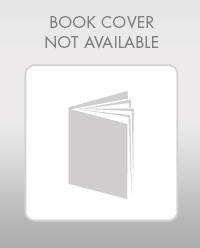
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 14.1, Problem 14.3CP
Explanation of Solution
Purpose of the code:
The program is to print the value of “arg” using recursive function.
Program:
//Include required header files
#include <iostream>//Line 1
using namespace std;//Line 2
//Function prototype
void showMe(int arg);//Line 3
//Main function
int main( )//Line 4
{//Line 5
//Declare a variable
int num = 0;//Line 6
//Call the function
showMe(num);//Line 7
//Return the statement
return 0;//Line 8
}//Line 9
//Function definition
void showMe(int arg)//Line 10
{//Line 11
//Check if the value of "arg" is less than 10
if(arg<10)//Line 12
//Call the function recursively
showMe(++arg);//Line 13
//else
else//Line 14
//Print the value of arg
&...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
FOR C++, PLASE SEND THE ANSER IN 30 MINUTES.
ACCORDING TO CODE BELOW,
#include "Vehicle.hh"
class Ship: public Vehicle {
private:
int passenger;
public:
Ship();
Ship(int, float, float);
int getPassenger();
void setPassenger(int);
void print();
};
(DEFINE FUNCTIONS CORRESPONDING TO SHIP HEADER CLASS IN PART 2 BELOW)
#include "Vehicle.hh"
#include "Ship.hh"
#include <iostream>
using namespace std;
// *** DEFINE --VEHICLE-- FUNCTIONS FOR PART 1 ***
// *** DEFINE --SHIP-- FUNCTIONS FOR PART 2 ***
void reduceSpeed(Ship*, float);
void takePassenger(Ship*, int);
int main() {
return 0;
}
// reduce the speed of ship given in percentage (0 < percentage < 1).
void reduceSpeed(Ship *s, float percentage) {
// *** FILL THIS FUNCTION FOR PART 3 ***
}
// takes the number of passengers to the ship
void takePassenger(Ship *s, int pas) {
// *** FILL THIS FUNCTION FOR PART 4 ***
}
programming paradigms
Please write the following functions in Haskell. Please also write type declarations for each function, including type classes, wherever necessary.
1. Modify isGodzilla from today's lecture to isNum where the function accepts an integer as an input parameter and outputs True if this integer matches the number in the function.
2. Write a function fibList that uses the fib function from today's lecture. fibList accepts a number n as parameter and outputs a list of the numbers in the Fibonacci series up to n. Note that fibList will use a list comprehension involving fib.
3. Write a function justHighScores that takes the list generated as output of the gradeBook function and returns a list of students who score >= 95. Please use the isHighScorer function from today's lecture in justHighScores. You will also have to implement the gradeBook function discussed in class today.
4. Modify the function lastElement from today's lecture to getSecondLast. The function…
Write a statement that calls the recursive function BackwardsAlphabet() with parameter startingLetter.
#include <iostream>using namespace std;
void BackwardsAlphabet(char currLetter){if (currLetter == 'a') {cout << currLetter << endl;}else{cout << currLetter << " ";BackwardsAlphabet(currLetter - 1);}}
int main() {char startingLetter;
startingLetter = 'z';
/* Your solution goes here */
return 0;}
Please help me with this problem using c++.
Chapter 14 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 14.1 - What is a recursive functions base case?Ch. 14.1 - What happens if a recursive function does not...Ch. 14.1 - Prob. 14.3CPCh. 14.1 - What is the difference between direct and indirect...Ch. 14 - What type of recursive function do you think would...Ch. 14 - Which repetition approach is less efficient; a...Ch. 14 - When should you choose a recursive algorithm over...Ch. 14 - Prob. 4RQECh. 14 - Prob. 5RQECh. 14 - Prob. 6RQE
Ch. 14 - Predict the Output 7. What is the output of the...Ch. 14 - Soft Skills 8. Programming is communication; the...Ch. 14 - Prob. 1PCCh. 14 - Recursive Conversion Convert the following...Ch. 14 - Prob. 3PCCh. 14 - Recursive Array Sum Write a function that accepts...Ch. 14 - Prob. 5PCCh. 14 - Recursive Member Test Write a recursive Boolean...Ch. 14 - Prob. 7PCCh. 14 - Prob. 8PCCh. 14 - Ackermanns Function Ackermanns function is a...Ch. 14 - Prefix to Postfix Write a program that reads...Ch. 14 - Prob. 11PCCh. 14 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In C++ Write the definition of a void function that has two parameters: an array, and an integer parameter that specifies the number of elements in the array. The functions swaps the first and last elements of the array.arrow_forwardFunctions With Parameters and No Return Values Quiz by CodeChum Admin Create a program that accepts an integer N, and pass it to the function generatePattern. generatePattern() function which has the following description: Return type - void Parameter - integer n This function prints a right triangular pattern of letter 'T' based on the value of n. The top of the triangle starts with 1 and increments by one down on the next line until the integer n. For each row of in printing the right triangle, print "T" for n times. In the main function, call the generatePattern() function. Input 1. One line containing an integer Output Enter·N:·4 T TT TTT TTTTarrow_forwardCreate a multiplication operator function for the Complex class in Program 11.8 that multiplies two complex numbers. Use the relationship that (a + bi) × (c + di) =(ab − bd) + (ad + bc)i.b. Include the function constructed for Exercise 4a in a complete C++ program. #include <iostream> #include <iomanip> using namespace std; //declaration section class Complex { private: double realpart; double imaginarypart; public: Complex(double real = 0.0, double imag = 0.0) { realpart = real; imaginarypart = imag;} void showcomplexvalues(); //accessor prototype void assignnewvalues(double real, double imag) //inline mutator { realpart = real; imaginarypart = imag;} }; //End of the class declaration //Implementation section void Complex::showcomplexvalues() //Accessor { char sign = '+'; if (imaginarypart < 0) sign = '-'; cout << realpart << ' ' << sign…arrow_forward
- Implement in C Programming 6.10.1: Function pass by pointer: Transforming coordinates. Define a function CoordTransform() that transforms its first two input parameters xVal and yVal into two output parameters xValNew and yValNew. The function returns void. The transformation is new = (old + 1) * 2. Ex: If xVal = 3 and yVal = 4, then xValNew is 8 and yValNew is 10. #include <stdio.h> /* Your solution goes here */ int main(void) { int xValNew; int yValNew; int xValUser; int yValUser; scanf("%d", &xValUser); scanf("%d", &yValUser); CoordTransform(xValUser, yValUser, &xValNew, &yValNew); printf("(%d, %d) becomes (%d, %d)\n", xValUser, yValUser, xValNew, yValNew); return 0;}arrow_forwardImplement in C Programming 7.11.1: LAB: Student struct Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters. Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as listed below: Student InitStudent() - initializes name to "Louie" and gpa to 1.0 Student SetName(char *name, Student s) - sets the student's name Student SetGPA(double gpa, Student s) - sets the student's GPA void GetName(char* studentName, Student s) - return the student's name in studentName double GetGPA(Student s) - returns the students GPA Ex. If a new Student object is created, the default output is: Louie/1.0 Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes: Felix/3.7 main.c #include <stdio.h> #include <string.h>…arrow_forwardIn a function aFunction, we have 2 values: num1 (float type), num2 (int type) declared locally and assigned to 2.1 and 6. Write code segment to return the two values from function aFunction.arrow_forward
- Set-up and implementation code for a void function MaxYou are not required to write a complete C++ program but must write and submit just your responses to the four specific function related questions below: QC1: Write the heading for a void function called Max that has three intparameters: num1, num2 and greatest. The first two parameters receive data from the caller, and greatest is used to return a value as a reference parameter. Document the data flow of the parameters with appropriate comments*. QC2: Write the function prototype for the function in QC1. QC3: Write the function definition of the function in QC1 so that it returns the greatest of the two input parameters via greatest, a reference parameter. QC4: Add comments to the function definition* you wrote in QC3 that also states its precondition and postcondition.arrow_forwardIn C language. Please don't copy similar programs from, bartleby or chegg Define a structure type auto_t to represent an automobile. Include components for the make and model (strings), the odometer reading, the manufacture and purchase dates (use another user-defined type called date_t), and the gas tank (use a user-defined type tank_t with components for tank capacity and current fuel level, giving both in gallons). Write I/O functions scan_date, scan_tank, scan_auto, print_date, print_tank, and print_auto, and also write a driver function that repeatedly fills and displays an auto structure variable until input is -30. Note: Each record has a number (1, 2, 3 ...),once the program scans record number -30, it should terminate without processing it. SAMPLE RUN #4: ./Structures Interactive Session Show Invisibles Highlight: Enter record number:1 Enter Make:Mercury Enter Model:Sable Enter Odometer Reading:99842 Enter Month:1 Enter Day:18 Enter Year:2001 Enter Month:5 Enter Day:30…arrow_forwardImplement in C Programming 7.8.1: LAB: Calculator Given main(), create the Calculator struct that emulates basic functions of a calculator: add, subtract, multiple, divide, and clear. The struct has one data member called value for the calculator's current value. Implement the Calculator struct and related function declarations in Calculator.h, and implement the related function definitions in Calculator.c as listed below: Calculator InitCalculator() - initialize the data member to 0.0 Calculator Add(double val, Calculator c) - add the parameter to the data member Calculator Subtract(double val, Calculator c) - subtract the parameter from the data member Calculator Multiply(double val, Calculator c) - multiply the data member by the parameter Calculator Divide(double val, Calculator c) - divide the data member by the parameter Calculator Clear(Calculator c) - set the data member to 0.0 double GetValue(Calculator c) - return the data member Given two double input values num1 and…arrow_forward
- Write a program in C that includes the following functions: A function elementsSummation() that takes an integer array and the array size as arguments and prints the summation of the array’s elements. A function smallestElement() that takes the array and its size as arguments and returns the smallest element of the array. A recursive function integerReverse() that takes the array, start index, and the array size as arguments and prints the array back to front.arrow_forwardPlease answer !!!!! Write a program in c that includes the following functions and demonstrates their use: A function elementsSummation() that takes an integer array and the array size as arguments and prints the summation of the array’s elements. A function smallestElement() that takes the array and its size as arguments and returns the smallest element of the array. A recursive function integerReverse() that takes the array, start index, and the array size as arguments and prints the array back to front.arrow_forwardC programing (condition using pointers) Q1) Write a function called letter grade that has a type int input parameter called points andreturns through an output parameters gradepLetter and gradepNumber. The appropriate lettergrade matching is given in the table below. Return through a second output parameter(just_missedp) an indication of whether the student just missed the next higher grade (true for89, 79, 64 and so on).Prototype: void letter_grade(int points, char *gradepLetter, char*gradepNumber, char *just_missedp);arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
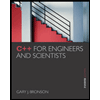
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr