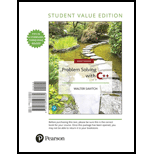
Concept explainers
Explanation of Solution
Program:
//Header file
#include <iostream>
//For standard input and output
using namespace std;
//Create namespace "sally"
namespace sally
{
//Function declaration for message()
void message( );
}
//Create unnamed namespace
namespace
{
//Function declaration for message()
void message( );
}
//Main function
int main( )
{
{
/* Call the message() function for unnamed namespace */
message( );
/* Call the function message() by "using" directive */
using sally::message;
//Call message() function
message( );
}
/* Call the message() function for unnamed namespace */
message( );
return 0;
}
/* Create function definition of namespace for "message()" function */
namespace sally
{
/* Function definition for message() */
void message( )
{
/* Display message */
cout << "Hello from Sally.\n";
}
}
/* Create function definition of unnamed namespace for "message()" function */
namespace
{
void message( )
{
/* Display message */
cout << "Hello from unnamed.\n";
}
}
Explanation:
The given code is used to display the message from “sally” namespace and unnamed namespace...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Problem Solving with C++, Student Value Edition
- THIS IS IN JAVA A programmer intended to compute (a AND b) OR c, and wrote the following code, which sometimes yields incorrect output. Why? <pre><code>c | (a & b)</code></pre> A. The parentheses are interfering, and should not be present B. The ORing with c should appear after the parentheses, not before C. The bitwise operators should be replaced by logical operators D. If code only sometimes yields incorrect output, the compiler is likely brokenarrow_forwardConsider the following program in C-like syntax:void f1();void f2();int main(){int x, y;...}int f1()int a, b, x;...}int f2(){int b, c, x, y;...} If the programming language uses dynamic scoping, what are the variables that arevisible in the last function in the following call sequences? Give not only the names ofthe variables, but also where they are declared.(a) main → f1 → f2(b) main → f2 → f1arrow_forwardPROBLEM: I need to make an ATM program that allows the user to check his or her balance after entering his or her correct PIN and the maintaining balance is Php 8000. A message is shown when the balance is below PHP 8,000 for withdrawal and its remaining balance. Another message is shown when the balance is PHP 8,000 and above for deposit and its updated balance. The code: import random import sys class ATM(): def __init__(self, name, account_number, balance = 0): self.name = name self.account_number = account_number self.balance = balance def account_detail(self): print("\n----------ACCOUNT DETAIL----------") print(f"Account Holder: {self.name.upper()}") print(f"Account Number: {self.account_number}") print(f"Available balance: Nu.{self.balance}\n") def deposit(self, amount): self.amount = amount self.balance = self.balance + self.amount print("Current account…arrow_forward
- I am having issues with C++ because this code does not work: int main() {int userNum; userNum = 5;cout << "Foretelling is hard." << endl;cout << "Particularly of the future." << endl;cout << "User num is:" << userNum << endl;/* Your solution goes here */ return 0; Please tell me what to do with this line of code.arrow_forwardCAN YOU CONVERT FROM C TO C++? #include <stdio.h>#include <stdlib.h>#include <time.h> int r_pc[3] = {0, 0, 0};int w_pc[3] = {0, 0}; int lock = 0;int rlock = 0;int wlock = 0; int AR = 0; int critical_readers = 0;int critical_writers = 0; int panic = 0; int testandset(int *lock){if (*lock == 1)return 1; else{*lock = 1; return 0; }}arrow_forward#include <iostream> using namespace std; void additionProblem(int topNumber, int bottomNumber) { int userAnswer; cout << "\n\n\n " << topNumber << " + " << bottomNumber << " = "; cin >> userAnswer; cin.ignore(1000, 10); int theAnswer = topNumber + bottomNumber; if (theAnswer == userAnswer) cout << " Correct!" << endl; else cout << " Very good, but a better answer is " << theAnswer << endl; } // additionProblem int main() { additionProblem(8, 2); additionProblem(4, 8); additionProblem(3, 7); additionProblem(4, 10); additionProblem(11, 2); } // main The above code needs to be modified according to the image I've attatched.arrow_forward
- In C++ 2.12 LAB: Hypotenuse Given two numbers that represent the lengths of a right triangle's legs (sides adjacent to the right angle), output the length of the third side (i.e. hypotenuse) with two digits after the decimal point. Output each floating-point value with two digits after the decimal point, which can be achieved by executingcout << fixed << setprecision(2); once before all other cout statements. Ex: If the input is: 3.0 4.0 the output is: Hypotenuse: 5.00arrow_forwardI need complete explanation of c++ code line by line. Need Explanation of all lines of code what is happening in this code where the data is stored.? Code #include <iostream>#include <iomanip>#include <time.h>#include <fstream>#include <conio.h>#include <string.h>#include <stdlib.h>using namespace std;class Bank {public:};class ATMAccountHolders:public Bank{string accountHolders;string accountHoldersAddress, branch;int accountNumber;double startBalance;double accountBalance; double amount;int count;public:void deposit();void withdraw();void accountExit();ATMAccountHolders(){accountNumber = 7787;accountHolders = " Ammad Naseer";accountHoldersAddress = " House no.112";startBalance = 6000.00;accountBalance = 6000.00;branch = " Islamabad";amount = 20000; }}; void ATMAccountHolders::deposit(){system("cls"); cout<<" ATM ACCOUNT DEPOSIT SYSTEM ";cout<<"\n\nThe Names of the Account Holders are…arrow_forward2. Consider the following program. #include <iostream> using namespace std; void summer(int&, int); void fall(int, int&); int x; int main() { int intNum1 = 2; int intNum2 = 5; x = 6; summer(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; fall(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; return 0; } void summer(int& a, int b) { int intNum1; intNum1 = b + 12; a = 2 * b + 5; b = intNum1 + 4; } void fall(int u, int& v) { int intNum2; intNum2 = x; v = intNum2 * 4; x = u - v; } Answer the following questions: a. What is the output? Consider variable scope. b. Considering the function summer, parameter 1 is called by reference. What is passed into the function for parameter 1, i.e., what value does parameter 1 receive? c. Again, considering the function summer, parameter 2 is called by value. What occurs in memory for parameter 2 and local…arrow_forward
- What is the output of the following C++ code? #include <iostream> using namespace std; class class0 { public: virtual ~class0(){} protected: char p; public: char getChar(); }; class class1 : public class0 { public: void printChar(); }; void class1::printChar() { cout << "True" << endl; } int main() { class1 c; c.printChar(); return 1; }arrow_forwardMy question: I don't understand why the last output has two letters inside. Can you please fix my code? Write a function `mostFrequentLetter(string)` that takes a string as an argument and returns the character that appears the most often. In case of a tie, you may return any of the characters. The string will have at least one character. Examples: console.log(mostFrequentLetter("apple")); // "p" console.log(mostFrequentLetter("banana")); // "a" console.log(mostFrequentLetter("What about a longer string?")); // " " My code: function countChar(string) { letcountChar = {}; for (leti = 0; i < string.length; i++) { letchar = string[i];//the future key elements if (charincountChar){//create keys countChar[char]++;//create values }else { countChar[char] = 1;//create values } } returncountChar; } function mostFrequentLetter(string) { letmaxCount = countChar(string); letnewArr = Object.entries(maxCount); letmax = newArr[0][1]; letresult = []; for (leti = 0; i < newArr.length;…arrow_forwardFOR C++, PLEASE SEND THE CODE IN 20 MINUTES!!! ANSWER THIS QUESTION IN THE GIVEN CODE Write a function that check whether there is enough material in the container for building the given recipe. #include <iostream>#include <string>#include <vector> using namespace std; class Material { private: string name; int amount; float quality; public: Material() { } Material(string name, int amount, float quality) { this->name = name; this->amount = amount; this->quality = quality; } string getName() { return this->name; } int getAmount() { return this->amount; } float getQuality() { return this->quality; } void print() { cout << "Name: " << this->name << " Amount: " << this->amount << " Quality: " << this->quality << endl; }}; bool isInGoodQuality(Material*, int);bool canBeBuilt(Material*, Material*,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
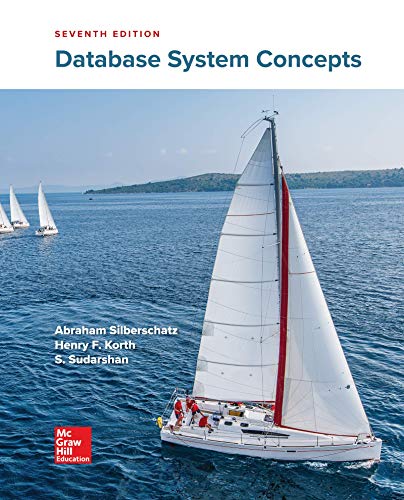
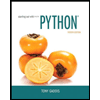
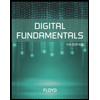
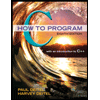
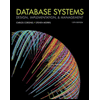
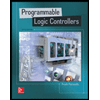