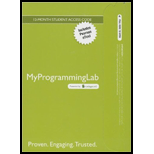
Starting out With C++, Early Objects - Access
8th Edition
ISBN: 9780133452259
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 7PC
Program Plan Intro
List Search
Program Plan:
- Include the required specifications into the program.
- Declare a class ListNode.
- Declare the member variables “value” and “*p” in structure named “ListNode”.
- The data value of node is stored in variable v and address to next pointer is stored in pointer p.
- Declare the constructor, destructor, and member functions in the class.
- Declare the structure variable “next” and a friend class Linked List.
- Declare a class LinkList.
- Function to insert elements into the linked list “void add(double n)”is defined.
- Function to check whether a particular node with a data value n is a part of linked list or not “bool isMember(double n)”.
- A recursive print method is defined to print all the data values present in the link list “void rPrint()”.
- A method to search for an element present in the link list called “int LinkedList::search(double x)” is called that returns the position of the element present in the link list.
- A destructor is called to delete the desired data value entered by the user called “LinkedList::~LinkedList( )”.
- A method to remove the element passed as a parameter from the link list is called “void LinkedList::remove(double x)”.
- Declare a method “void reverse( )” to reverse the elements present in the link list by traversing through the list and Move node at end to the beginning of the new reverse list being constructed.
- Declaration of structure variable head to store the first node of the list “ListNode * head” is defined.
- A function “void LinkedList::add(double n)” is defined which adds or inserts new nodes into the link list.
- A function “bool LinkedList::isMember(double n)” is defined which searches for a given data value within the nodes present in the link list.
- A destructor “LinkedList::~LinkedList()” deallocates the memory for the link list.
- A function “void LinkedList::print()” is used to print all the node data values present in the link list by traversing through each nodes in the link list.
- A recursive member function check is defined called “bool LinkedList::rIsMember(ListNode *pList,double x)” .
- If the data value entered is present within the link list, it returns true, else it returns false.
- Declare the main class.
- Create an empty list to enter the data values into the list.
- Copy is done using copy constructor.
- Input “5” numbers from user and insert the data values into the link list calling “void LinkedList::add(double n)” function.
- Print the data values of the nodes present in the link list.
- “int LinkedList::search(double x)” function is called to search the element present in the link list and prints the position of the element.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a struct Student that has member variables: (string) first name, (int) age and (double) fee. Write the functions as described in the class for the following purposes.1. Write a c++ function to create a dynamic sorted (in ascending order according to the age)doubly linked list, where the data component of each node is an instance of the structStudent.2. Write a function to insert the instances in the linked list. You also need to write a function to find the spot for insertion of the nodes.3. Write a function to remove the node from the linked list.4. Write a function to count the elements of the linked list.5. Write a function to determine check whether an element belongs to the linked list.6. Write a function to print the linked list (from head node) on the console.7. Write a function to print the linked list (from tail node) on the console.
Implement the above functions as follows.Initially, the list must have five nodes that are the instances of the struct whose member…
Write a struct ‘Student’ that has member variables: (string) first name, (int) age and (double) fee. Create a sorted (in ascending order according to the age) linked list of three instances of the struct Student. The age of the students must be 21, 24 and 27. Write a function to insert the new instances (elements of the linked list), and insert three instance whose member variable have age (i) 20, (ii) 23 and (iii) 29. Write a function to remove the instances from the linked list, and remove the instances whose member variables have age (i) 21 and (ii) 29. Write a function to count the elements of the linked list. Write a function to search the elements from the linked list, and implement the function to search an element whose age is 23. Write a function to print the linked list on the console.
Consider the Student struct as defined above. Create a stack of 5objects of the class. Implement the following (i) push an element to the stack (ii) pop an element from the stack (iii) get the…
HELP
Write C code that implements a soccer team as a linked list.
1. Each node in the linkedlist should be a member of the team and should contain the following information:
What position they play
whether they are the captain or not
Their pay
2. Write a function that adds a new members to this linkedlist at the end of the list.
Chapter 17 Solutions
Starting out With C++, Early Objects - Access
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ (Last time the solution was unclear with errors) Write a class template that uses a vector to implement a stack data structure with these member functions: 1. (constructor) : Construct stack (public member function ) 2. empty : Test whether container is empty (public member function ) 3. size : Return size (public member function ) 4. top : Access next element (public member function ) 5. push : Insert element (public member function ) 6. pop : Remove top element (public member function ) Put function definitions outside the definition of the class (i.e. inline functions are not allowed).arrow_forwardWrite a struct ‘Student’ that has member variables: (string) first name, (int) age and (double) fee. Create a sorted (in ascending order according to the age) linked list of three instances of the struct Student. The age of the students must be 21, 24 and 27. Write a c++ function to insert the new instances (elements of the linked list), and insert three instance whose member variable have age (i) 20, (ii) 23 and (iii) 29. Write a c++ function to remove the instances from the linked list, and remove the instances whose member variables have age (i) 21 and (ii) 29. Write a c++ function to count the elements of the linked list. Write a c++ function to search the elements from the linked list, and implement the function to search an element whose age is 23. Write a c++ function to print the linked list on the console.arrow_forwardC++ function Linked list Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.arrow_forward
- Write a function nth_member(data, n) that takes a list data and an integer n as parameters and returns the nth member of the list of data, assuming the first member has an index of n = 0. You may assume that data contains at least n + 1 members.arrow_forwardwrite in c++ Define the 3 bolded functions for the following DynIntStack (linked list): class DynIntStack {private: struct Node { int value; // Value in the node Node *next; // Pointer to the next node }; Node *top; // Pointer to the stack toppublic: DynIntStack() { head = nullptr; } void push(int); //assume this is already defined void removeTop(); // removes the top element without returning it int topValue(); // returns the top element without removing it bool isEmpty() { return head == nullptr; } bool isFull() { return false; } void pushMany(int values[], int n); //add n values from the array}; Hints: void removeTop() (hint 3 lines of code) int topValue() (hint 1 line of code) void pushMany(int values[], int n) (hint 2 lines of code, use a for loop, call another function)arrow_forwardLINKED LIST IMPLEMENTATION Linked list Write a C++ program to implement insertion, deletion, and display operations in a Linked List Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.The class Node has the following member variable Datatype Variable Usage int data to store data Node* next to store the next node Define the following public member functions in the class LinkedList. Member function Function description void insertNode(int value) This function inserts the data into the linked list at the end void deleteNode(int value) This function deletes the node from the linked list void display() This function is used to display the nodes in the linked list In the main() function, read inputs and call the functions of the LinkedList class based on the inputs. Note: If the Linked list is empty while…arrow_forward
- Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forwardIn C++ Plz LAB: Grocery shopping list (linked list: inserting at the end of a list) Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts ItemNode.h Default Code: #include <iostream>#include <string>using namespace std; class ItemNode {private: string item; ItemNode* nextNodeRef; public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; } // Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; } // ConstructorItemNode(string itemInit, ItemNode *nextLoc) {this->item = itemInit;this->nextNodeRef = nextLoc;} // Insert node after this…arrow_forwardUnordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forward
- (Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forwardCreate a ( operator overlod = ) for a doubly linked list, in C++. for this class #include<iostream>using namespace std; class Node{ public: int data; Node *next, *prev; Node(int val){ data = val; next = NULL; prev = NULL; }}; class DLL{ private: Node *root; public: //default constructor DLL(){ root = NULL; } //copy constructor DLL(const DLL& list){ Node *newNode, *tail; Node *current = list.root; while(current != NULL){ newNode = new Node(current->data); if(root == NULL) { root = newNode; tail = root; } else{ tail->next = newNode; newNode->prev = tail; tail = newNode; } current =…arrow_forwardin C++... kth Element Extend the class linkedListType by adding the following operations:a. Write a function that returns the info of the kth element of the linked list. If no such element exists, terminate the program.b. Write a function that deletes the kth element of the linked list. If no such element exists, terminate the program. Provide the definitions of these functions in the class linkedListType. PLEASE DON'T reject this question, this is the whole question that I have... so please do it however u can, Thank you!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
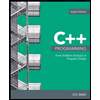
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning