
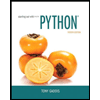
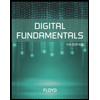
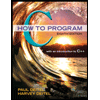
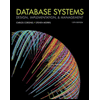
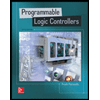