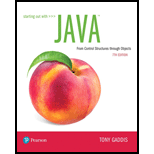
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11, Problem 4PC
Program Plan Intro
Month class exception
Program Plan:
Month.java:
- Definition of class “Month”.
- Declare the variable “monNum” as “int”.
- Create a constructor for “Month()”.
- Assign “monNum” to “1”.
- Create a parameterized constructor which throws the “InvalidMonthNumExc”.
- Check the condition.
- Throw the exception.
- Otherwise, assign “m” to “monNum”.
- Check the condition.
- Create a parameterized constructor which throws the “InvalidMonthNumExc”.
- Check the condition.
- Assign “1” to “monNum”.
- Condition to check the month is “february”.
- Assign “2” to “monNum”.
- Condition to check the month is “march”
- Assign “3” to “monNum”.
- Condition to check the month is “april”.
- Assign “4” to “monNum”.
- Condition to check the month is “may”
- Assign “5” to “monNum”.
- Condition to check the month is “june”.
- Assign “6” to “monNum”.
- Condition to check the month is “july”
- Assign “7” to “monNum”.
- Condition to check the month is “august”
- Assign “8” to “monNum”.
- Condition to check the month is “September”.
- Assign “9” to “monNum”.
- Condition to check the month is :”October”.
- Assign “10” to “monNum”.
- Condition to check the month is “November”.
- Assign “11” to “monNum”.
- Condition to check the month is “December”.
- Assign “12” to “monNum”.
- Check the condition.
- Mutator method “setMonNum()”
- Check the condition and throw the exception
- Accessor method “getMonNum()”.
- Return the “monNum”.
- Accessor method “getMonName()”.
- Declare the variable “name”.
- Switch case to check the month equals to “January”, “February”, “March”, “April”, “May”, “June”, “July”, “August”, “September”, “October”, “November”, “December”.
- Return the name.
- Declare the variable “name”.
- Method definition for “toString()”.
- Return the “getMonName()”.
- Method definition of “equals()”
- Declare the boolean variable “flag”.
- Check the condition and assign the flag variable “true” or “false” according to the condition.
InvalidMonthNumExc.java:
- Definition for “InvalidMonthNumExc”
- Definition for non-parameterized constructor.
- Print the statement.
- Definition for parameterized constructor
- Print the statement.
- Definition for non-parameterized constructor.
InvalidMonthNameExc.java:
- Definition for “InvalidMonthNameExc”
- Definition for non-parameterized constructor.
- Print the statement.
- Definition for parameterized constructor
- Print the statement.
- Definition for non-parameterized constructor.
DemoOne.java:
- Definition of class “DemoOne”.
- Definition of main class.
- Create an object “m” for “month()”.
- Inside the try block,
- Print the month
- Inside the catch block,
- Print the exception.
- Inside the try block,
- Create an object “m” for “month()”.
DemoTwo.java:
- Definition of class “DemoTwo”.
- Definition of main class.
- Inside the try block,
- Create an object “m” for “month()”.
- Print the month
- Inside the catch block,
- Print the exception.
- Inside the try block,
DemoThree.java:
- Definition of class “DemoThree”.
- Definition of main class.
- Inside the try block,
- Create an object “m” for “month()”.
- Print the month
- Inside the catch block,
- Print the exception.
- Inside the try block,
- Create an object “m1” for “month()”.
- Print the month
- Inside the catch block,
- Print the exception.
- Inside the try block,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
15 OF 25 QUESTIONS REMAININ
Consider the following code. You want to print the array values in the div as an ordered list. What statement would you use to
replace the comment in the code below?
Two
J
// what statement goes here?
-
لبية للالكالا
const app = Vue.createApp({
data ((
return (
lunch: [
'Burrito',
'Soup',
'Pizza',
'Rice'
})
app.mount ('#app6')
-
-
Please answer JAVA OOP problem below:
Assume you have three data definition classes, Person, Student and Faculty. The Student and Faculty classes extend Person. Given the code snippet below, in Java, complete the method determinePersonTypeCount to print out how many Student and Faculty objects exist within the Person array. You may assume that each object within the Person[] is either referencing a Student or Faculty object.
public static void determinePersonTypeCount(Person[] people){
// Place your code here
}
Please answer JAVA OOP question below:
Consider the following relationship diagram between the Game and VideoGame data defintion classes.
Game has a constructor that takes in two parameters, title (String) and cost (double). The VideoGame constructor has an additional parameter, genre (String). In Java, efficiently write the constructors needed within the Game class and VideoGame classes.
Hint: Remember to think about the appropriate validation
Chapter 11 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 11.1 - Prob. 11.1CPCh. 11.1 - Prob. 11.2CPCh. 11.1 - Prob. 11.3CPCh. 11.1 - Prob. 11.4CPCh. 11.1 - Prob. 11.5CPCh. 11.1 - Prob. 11.6CPCh. 11.1 - Prob. 11.7CPCh. 11.1 - Prob. 11.8CPCh. 11.1 - Prob. 11.9CPCh. 11.1 - When does the code in a finally block execute?
Ch. 11.1 - What is the call stack? What is a stack trace?Ch. 11.1 - Prob. 11.12CPCh. 11.1 - Prob. 11.13CPCh. 11.1 - Prob. 11.14CPCh. 11.2 - What does the throw statement do?Ch. 11.2 - Prob. 11.16CPCh. 11.2 - Prob. 11.17CPCh. 11.2 - Prob. 11.18CPCh. 11.2 - Prob. 11.19CPCh. 11.3 - What is the difference between a text file and a...Ch. 11.3 - What classes do you use to write output to a...Ch. 11.3 - Prob. 11.22CPCh. 11.3 - What class do you use to work with random access...Ch. 11.3 - What are the two modes that a random access file...Ch. 11.3 - Prob. 11.25CPCh. 11 - Prob. 1MCCh. 11 - Prob. 2MCCh. 11 - Prob. 3MCCh. 11 - Prob. 4MCCh. 11 - FileNotFoundException inherits from __________. a....Ch. 11 - Prob. 6MCCh. 11 - Prob. 7MCCh. 11 - Prob. 8MCCh. 11 - Prob. 9MCCh. 11 - Prob. 10MCCh. 11 - Prob. 11MCCh. 11 - Prob. 12MCCh. 11 - Prob. 13MCCh. 11 - Prob. 14MCCh. 11 - Prob. 15MCCh. 11 - This is the process of converting an object to a...Ch. 11 - Prob. 17TFCh. 11 - Prob. 18TFCh. 11 - Prob. 19TFCh. 11 - True or False: You cannot have more than one catch...Ch. 11 - Prob. 21TFCh. 11 - Prob. 22TFCh. 11 - Prob. 23TFCh. 11 - Prob. 24TFCh. 11 - Find the error in each of the following code...Ch. 11 - // Assume inputFile references a Scanner object,...Ch. 11 - Prob. 3FTECh. 11 - Prob. 1AWCh. 11 - Prob. 2AWCh. 11 - Prob. 3AWCh. 11 - Prob. 4AWCh. 11 - Prob. 5AWCh. 11 - Prob. 6AWCh. 11 - The method getValueFromFile is public and returns...Ch. 11 - Prob. 8AWCh. 11 - Write a statement that creates an object that can...Ch. 11 - Write a statement that opens the file...Ch. 11 - Assume that the reference variable r refers to a...Ch. 11 - Prob. 1SACh. 11 - Prob. 2SACh. 11 - Prob. 3SACh. 11 - Prob. 4SACh. 11 - Prob. 5SACh. 11 - Prob. 6SACh. 11 - What types of objects can be thrown?Ch. 11 - Prob. 8SACh. 11 - Prob. 9SACh. 11 - Prob. 10SACh. 11 - What is the difference between a text file and a...Ch. 11 - What is the difference between a sequential access...Ch. 11 - What happens when you serialize an object? What...Ch. 11 - TestScores Class Write a class named TestScores....Ch. 11 - Prob. 2PCCh. 11 - Prob. 3PCCh. 11 - Prob. 4PCCh. 11 - Prob. 5PCCh. 11 - FileArray Class Design a class that has a static...Ch. 11 - File Encryption Filter File encryption is the...Ch. 11 - File Decryption Filter Write a program that...Ch. 11 - TestScores Modification for Serialization Modify...Ch. 11 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In a shopping cart, there are various items, which can either belong to the category of household items or electronic items. The following UML diagram illustrates the relationship between items, household items, and electronic items. //Implementation Class public class ShoppingCart{ public static void main(String[] args){ final int MAX_ITEM = 50; Item cart = new Item[MAX_ITEM]; addItem(cart); // populate the item array printItem(cart); } } Considering that all the data definition classes and the implementation class are complete, which of the following Object-Oriented Programming (OOP) concepts do you need to use in the above context? i) Polymorphism ii) Method Overloading iii) Method Overriding iv) Dynamic Binding v) Abstract Class Explain, using course terminology, how you would use any of the above concepts to model the given scenario.arrow_forwardAnswer this JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank public class TestImplementation{ public static void main(String[] args){ Employee[] allEmployee = new Employee[100]; // create an employee object with name Tom Evan, employee ID 001 and department IST and store it in allEmployee // create a faculty object with name Adam Scott, employee ID 002, department IST and rank Professor and store it in allEmployee } }arrow_forwardPlease answer this JAVA OOP question that is given below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: A toString() method that includes both the inherited attributes and the specializationarrow_forward
- Please answer JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: Instance variable(s) A Constructorarrow_forwardDevelop a C++ program that execute the operation as stated by TM for addition of two binary numbers (see attached image). Your code should receive two binary numbers and output the resulting sum (also in binary). Make sure your code mimics the TM operations (dealing with the binary numbers as a string of characters 1 and 0, and following the logic to increase the first number and decreasing the second one. Try your TM for the following examples: 1101 and 101, resulting 10010; and 1101 and 11, resulting 10000.arrow_forwardI need to define and discuss the uses of one monitoring or troubleshooting tool in Windows Server 2019. thank youarrow_forward
- I would likr toget help with the following concepts: - Windows Server features - Windows Server versus Windows 10 used as a client-server networkarrow_forwardI need to define and discuss the uses of one monitoring or troubleshooting tool in Windows Server 2019. thank youarrow_forwardWhy is planning for the retirement system and transition critical?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
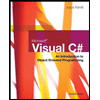
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
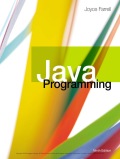
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
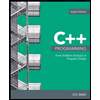
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
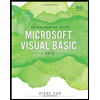
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
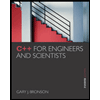
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr