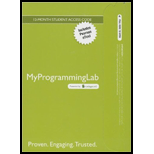
Starting out With C++, Early Objects - Access
8th Edition
ISBN: 9780133452259
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 1PC
Program Plan Intro
Simple Linked List Class
Program Plan:
- Include the required specifications into the program.
- Declare a class ListNode.
- Declare the member variables “value” and “*p” in structure named “ListNode”.
- The data value of node is stored in variable v and address to next pointer is stored in pointer p
- Declare the constructor, destructor, and member functions in the class.
- Declare the structure variable “next” and a friend class Linked List
- Declare a class LinkList.
- Function to insert elements into the linked list “void add(double n)” is defined.
- Function to check whether a particular node with a data value n is a part of linked list or not “bool isMember(double n)”.
- Declaration of structure variable head to store the first node of the list “ListNode * head” is defined.
- A function “void LinkedList::add(double n)” is defined which adds or inserts new nodes into the link list.
- A function “bool LinkedList::isMember(double n)” is defined which searches for a given data value within the nodes present in the link list.
- A destructor “LinkedList::~LinkedList()” deallocates the memory for the link list.
- Declare the main class.
- Create an empty list to enter the data values into the list.
- Input “5” numbers from user and insert the data values into the link list calling “void LinkedList::add(double n)” function.
- Check whether a data value is a member of the linked list or not by calling the “bool LinkedList::isMember(double n)” function.
- Print whether the data value entered is present within the link list or not.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In this chapter, the class to implement the nodes of a linked list is defined as a struct. The following rewrites the definition of the struct nodeType so that it is declared as a class and the member variables are private.
template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type> *ptr);//Function to set the link of the node.//Postcondition: link = ptr;nodeType<Type>* getLink() const;//Function to return the link of the node.//Postcondition: The value of link is returned.nodeType();//Default constructor//Postcondition: link = NULL;nodeType(const Type& elem, nodeType<Type> *ptr);//Constructor with parameters//Sets info point to…
In this chapter, the class to implement the nodes of a linked list is defined as a struct .
The following rewrites the definition of the struct nodeType so that it is declared as a class and the member variables are private.
template <class Type> class nodeType{ public: const nodeType<Type>& operator=(const nodeType<Type>&); //Overload the assignment operator.
void setInfo(const Type& elem); //Function to set the info of the node. //Postcondition: info = elem;
Type getInfo() const; //Function to return the info of the node. //Postcondition: The value of info is returned.
void setLink(nodeType<Type> *ptr); //Function to set the link of the node. //Postcondition: link = ptr;
nodeType<Type>* getLink() const; //Function to return the link of the node. //Postcondition: The value of link is returned.
nodeType(); //Default constructor //Postcondition: link = nullptr;…
(IntelliJ)
Write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference (Java) or either a reference or a pointer (C++) to the first node, as well as one to the last node.
* Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null.
* Create a different method or function that iteratively explores the list, printing the int data values as…
Chapter 17 Solutions
Starting out With C++, Early Objects - Access
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the…arrow_forward8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 I need the //TODO completed Main.cpp #include "MileageTrackerNode.h" #include <string> #include <iostream> using namespace std; int main (int argc, char* argv[]) { // References for MileageTrackerNode objects MileageTrackerNode* headNode; MileageTrackerNode* currNode; MileageTrackerNode* lastNode; double miles; string date; int i; // Front of nodes list headNode = new MileageTrackerNode(); lastNode = headNode; // TODO: Read in the number of nodes cin >> i int p = i; currNode…arrow_forwardwrite in c++ Define the 3 bolded functions for the following DynIntStack (linked list): class DynIntStack {private: struct Node { int value; // Value in the node Node *next; // Pointer to the next node }; Node *top; // Pointer to the stack toppublic: DynIntStack() { head = nullptr; } void push(int); //assume this is already defined void removeTop(); // removes the top element without returning it int topValue(); // returns the top element without removing it bool isEmpty() { return head == nullptr; } bool isFull() { return false; } void pushMany(int values[], int n); //add n values from the array}; Hints: void removeTop() (hint 3 lines of code) int topValue() (hint 1 line of code) void pushMany(int values[], int n) (hint 2 lines of code, use a for loop, call another function)arrow_forward
- Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardCode Restructuring: Methods for Linked List operations are given. Restructure the codes to improve performance of codes. Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html Instruction 1: Two (2) Java classes are provided, first, the Linked List class and second, the Linked List interface. On the Linked List class, check the comments, analyze then try to recode the said method/s. Post the changes in your code, if any. package com.linkedlist; public class LinkedList<E> implements ListI<E>{ //The Node Object signatures and constructor class Node<E>{ E data; Node<E> next; public Node(E obj) { data=obj; next=null; } }…arrow_forward8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward
- 8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward
- Define the 3 bolded functions for the following DynIntStack (linked list): class DynIntStack {private: struct Node { int value; // Value in the node Node *next; // Pointer to the next node }; Node *top; // Pointer to the stack toppublic: DynIntStack() { head = nullptr; } void push(int); //assume this is already defined void removeTop(); // removes the top element without returning it int topValue(); // returns the top element without removing it bool isEmpty() { return head == nullptr; } bool isFull() { return false; } void pushMany(int values[], int n); //add n values from the array}; Hints: void removeTop() (hint 3 lines of code) int topValue() (hint 1 line of code) void pushMany(int values[], int n) (hint 2 lines of code, use a for loop, call another function)arrow_forwardIn c++ , write a program to create a structure of a node, create a class Linked List. Implement all operations of a linked list as member function of this class. • create_node(int); • insert_begin(); • insert_pos(); • insert_last(); • delete_pos(); • sort(); • search(); • update(); • reverse(); • display(); ( Drop coding in words with screenshot of output as well )arrow_forwardImplement in C Programming 8.18.1: LAB: Simple linked list Given an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list: IntNode* IntNode_GetNth(IntNode* firstNode, int n)- Return a pointer to the nth node of the list starting at firstNode. void IntNode_PrintList(IntNode* firstNode) - Call IntNode_PrintNodeData() to output values of the list starting at firstNode. Do not add extra space characters in between values. int IntNode_SumList(IntNode* firstNode) - Return the sum of the values of all nodes starting at firstNode. Note: The code for IntNode_Create() provided here differs from the code shown in the book. The given main() performs various actions to test IntNode_GetNth(), IntNode_PrintList(), and IntNode_SumList(). main() reads 5 integers from a user: The number of nodes to be added to a new list The value of the first node of the list An increment between the values of two consecutive…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
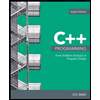
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning