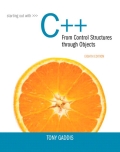
Starting Out with C++
8th Edition
ISBN: 9780133888201
Author: GADDIS
Publisher: PEARSON CUSTOM PUB.(CONSIGNMENT)
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 15PC
Program Plan Intro
Pop( ) and push( ) Member functions
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare a variable named “size” in type of integer and initialize the variable as “0” in the constructor.
- Define the constructor, destructor, and member functions in the class.
- Declare the functions prototypes of “pop_back()”, “pop_front()”, “push_back()”, and “push_front()”.
“IntList.cpp”:
- Include the required header files into the program.
- Define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the “newNode” value into received variable “num” and assign the “newNode” address into null.
- Using “if…else” condition check whether the list is empty or not, if the “head” is empty then make a new node into “head” pointer. Otherwise, make a loop to find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Increment the value of the variable “size” by “1”.
- Define a function named “display()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Define a function named “insertNode()” to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Use “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Increment the value of the variable “size” by “1”.
- Define a function named “deleteNode()” to delete a value from the list.
- Declare the structure pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Use “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Decrement the value of the variable “size” by “1”.
- Define the function named “getSize()” to return the current value of the “size”.
- Define the destructor to destroy the list values from the memory.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the “head” value into the “dataPtr”.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
- Define the function named “pop_back()” to insert a value at end of the list.
- Declare the pointer variables for structure named “ListNode” and assign null to the “previousNode”.
- Using “if” condition check whether the list is empty or not.
- If the list is empty then throw a message on the screen.
- Using “while” loop to traverse the list.
- Initialize the variable “previousNode” with the value of the variable “node”.
- Assign null to the address of “previousNode”.
- Delete the value of “node” using “delete” operator.
- Return the value of “temp” to the calling function.
- Define the function named “pop_front()” to insert a value at front of the list.
- Declare the pointer variable “node” for a structure “ListNode”.
- Using “if” condition check whether the list is empty or not.
- If the list is empty then throw a message on the screen.
- Using “while” loop to traverse the list.
- Initialize the variable “temp” with the value of the variable “head”.
- Assign null to the address of “head”.
- Delete the value of “node” using “delete” operator.
- Return the value of “temp” to the calling function.
- Define the function named “push_back()” to insert the value at end of the list.
- Make a call to the “appendNode()” function to insert the received value of variable “value” at end of the list.
- Define the function named “push_front()” to insert the value at front of the list.
- Declare a pointer variable “newNode” for the structure.
- Initialize the variable “newNode” with the value of received variable “value”.
- Using “if…else” condition check the list is empty.
- If the list is empty then add a value into “head” pointer.
- Otherwise, add a value into address of “newNode”.
- Increment the “size” variable by “1”.
“main.cpp”:
- Include the required header files into the program.
- Declare an object named “obj” for the class “IntList”.
- Make a call to functions for append and display operations.
- Make a call to function “pop_back()”, “pop_front()”, “push_front()”, and “push_back()” and display the values using a function “display()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Question:
struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question:
struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.
Write a struct ‘Student’ that has member variables: (string) first name, (int) age and (double) fee. Create a sorted (in ascending order according to the age) linked list of three instances of the struct Student. The age of the students must be 21, 24 and 27. Write a c++ function to insert the new instances (elements of the linked list), and insert three instance whose member variable have age (i) 20, (ii) 23 and (iii) 29. Write a c++ function to remove the instances from the linked list, and remove the instances whose member variables have age (i) 21 and (ii) 29. Write a c++ function to count the elements of the linked list. Write a c++ function to search the elements from the linked list, and implement the function to search an element whose age is 23. Write a c++ function to print the linked list on the console.
Concatenate Map
This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map.
You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps.
Signature:
public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList)
Example:
INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93}
INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43}
INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…
Chapter 17 Solutions
Starting Out with C++
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - Prob. 11RQECh. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - Prob. 22RQECh. 17 - Prob. 23RQECh. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - T F The programmer must know in advance how many...Ch. 17 - T F It is not necessary for each node in a linked...Ch. 17 - Prob. 28RQECh. 17 - Prob. 29RQECh. 17 - Prob. 30RQECh. 17 - Prob. 31RQECh. 17 - Prob. 32RQECh. 17 - Prob. 33RQECh. 17 - Prob. 34RQECh. 17 - Prob. 35RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - List Template Create a list class template based...Ch. 17 - Prob. 9PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Prob. 13PCCh. 17 - Prob. 14PCCh. 17 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Need help only part 2. Thank you! In this assignment, you will create a Linked List data structure variant called a “Circular Linked List”. The Node structure is the same as discussed in the slides and defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; } For the Circular Linked List, its class definition is as follows: public class CircularLinkedList { public int currentSize; public Node current; } In this Circular Linked List (CLL), each node has a reference to an existing next node. When Node elements are added to the CLL, the structure looks like a standard linked list with the last node’s next pointer always pointing to the first. In this way, there is no Node with a “next” pointer in the CLL that is ever pointing to null. For example, if a CLL has elements “5”, “3” and “4” and “current” is pointing to “3”, the CLL should look like: Key observations with this structure:…arrow_forwardWrite C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardAssignment 6 - More on ListsWrite pseudo-code not Python for problems requiring code. You are responsible for the appropriate level of detail.The questions in this assignment give you the opportunity to explore a new data structure and to experiment with the hybrid implementation in Q3. 1. A deque (pronounced deck) is an ordered set of items from which items may be deleted at either end and into which items may be inserted at either end. Call the two ends left and right. This is an access-restricted structure since no insertions or deletions can happen other than at the ends. Implement the deque as a doubly-linked list (not circular, no header). Write InsertLeft and DeleteRight.2. Implement a deque from problem 1 as a doubly-linked circular list with a header. Write InsertRight and DeleteLeft.3. Write a set of routines for implementing several stacks and queues within a single array. Hint: Look at the lecture material on the hybrid implementation.arrow_forward
- Using user-defined function, create a C++ or Java application implementing a linked list datastructure. The application should be able to perform the following operations on the datastructure can with the following operations. i. insertion into the listii. deletion from the list iii. printing the content of the listarrow_forwardCell Phones Records In this part, you are required to write a program, using linked lists, that manipulates a set of records of cell phones and performs some operations on these records. I) The CellPhone class has the following attributes: a serialNum (long type), a brand (String type), a year (int type, which indicates manufacturing year) and a price (double type). It is assumed that brand name is always recorded as a single word (i.e. Motorola, SonyEricsson, Panasonic, etc.). It is also assumed that all cellular phones follow one system of assigning serial numbers, regardless of their different brands, so no two cell phones may have the same serial number. You are required to write the implementation of the CellPhone class. Beside the usual mutator and accessor methods (i.e. getPrice(), setYear()) the class must have the following: (a) Parameterized constructor that accepts four values and initializes serialNum, brand, year and price to these passed values; (b) Copy constructor,…arrow_forward(Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forward
- HELP Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardThe definition of linked list is given as follows: struct Node { ElementType Element ; struct Node *Next ; } ; typedef struct Node *PtrToNode, *List, *Position; If L is head pointer of a linked list, then the data type of L should be ??arrow_forwardRemove Duplicates This function will receive a list of elements with duplicate elements, this function should remove the duplicate elements in the list and return a list without duplicate elements. The elements in the returned list must be in the same order that they were found in the list the function received. A duplicate element is an element found more than one time in the specified list.arrow_forward
- C++ function Linked list Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.arrow_forwardPart-1 a. Create a doubly linked list-based DEQueue class that implements the DequeInterface. The class skeleton and interface are provided to you. b. Implement a String toString () method that creates and returns a string that correctly represents the current deque. Such a method could prove useful for testing and debugging the class and for testing and debugging applications that use the class. Assume each queued element already provides its own reasonable toString method.Example: 35<-->22<-->28<-->11<-->22<-->7<-->11<-->19arrow_forward8.19 LAB*: Program: Playlist You will be building a linked list. Make sure to keep track of both the head and tail nodes. (1) Create three files to submit. PlaylistNode.h - Class declaration PlaylistNode.cpp - Class definition main.cpp - main() function Build the PlaylistNode class per the following specifications. Note: Some functions can initially be function stubs (empty functions), to be completed in later steps. Default constructor (1 pt) Parameterized constructor (1 pt) Public member functions InsertAfter() - Mutator (1 pt) SetNext() - Mutator (1 pt) GetID() - Accessor GetSongName() - Accessor GetArtistName() - Accessor GetSongLength() - Accessor GetNext() - Accessor PrintPlaylistNode() Private data members string uniqueID - Initialized to "none" in default constructor string songName - Initialized to "none" in default constructor string artistName - Initialized to "none" in default constructor int songLength - Initialized to 0 in default constructor PlaylistNode*…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
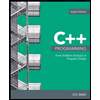
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning