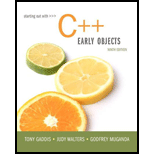
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 8PC
Program Plan Intro
Member Insertion by Position
Program Plan:
- Include the required specifications into the program.
- Declare a class ListNode.
- Declare the member variables “value” and “*p” in structure named “ListNode”.
- The data value of node is stored in variable v and address to next pointer is stored in pointer p
- Declare the constructor, destructor, and member functions in the class.
- Declare the structure variable “next” and a friend class Linked List
- Declare a class LinkList.
- Function to check whether a particular node with a data value n is a part of linked list or not “bool isMember(double n)”.
- A recursive print method is defined to print all the data values present in the link list “void rPrint()”.
- A link list method to insert elements into the link list called “void LinkedList::insert(double x, int pos)” is called that insert elements into the link list at specific positions.
- A method to search for an element present in the link list called “ int LinkedList::search(double x)” is called that returns the position of the element present in the link list.
- A destructor is called to delete the desired data value entered by the user called “LinkedList::~LinkedList( )”.
- A method to remove the element passed as a parameter from the link list is called “void LinkedList::remove(double x)”.
- Declare a method “void reverse( )” to reverse the elements present in the link list by traversing through the list and Move node at end to the beginning of the new reverse list being constructed.
- Declaration of structure variable head to store the first node of the list “ListNode * head” is defined.
- A function “void LinkedList::add(double n)” is defined which adds or inserts new nodes into the link list.
- A function “bool LinkedList::isMember(double n)” is defined which searches for a given data value within the nodes present in the link list.
- A destructor “LinkedList::~LinkedList()” deallocates the memory for the link list.
- A function “void LinkedList::print()” is used to print all the node data values present in the link list by traversing through each nodes in the link list.
- A recursive member function check is defined called “bool LinkedList::rIsMember(ListNode *pList,double x)” .
- If the data value entered is present within the link list, it returns true, else it returns false.
- Declare the main class.
- Create an empty list to enter the data values into the list.
- Copy is done using copy constructor.
- Input “5” numbers from user and insert the data values into the link list calling “void LinkedList::add(double n)” function.
- Print the data values of the nodes present in the link list.
- “int LinkedList::search(double x)” function is called to search the element present in the link list and prints the position of the element.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ function Linked list
Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.
CLASSES, DYNAMIC ARRAYS AND POINTERS
Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string).
Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list.
Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list.
in C++
Write member functions to:
remove and return the last line from the list
add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array
empty the entire list
return the number of lines still on the list
take two lists and return one combined list (with no duplicates)
copy constructor to support deep copying
remember the destructor!
C++The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the copy constructor to List.
List (const List & list) {
for (int i = 0; i <list.size (); i ++) {
push_back (list [i]);
}
}
What problems does the copy constructor have?
Select one or more options:
1. List (const List & list) {} does not define a copy constructor.
2. The list parameter should not be constant.
3. The new elements will be added to the wrong list.
4. Copy becomes shallow rather than deep.
5. The copy will create dangling pointers.
6. Copying will create memory leaks.
7. The condition must be: i <size ()
8. head is never initialized.
Chapter 17 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Computer Science //iterator() creates a new Iterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::Iterator DoublyLinkedList<ValueType>::iterator(){//return iterator(head);} //constIterator() creates a new ConstIterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::ConstIterator DoublyLinkedList<ValueType>::constIterator() const{//return constIterator(head);} //Initializes a newly-constructed IteratorBase to operate on//the given list. It will initially be referring to the first//value in the list, unless the list is empty, in which case//it will be…arrow_forwardUnordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forwardCount dominators def count_dominators(items): An element of items is said to be a dominator if every element to its right (not just the one element that is immediately to its right) is strictly smaller than it. By this definition, the last item of the list is automatically a dominator. This function should count how many elements in items are dominators, and return that count. For example, dominators of [42, 7, 12, 9, 13, 5] would be the elements 42, 13 and 5. Before starting to write code for this function, you should consult the parable of "Shlemiel the painter" and think how this seemingly silly tale from a simpler time relates to today's computational problems performed on lists, strings and other sequences. This problem will be the first of many that you will encounter during and after this course to illustrate the important principle of using only one loop to achieve in a tiny fraction of time the same end result that Shlemiel achieves with two nested loops. Your workload…arrow_forward
- Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.arrow_forwardC++ The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the destructor to List. ~ List () { for (int i = 0; i <size (); i ++) { pop_back (); } } What problems does the destructor have? Select one or more options: 1. There are no parameters for the destructor. 2. The return value from pop_back (if any) is nerver handled. 3. The destructor will create a stack overflow. 4. The destructor will create dangling pointers. 5.The destructor will create memory leaks. 6.The destructor will create undefined behavior (equivalent to zero pointer exception). 7.The condition must be: i <size () - 1 8. There is at least one problem with the destructor, but none of the above.arrow_forwardMUST BE DONE IN C#!!! Create a class called StudentGrades. This class should be designed based off the following UML Diagram: class StudentGrades -double list grades_list +<constructor> +AddGrade(double): void +RemoveGrade(double): bool +GetClassAverage(): double +GetHighestGrade(): double +GetLowestGrade(): double Method Descriptions: a. <constructor> - creates an empty array for the grades_list array. b. AddGrade – Adds the passed in grade to the list. Note that any number that is NOT 0 – 100 should be rejected. c. RemoveGrade – Removes the first occurrence of the passed in grade from the list. If the grade is not found, the method should return false. d. GetClassAverage() – Average the grades in the list and return the average. e. GetHighestGrade - Find and return the highest grade in the list. f. GetLowestGrade – Find and return the lowest grade in the list. In main, create a StudentGrades object to test the listed methods. Add 10 grades randomly and call each…arrow_forward
- In C++ Plz LAB: Grocery shopping list (linked list: inserting at the end of a list) Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts ItemNode.h Default Code: #include <iostream>#include <string>using namespace std; class ItemNode {private: string item; ItemNode* nextNodeRef; public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; } // Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; } // ConstructorItemNode(string itemInit, ItemNode *nextLoc) {this->item = itemInit;this->nextNodeRef = nextLoc;} // Insert node after this…arrow_forward1. Design your own linked list class to hold a series of integers using C++. The class should have member functions for appending, inserting, and deleting nodes. Don't forget to add a destructor that destroys the list. Demonstrate the class with a driver program. 2. Modify the linked list class you created to add a print member function. The function should display all the values in the linked list. Test the class by starting with an empty list, adding some elements, and then printing the resulting list out.arrow_forwardProgramming in C: Challenge 1: Create and Print a Doubly Linked List In class, I briefly mentioned how to create a doubly linked list (a linked list with two directions: forward and backwards). This task challenges you to create a doubly linked list of 5 nodes. If your nodes were called n1, n2, n3, n4 and n5 (which they shouldn’t, please give them original names), your list should be linked as follows: We discussed what "start" and "end" should be. Then, also in main, you will have to print the list forwards by taking advantage of the arrows pointing to the right. You will also have to print it backwards taking advantage of the arrows pointing to the left. Again, everything must be done in main. Hint: look at the way in which I printed the singly linked list in class. In other words, if your nodes had the values: n1=1, n2=2, n3=3, n4=4 and n5=5; your program should print:1 3 5 2 4 (forwards) and 4 2 5 3 1 (backwards). Please give different values to your nodes, they should not have…arrow_forward
- Exercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forward(Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forward8.19 LAB*: Program: Playlist You will be building a linked list. Make sure to keep track of both the head and tail nodes. (1) Create three files to submit. PlaylistNode.h - Class declaration PlaylistNode.cpp - Class definition main.cpp - main() function Build the PlaylistNode class per the following specifications. Note: Some functions can initially be function stubs (empty functions), to be completed in later steps. Default constructor (1 pt) Parameterized constructor (1 pt) Public member functions InsertAfter() - Mutator (1 pt) SetNext() - Mutator (1 pt) GetID() - Accessor GetSongName() - Accessor GetArtistName() - Accessor GetSongLength() - Accessor GetNext() - Accessor PrintPlaylistNode() Private data members string uniqueID - Initialized to "none" in default constructor string songName - Initialized to "none" in default constructor string artistName - Initialized to "none" in default constructor int songLength - Initialized to 0 in default constructor PlaylistNode*…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
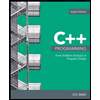
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
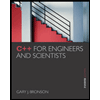
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr