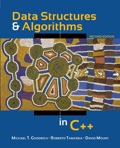
Explanation of Solution
Program code:
//include the required header files
#include <iostream>
#include <string>
#include <iomanip>
#include <fstream>
//use the std namespace
using namespace std;
//create a string array MONTHARRAY
const string MONTHARRAY[12] = { " January", " February", " March", " April", " May", " June", " July", " August", " September", " October", " November", " December"};
//create a string array DAYARRAY
const string DAYARRAY[7] = {"Sun ", " Mon ", " Tue ", " Wed ", " Thu ", " Fri ", " Sat"};
int a,y,day,m,d, x;
//create integer variable month
int month;
//define two functions printMonth() and getFirst
void printMonth(int month, int startDay, int year);
int getFirst(int month, int day, int year);
//define the main() function
int main (void)
{
//prompt the user to enter the year later than 1582
cout<<"Please enter a year later than 1582: ";
//scan for the value
cin>>x;
//if the value of x is greater than 1582
if(x>1582)
{
//iterate a for loop
for (int i = 0; i<12; i++)
{
//call the methods
getFirst(i, 1, x);
printMonth(i, d, x);
}
}
//if the value of x is less than 1582
else
{
//print the statement
cout<<"That year is before 1582, please enter a better year: ";
//scan for the value
cin>>x;
}
//return 0
return 0;
}
//define the method getFirst()
int getFirst(int month, int day, int year)
{
//set the values
a = (14-month)/12;
y = year-a;
m = month+12*a-2;
d = (day+y+y/4-y/100+y/400+(31*m/12))%7;
//return the value of d
return d;
}
//define the method printMonth()
void printMonth(int month, int startDay, int year)
{
//create integer array to hold the dates
int daysInMonths[12] = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
//create two integer variables
int copy = 1;
int counter = 1;
//print the values of M ONTHARRAY[]
cout<<MONTHARRAY[month]<<endl;
//finding leap year
//if the year is divisible by 4
if(year%4 == 0)
//set the daysInMonths[1] as 29
daysInMonths[1] = 29;
//iterate a for loop
for(int i = 0; i <=6; i++)
{
//print the values of DAYARRAY[]
cout<<DAYARRAY[i];
//if the value i is 6
if(i == 6)
//new line
cout<<endl;
}
//iterate a while loop
while(copy<=daysInMonths[month])
{
//if the value of counter is less than or equal to startDay
if(counter <= startDay)
//print the value of left and call the method setw()
cout<<left<<setw(5)<<" ";
//if the value of counter is greater than startDay
else
{
//print the value of left and call the method setw()
cout<<left <<setw(5) <<copy;
//increment the value of copy by 1
copy++;
}
//if the counter is divisible by 7
if(counter%7 == 0 )
//print a new line
cout<<endl;
//increment the counter by 1
counter++;
}
//print a new line
cout<<endl;
}
Explanation:
The above snippet of code is used create the calendar of any year later 1582. Check the leap year. In the code,
- Include the required header files.
- Use the “std” namespace.
- Create string array “MONTHARRAY[]” and “DAYARRAY[]”.
- Create the required integer variables.
- Declare two functions “printMonth()” and “getFirst()”.
- Define “main()” function.
- Prompt the user to enter the year later than 1582.
- Scan for the value.
- If the value of “x” is greater than 1582.
- Iterate a “for” loop.
- Call the method “getFirst()” and “printMonth()”.
- Iterate a “for” loop.
- If the value of “x” is less than 1582.
- Prompt the statement.
- Print the value of “x”.
- Return “0”.
- Define a method “printMonth()”.
- Declare integer array, “daysInMonth[]” and variables “copy”, “counter”.
- Print the values of the array “MONTHARRAY[]”.
- If the year is divisible by “4”.
- Iterate a “for” loop;
- Print the values of the array “DAYARRAY[]”.
- If the value of “i” is equal to “6”.
-
- Print a new line.
- Iterate a “while” loop.
- If the value of “counter” is less than or equal to “startDay”.
- Print the value of “left” and “setw(5)”.
- If the value of “counter” is greater than “startDay”.
- Print the value of “left” and “setw(5)”.
- Increment the value of “copy” by 1.
- If the value of “counter” is divisible by “7”.
- Print a new line.
- Increment the “counter” by 1
- If the value of “counter” is less than or equal to “startDay”.
- Print a new line.
- Iterate a “while” loop.
- Print a new line.
- Iterate a “for” loop;
Output:
Please enter a year later than 1582: 1992
January
Sun Mon Tue Wed Thu Fri Sat
1 2 3 4 5 6 7
8 9 10 11 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
29 30 31
February
Sun Mon Tue Wed Thu Fri Sat
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29
March
Sun Mon Tue Wed Thu Fri Sat
;&#x...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
Data structures and algorithms in C++
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
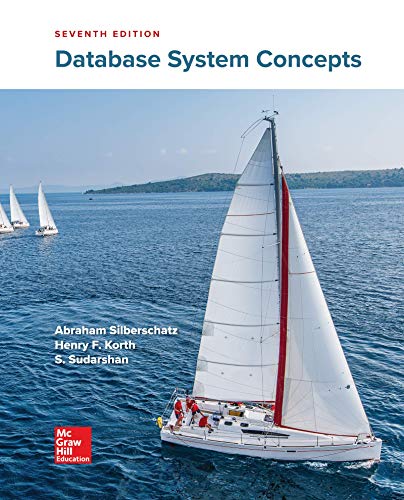
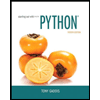
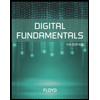
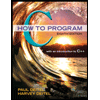
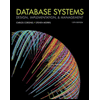
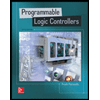