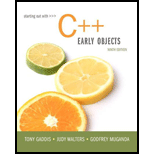
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 15PC
Program Plan Intro
Stack Based evaluation of prefix expression
Program Plan:
- Declare a structure to create a stack element.
- Include all the required header files.
- Declare a function to input prefix statements.
- Declare a Function int evaluate that evaluates the prefix expression by considering if the next token in the input stream is an integer, read the integer and push it onto the stack using the push() operation of the stack .
- But if the input stream is an operator, pop the last two values from the stack using the pop operation and apply the operator, and push the result onto the stack and the lone value is the result.
- Declare the function bool prefix_reducible that returns true if there are at least three elements on the stack and top two elements are values and the third from the top is an operator.
- Declare the main function.
- Prompt the user to enter a prefix expression.
- Evaluate the prefix expression by calling the int evaluate function and print the result.
- Prompt the user to enter a prefix expression.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Add remain code and explanation of whole code.
Given a stack, a function is_consecutive takes a stack as a parameter and thatreturns whether or not the stack contains a sequence of consecutive integersstarting from the bottom of the stack (returning true if it does, returningfalse if it does not).
For example:bottom [3, 4, 5, 6, 7] topThen the call of is_consecutive(s) should return true.bottom [3, 4, 6, 7] topThen the call of is_consecutive(s) should return false.bottom [3, 2, 1] topThe function should return false due to reverse order.
Note: There are 2 solutions:first_is_consecutive: it uses a single stack as auxiliary storagesecond_is_consecutive: it uses a single queue as auxiliary storage"""import collections
def first_is_consecutive(stack): storage_stack = [] for i in range(len(stack)): first_value = stack.pop() if len(stack) == 0: # Case odd number of values in stack return True second_value = stack.pop() if first_value -…
(Postfix Evaluation) Write a program that evaluates a valid postfix expression such as
6 2 + 5 * 8 4 / -The program should read a postfix expression consisting of digits and operators into a string. Using modified versions of the stack functions implemented earlier in this chapter, the program should scan the expression and evaluate it. The algorithm is as follows:
While you have not reached the end of the string, read the expression from left to right.
If the current character is a digit,
Push its integer value onto the stack (the integer value of a digit character is its value in the computer’s character set minus the value of '0' in the computer’s character set).
Otherwise, if the current character is an operator,
Pop the two top elements of the stack into variables x and y.
Calculate y operator x.
Push the result of the calculation onto the stack.
When you reach the end of the string, pop the top value of the stack. This is the result of the postfix expression.
[Note: In Step 2…
Min Stack (interesting stack implementation):
Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.
Implement the MinStack class:
MinStack() initializes the stack object.
void push(int val) pushes the element val onto the stack.
void pop() removes the element on the top of the stack.
int top() gets the top element of the stack.
int getMin() retrieves the minimum element in the stack.
You must implement a solution with O(1) time complexity for each function.
Code in Java.
A brief explanation of how and why your code works and how it relates to the concepts learned (for example, it uses bitwise operation, stack, or takes into account a word size).
Chapter 18 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- Balancing Parenthesis Input Output ((())) Balance (())) Unbalance (()()(())) Balance Given a sequence consisting of parentheses, determine whether the expression is balanced. A sequence of parentheses is balanced if every open parenthesis can be paired uniquely with a closing parenthesis that occurs after the former. Algorithm: Create an empty stack using list. Allow user to enter the set of parentheses in string format Read each character of the string (parentheses) from left to right If the character is an open parenthesis then push It onto the stack Else check stack if empty If yes then Print a message “Unbalance” and exit loop If no pop once and proceed to the next step Repeat step 3 steps until the last parenthesis. Check stack. If empty then print message “Balance” else “Unbalance” Note: Assuming all inputs are valid. Error and exception handling not neededarrow_forward(Printing a Sentence in Reverse Order with a Stack) Write a program that inputs a line of text and uses a stack object to print the line reversed.arrow_forwardIn a stack, if a user tries to remove an element from an empty stack it is called a) Underflow b) Empty collection c) Overflow d) Garbage Collectionarrow_forward
- Q1: Write a java application for the stack operations with Linked List. Q2: Write java program to take the order from the customer and display the ordered items and total payment. If customer is entering the wrong name or not following the order to enter the menu it has to display error messages. (Find in the sample code) Sample Output:arrow_forwardJAVA LANGUAGE CODE Postfix Calculator by CodeChum Admin One good implementation of computing infix expressions is to transform them to postfix and then evaluate via the postfix expression. Infix expressions is the common way of writing arithmetic expressions. The binary operator come between them as shown below: 2 * 5 + 9 - 10 / 20 In postfix expressions, the operands come first before the operator: 2 5 * 9 + 10 20 / - A stack can be used to evaluate postfix expressions. The operands are pushed onto the Stack and when an operator is found two operands are popped and the operation is performed and finally the result is pushed back onto the Stack. The final answer will be the lone element of the Stack. Input The first line contains a positive integer n representing the number of postfix expressions. What follows are n postfix expressions themselves. 5 10 20 + 30 15 2 * + 100 20 30 + / 90 20 10 + + 0 / 9 3 - 10 + 2 * Output A single line containing the result of…arrow_forwardC# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. private int [] stack; private int size; public void ReverseStack(){ }arrow_forward
- struct Faculty { int id; char name[30]; char status[10]; //status permanent or visiting Faculty next; }; Assume we have three stacks S, V and P. S is a stack having mix data of visiting and permanent faculty members, V is empty and for visiting faculty memebrs and P is also empty for permanent faculty members. Using primitive functions of stack write a C++ code which reads entire data from S and write on respective stack V or P.arrow_forwardWrite the program by completing the main program that doesthe following:1. Call the push function three times.2. Prints out the updated stack3. Calls the pop function once4. Print the updated stack again.No need to write the algorithm for this problem, it is already given to you.#include <stdio.h>#define STACK_EMPTY '0'#define STACK_SIZE 20voidpush(char stack[], /* input/output - the stack */char item, /* input - data being pushed onto the stack */int *top, /* input/output - pointer to top of stack */int max_size) /* input - maximum size of stack */{if (*top < max_size-1) {++(*top);stack[*top] = item;}}charpop (char stack[], /* input/output - the stack */int *top) /* input/output - pointer to top of stack */{char item; /* value popped off the stack */if (*top >= 0) {item = stack[*top];--(*top);} else {item = STACK_EMPTY;}return (item);}intmain (void){char s [STACK_SIZE];int s_top = -1; // stack is empty/* complete the program here */return (0);}arrow_forwardMachine organization The following operations are performed on a stack: PUSH A, PUSH B, POP, PUSH C, POP,POP,PUSH D, PUSH E, POP, PUSH F. What does the stack contain after each operation?arrow_forward
- Data Structure & Algorithm: Write a program to perform the following operations on a stack. a) Create functions for push and pop operations of the stack.b) Write a function to convert an infix expression to a postfix expression. Pass a one-dimensional character array P to the function as input (infix exp) and return character array Q (postfix exp). Test your program for the following input P : ( A – (B / C ) * D + E ) * F % G c) Write a function for the evaluation of a given postfix expression. For testing pass the postfix expression Q of part b and supply the following set of values. A = 90, B = 50, C = 2, D = 3, E = 1, F = 2, G = 5arrow_forwardIn C programming language please Write a program that uses a stack to check if a given string is a palindrome or not. A palindrome is a word, phrase, number, or other sequence of characters that reads the same backward as forward. Your program should use a stack to reverse the characters of the given string, and then compare the reversed string with the original string to determine if it is a palindrome or not. Here are some additional requirements for your program: Your program should prompt the user to enter a string and read it from the standard input. Your program should define a stack data structure with push() and pop() functions to store and retrieve characters. Your program should use the stack to reverse the characters of the input string and store the reversed string in a separate variable. Your program should then compare the reversed string with the original string to determine if it is a palindrome. Your program should output whether or not the input string is a…arrow_forwardJava Files Recursive.java RecursiveDemo.java The purpose of the assignment is to practice writing methods that are recursive. We will write four methods each is worth 15 points. a- int sum_sqr_rec(stack<int> stk) which will receive a stack of "int" and output the sum of the squares of the elements in the stack. b- int plus_minus_rec(stack<int> stk) which will receive a stack of "int" (example: {a,b,c,d,e,f,g,h,i,j}) and output the sum of the elements in the stack as follows: a - b + c - d + e - f + g - h + i -j c- void prt_chars_rev_rec(stack<char> stk) which will receive a stack of "char" and print its elements in reverse. d- void prt_chars_rec(queue<char> stk) which will receive a queue of "char" and print its elements Remember to use the stack and queue STL. The Assignment will require you to create 2 files: Recursive.java which contains the details of creating the 4 methods as specified above: int sum_sqr_rec(stack<int> stk), (15 points)…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
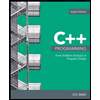
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning