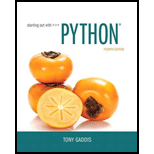
Concept explainers
Cash Register
This exercise assumes you have created the RetailItem class for
■ A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list.
■ A method named get_total: that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list.
■ A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list.
■ A method named clear that should clear the CashRegister object’s internal list.
Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the program should display a list of all the items he or she has selected for purchase, as well as the total price.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Starting Out with Python (4th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++: Early Objects
Starting Out with Programming Logic and Design (4th Edition)
Computer Science: An Overview (12th Edition)
Starting out with Visual C# (4th Edition)
- JAVA PROGRAMMING: Lesson – Overloading Constructors Create a class named House that includes data fields for the number of occupants and the annual income as well as methods named setOccupants(), setIncome(), getOccupants() and getIncome() that set and return those values respectively. Additionally, create a constructor that requires no arguments and automatically sets the occupants field to 1 and income field to 0. Create an additional overloaded constructor . This constructor receives an integer argument and assigns the value to the occupants field. Create a third overloaded constructor this time, the constructor receives 2 arguments, the values of which are assigned to the occupants and income fields respectively. Create another class named I_house that instantiates the House class and see if the constructors work correctly.arrow_forwardRetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Item #3 Shirt 20 24.95arrow_forwardEmployee and ProductionWorker Classes Create an Employee class that has properties for the following data: Employee name Employee number Next, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data: Shift number (an integer, such as 1, 2, or 3) Hourly pay rate The workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values.arrow_forward
- Circle ClassWrite a Circle class that has the following fields:• radius: a double• PI: a final double initialized with the value 3.14159The class should have the following methods:• Constructor. Accepts the radius of the circle as an argument.• Constructor. A no-arg constructor that sets the radius field to 0.0.• setRadius. A mutator method for the radius field.• getRadius. An accessor method for the radius field.• area. Returns the area of the circle, which is calculated asarea = PI * radius * radius• diameter. Returns the diameter of the circle, which is calculated asdiameter = radius * 2• circumference. Returns the circumference of the circle, which is calculated ascircumference = 2 * PI * radiusWrite a program that demonstrates the Circle class by asking the user for the circle’s radius, creating a Circle object, and then reporting the circle’s area, diameter, and circumference.arrow_forwardClass Student __init__(self, id: int, fist_name: str, last_name: str, town:str): """ This creates a student object with the specified ID first and last name and home town. This constructor should also create data structure for holding the students grades for all of there assignments. Additionally it should create a variable that holds the student's energy level which will be a number between 0 and 1. :param id: The student's identifiaction number :param fist_name: The student's first name :param last_name: The student's last name :param town: The student's home town """ get_id(self)->int: """ Returns the ID of the student as specified in the constructor. :return: The student's ID """ get_first_name(self) -> str: """ Returns the first name of the student. :return: The student's first name """ set_first_name(self, name:str): """ Changes the student first name to the specified value of the name parameter. :param name: The value that the first name of the student will equal. """…arrow_forwardQuestion Create a class called Quadratic for performing arithmetic on and solving quadratic equations. A quadratic equation is an equation of the form ax2 + bx + c = 0 Where a !=0. Use double variables to represent the values of a, b, and c and provide a constructor that enables objects of this class to be initialized when they are created. Give default values of a = 1, b = 0, and c = 0. Create a char variable called variable to represent the variable used in the equation and give it a default value of x. The constructor should not allow the value of a to be 0. If 0 is given, assign 1 to a). Provide public member functions that perform the following tasks. add—adds two Quadratic equations by adding the corresponding values of a, b, and c. The function takes another object of type Quadratic as its parameter and adds it to the calling object. subtract—subtracts two Quadratic equations by subtracting corresponding values of a, b, and…arrow_forward
- C# (Odd or Even) Write an app that reads an integer, then determines and displays whether it’s odd or even. (Hints: use the remainder operator) (Multiples) Write an app that reads two integers, determines whether the first is a multiple of the second, and displays the result. (Hints: use the remainder operator). Create a class called Date that includes three pieces of information as auto-implemented properties: a month (type int), a day (type int), and a year (type int). Your class should have a constructor that initializes the three fields. Provide a method DisplayDate() that displays the three fields (separated by '/'). Write a test app named DateDemo that demonstrates class Date’s capabilities.arrow_forwardCircle Class (Easy) Write a Circle class that has the following member variables: radius : a double The class should have the following member functions: Default Constructor: default constructor that sets radius to 0.0. Constructor: accepts the radius of the circle as an argument. setRadius: an mutator function for the radius variable. getRadius: an accessor function for the radius variable. getArea: returns the area of the circle, which is calculated as area = pi * radius * radius getCircumference: returns the circumference of the circle, which is calculated as circumference = 2 * pi * radius Step1: Create a declaration of the class. Step2: Write a program that demonstrates the Circle class by asking the user for the circle’s radius, creating Circle objects, and then reporting the circle’s area, and circumference. You should create at least two circle objects, one sets the radius to 0.0 and one accepts the radius as an…arrow_forwardProblem: Employee and ProductionWorker Classes Write a python class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3)• Hourly pay rateThe workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for this class. Once you have written the class, write a program that creates an object of the ProductionWorker class, and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen Note: The program should be written in python. Sample Input/Output: Enter the name: Ahmed Al-AliEnter the ID number: 12345Enter the department:…arrow_forward
- inventory.py templete #========The beginning of the class ========== classShoe: def__init__(self,country,code,product,cost,quantity): pass ''' In this function, you must initialise the following attributes: ● country, ● code, ● product, ● cost, and ● quantity. ''' defget_cost(self): pass ''' Add the code to return the cost of the shoe in this method. '''defget_quantity(self):pass''' Add the code to return the quantity of the shoes. ''' def__str__(self): pass ''' Add a code to returns a string representation of a class. ''' #=============Shoe list==========='''The list will be used to store a list of objects of shoes.''' shoe_list=[] #==========Functions outside the class============== defread_shoes_data(): pass ''' This function will open the file inventory.txt and read the data from this file, then create a shoes object with this data and append this object into the shoes list. One line in this file represents data to create one object of shoes. You must use the try-except in this…arrow_forwardT/F A method defined in a class can access the class' instance data without needing to pass them as parameters or declare them as local variables.arrow_forward(Invoice Class) Create a class called Invoice that a hardware store might use to representan invoice for an item sold at the store. An Invoice should include four data members—a part number (type string), a part description (type string), a quantity of the item being purchased (typeint) and a price per item (type int). Your class should have a constructor that initializes the fourdata members. A constructor that receives multiple arguments is defined with the form:ClassName( TypeName1 parameterName1, TypeName2 parameterName2, ... )Provide a set and a get function for each data member. In addition, provide a member functionnamed getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by theprice per item), then returns the amount as an int value. If the quantity is not positive, it should beset to 0. If the price per item is not positive, it should be set to 0. Write a test program that demonstrates class Invoice’s capabilitiesarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
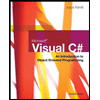
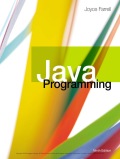