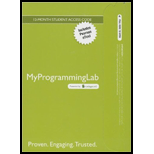
Concept explainers
A)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::printList()//Line 1
{//Line 2
//loop
//Error line3
while(head)//Line 3
{//Line 4
/*Print the data value of the node while traversing through the list*/
cout<<head->value; //Line5
/*the pointer is moved one position ahead till end of list */
head= head->next;//Line6
}//Line 7
}//Line 8
Error in the given code:
- In “line 3”, use of the head pointer to walk down the list destroys the list.
- This should be written as an “auxiliary pointer”. So, correct code is given below:
ListNode *nodePtr = head
- This should be written as an “auxiliary pointer”. So, correct code is given below:
while (nodePtr != null)
;&#x...
B)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
//Error Line4
while (p->next) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Line7
p=p->next; //the pointer is moved one position ahead till end of list
}//Line8
}//Line9
Error in the given code:
- In “line 4”, eventually the pointer p becomes “NULL”, at which time the attempt to access p->NULL will result in an error.
- This should be written by replacing the text p->next in the while loop with p.
while (p) //Line4
/*Here the loop will traverse till p exists or till p is not NULL */
- The function fails to declare a return type of void...
C)
Explanation of Solution
Purpose of the given code:
The given code is trying to print the members of a linked list by traversing through the entire list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::PrintList()//Line 1
{//Line 2
//Declaration of structure pointer variables
//Line3
ListNode *p =head; /*the start or head of the list is stored in p */
//loop
// Line4
while (p) //Line4
{//Line 5
/*Print the data value of node p while traversing through the linklist */
//Line6
cout<<p->value; /*print the individual data values of each node */
//Error Line7
//Line7
p++//the pointer is incremented by one position
}//Line8
}//Line9
Error in the given code:
- In “line 7”, the function uses p++ erroneously in place of p=p->next when attempting to move to the next node in the list. This is not possible as increment operator can work only on variables containing data values but here p is a node of a link list which contains an address value pointer pointing to the next list along with a data value.
- This should be written by replacing the text p++ in the while loop body with p=p->next...
D)
Explanation of Solution
Purpose of the given code:
The given code is trying to destroy the members of a linked list by using a destructor. A destructor is called when the program ends or the destructor function calls.
Given Code:
//Definition of destructor
NumberList::~NumberList()//Line 1
{//Line 2
//Declaration of structure pointer variables
ListNode *nodePtr, *nextNode;//Line 3
//Storing "head" pointer into "nodePtr"
nodePtr = head;//Line 4
//loop
while (nodePtr != nullptr)//Line 5
{//Line 6
//Assign address of next into "nextNode"
nextNode = nodePtr->next;//Line 7
//Error
nodePtr->next=nullptr;//Line 8
//Assign nextNode into "nodePtr"
nodePtr = nextNode;//Line 9
}//Line 10
}//Line 11
Error in the given code:
In “line 8”, the address of “next” in “nodePtr” is assigned as “nullptr”.
- This should be written as “delete nodePtr” to delete the value of node from the list, because, “delete” operator is used to free the memory space allocated by the list...

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
Starting out With C++, Early Objects - Access
- Complete the following function where a node is perculated through a MaxHeap when given the value of the parent node (through the use of a linked list) void BinMaxHeap::percolateUp(BHNode *p) { }arrow_forwardA double-ended queue or deque is a generalization of a stack and a queue that supports adding and removing items from either the front or the back of the data structure. This assignment has two parts: Part-1 Create a doubly linked list based DeQueDLL class that implements the DequeInterface. The class skeleton and interface are provided to you. Implement a String toString () method that creates and returns a string that correctly represents the current deque. Such a method could prove useful for testing and debugging the class and for testing and debugging applications that use the class. Assume each queued element already provides its own reasonable toString method. Part-2 Create an application program that gives a user the following three options to choose from – insert, delete, and quit. If the user selects ‘insert’, the program should accept the integer input from the user and insert it into the deque in a sorted manner. If the user selects ‘delete’, the program should…arrow_forwardclass Node: def __init__(self, e, n): self.element = e self.next = n class LinkedList: def __init__(self, a): # Design the constructor based on data type of a. If 'a' is built in python list then # Creates a linked list using the values from the given array. head will refer # to the Node that contains the element from a[0] # Else Sets the value of head. head will refer # to the given LinkedList # Hint: Use the type() function to determine the data type of a self.head = None # To Do # Count the number of nodes in the list def countNode(self): # To Do # Print elements in the list def printList(self): # To Do # returns the reference of the Node at the given index. For invalid index return None. def nodeAt(self, idx): # To Doarrow_forward
- In c++ , write a program to create a structure of a node, create a class Linked List. Implement all operations of a linked list as member function of this class. • create_node(int); • insert_begin(); • insert_pos(); • insert_last(); • delete_pos(); • sort(); • search(); • update(); • reverse(); • display(); ( Drop coding in words with screenshot of output as well )arrow_forwardJAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forwardUsing C languge, implement programmer defined-data types with linked lists. A set of integers may be implemented using a linked list.Implement the following functions given the definition:typedef struct node* nodeptr;typedef struct node{int data;nodeptr next;}Node;typedef Node* Set;Set initialize();- simply initialize to NULLvoid display(Set s);- display on the screen all valid elements of the listSet add(Set s, elem);- simply store elem in the listint contains(Set s, int elem);- search the array elements for the value elemSet getUnion(Set result, Set s1, Set s2);- store in the set result the set resulting from the union of s1 and s2- x is an element of s1 union s2 if x is an element of s1 or x is an element of s2Set intersection(Set result, Set s1, Set s2);- store in the set result the set resulting from the intersection of s1 and s2- x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2Set difference(Set result, Set s1, Set s2);- store in the set…arrow_forward
- Code Restructuring: Methods for Linked List operations are given. Restructure the codes to improve performance of codes. Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html Instruction 1: Two (2) Java classes are provided, first, the Linked List class and second, the Linked List interface. On the Linked List class, check the comments, analyze then try to recode the said method/s. Post the changes in your code, if any. package com.linkedlist; public class LinkedList<E> implements ListI<E>{ //The Node Object signatures and constructor class Node<E>{ E data; Node<E> next; public Node(E obj) { data=obj; next=null; } }…arrow_forwardPart2: LinkedList implementation 1. Create a linked list of type String Not Object and name it as StudentName. 2. Add the following values to linked list above Jack Omar Jason. 3. Use addFirst to add the student Mary. 4. Use addLast to add the student Emily. 5. Print linked list using System.out.println(). 6. Print the size of the linked list. 7. Use removeLast. 8. Print the linked list using for loop for (String anobject: StudentName){…..} 9. Print the linked list using Iterator class. 10. Does linked list hava capacity function? 11. Create another linked list of type String Not Object and name it as TransferStudent. 12. Add the following values to TransferStudent linked list Sara Walter. 13. Add the content of linked list TransferStudent to the end of the linked list StudentName 14. Print StudentName. 15. Print TransferStudent. 16. What is the shortcoming of using Linked List?arrow_forwardJava/Data Structures: The public ArrayList() constructor in the Java Class Library ArrayList creates an empty list with an initial capacity of ____. Multiple choice. A) 0 B) 1 C) 10 D) 100arrow_forward
- C Language In a linear linked list, write a function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list.arrow_forwardThink about the following example: A computer program builds and modifies a linked list like follows:Normally, the program would keep tabs on two unique nodes, which are as follows: An explanation of how to use the null reference in the linked list's node in two common circumstancesarrow_forwardIn Java. The following is a class definition of a linked list Node:class Node{int info;Node next;}Show the instructions required to create a linked list that is referenced by head and stores in order, the int values 13, 6 and 2. Assume that Node's constructor receives no parameters.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
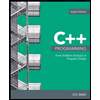