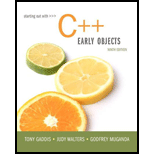
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 5PC
Program Plan Intro
Error Testing
Dynamic Stack:
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “dstack” for stack.
- Declare a variable named “popElem”.
- Push 3 elements inside the stack using the function “push ()”.
- Pop 3 elements from the stack using the function “pop ()”.
DynIntStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynIntStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack.
- Create an object for the template.
- Create a stack pointer name “next”.
- Create a stack pointer name “top”.
- Give structure declaration for the stack.
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Define function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node,
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Define function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top most node and make temp as the top node.
- Define function definition for “isEmpty ()”.
- Assign Boolean value to the variable.
- Check if the top node is null.
- Assign true to “status”.
- Return the status.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
for c++ thank you
Error Testing
The DynIntStack and Dyn IntQueue classes shown in this chapter are abstract data types using a dynamic stack and dynamic queue, respectively. The classes do not cur- rently test for memory allocation errors. Modify the classes so they determine whether new nodes cannot be created by handling the bad_alloc exception.
here is the extention.h file please use it
#ifndef DYNINTQUEUE_H
#define DYNINTQUEUE_H
class DynIntQueue
{
private:
// Structure for the queue nodes
struct QueueNode
{
int value; // Value in a node
QueueNode *next; // Pointer to the next node
};
QueueNode *front; // The front of the queue
QueueNode *rear; // The rear of the queue
int numItems; // Number of items in the queue
public:
// Constructor
DynIntQueue();
// Destructor
~DynIntQueue();
// Queue operations
void enqueue(int);
void dequeue(int &);
bool isEmpty() const;
bool isFull() const;
void…
Project 4: Maze Solver (JAVA)
The purpose of this assignment is to assess your ability to:
Implement stack and queue abstract data types in JAVA
Utilize stack and queue structures in a computational problem.
For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end.
The following code and related files are provided. Carefully ready the code and documentation before beginning.
A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited.
A Source file that creates the maze and calls your depth-first search method.
An input file, maze.in, that may be used for testing.
You…
stacks and queues.
program must be able to handle all test cases without causing an exception
Note that this problem does not require recursion to solve (though you can use that if you wish).
Chapter 18 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- JAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forwardWrite a struct Student that has member variables: (string) first name, (int) age and (double) fee. Write the functions as described in the class for the following purposes.1. Write a c++ function to create a dynamic sorted (in ascending order according to the age)doubly linked list, where the data component of each node is an instance of the structStudent.2. Write a function to insert the instances in the linked list. You also need to write a function to find the spot for insertion of the nodes.3. Write a function to remove the node from the linked list.4. Write a function to count the elements of the linked list.5. Write a function to determine check whether an element belongs to the linked list.6. Write a function to print the linked list (from head node) on the console.7. Write a function to print the linked list (from tail node) on the console. Implement the above functions as follows.Initially, the list must have five nodes that are the instances of the struct whose member…arrow_forwardJava - For 7 and 8 use the following definition: class node { boolean data; node link; } 7. Consider a structure using a linked list in which you added and removed from the back of the list. Using the node definition above, write the pseudocode for a member method “add” to this structure. Assume the existence of a “front” and “back” reference. 8. Assume you are working with a stack implementation of the linked list definition above (above question #7), write a member method “pop”. The method should return a value (in the “popped” node). Assume the existence of the node references called “TheStack” and “Top”. These references point to the start (or bottom) and top of the stack (or back of the list).arrow_forward
- // FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…arrow_forward// FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…arrow_forwardC# Programing Language Given the code below 1.Change all of the low-level arrays in your program to use the appropriate C# collection class instead. Be sure to implement Deck as a Stack 2. Make sure that you use the correct type-safe generic designations throughout your program. 3.Write code that watches for that incorrect data and throws an appropriate exception if bad data is found.4. Write Properties instead of Getter and Setter methods. no lamdas, no delegates, no LINQ, no eventsGo Fish using System; namespace GoFish{public enum Suit { Clubs, Diamonds, Hearts, Spades };public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King }; // ----------------------------------------------------------------------------------------------------- public class Card{public Rank Rank { get; private set; }public Suit Suit { get; private set; } public Card(Suit suit, Rank rank){this.Suit = suit;this.Rank = rank;} public override string ToString(){return ("[" +…arrow_forward
- Programming Exercise 8 asks you to redefine the class to implement the nodes of a linked list so that the instance variables are private. Therefore, the class linkedListType and its derived classes unorderedLinkedList and orderedLinkedList can no longer directly access the instance variables of the class nodeType. Rewrite the definitions of these classes so that these classes use the member functions of the class nodeType to access the info and link fields of a node. Also write programs to test various operations of the classes unorderedLinkedList and orderedLinkedList. template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type>…arrow_forward1. Assume the existence of a class Stack whose implementation is unknown (array versus nodes), withoperationspushandpop. Write a Stack methodvoid reverseStack()that reverses the order of theelements contained in the Stack. You can assume the existence of any other ADT data structures andtheir operations.arrow_forwardTwo stacks of the same kind are the same if they contain the same number of elements and have the same items at the same places. Overload the relational operator == for the class stackType, which returns true if two stacks of the same type are identical; otherwise, it returns false. Also, write the function template definition to overload this operator. Create a programme to test the overloaded operators and functions of the stackType class.arrow_forward
- for c++ need three files Dynlntstack, Dynlntqueue, and cpp file use those file i uploaded just creat cpp file please type the code so i can copy Error Testing The DynIntStack and DynIntQueue classes shown in this chapter are abstract data types using a dynamic stack and dynamic queue, respectively. The classes do not cur- rently test for memory allocation errors. Modify the classes so they determine whether new nodes cannot be created by handling the bad_alloc exception. here is the extention.h file please use it #ifndef DYNINTQUEUE_H #define DYNINTQUEUE_H class DynIntQueue { private: // Structure for the queue nodes struct QueueNode { int value; // Value in a node QueueNode *next; // Pointer to the next node }; QueueNode *front; // The front of the queue QueueNode *rear; // The rear of the queue int numItems; // Number of items in the queue public: // Constructor DynIntQueue(); // Destructor ~DynIntQueue(); //…arrow_forwardTrue or False For each statement below, indicate whether you think it is True or False. If you like, you can provide a description of your answer for partial credit in case you are incorrect. Given a stack implemented as an array, and the following functions performed: Push(A), Push (B), Push(C), Pop(), Push(D), Push(E), Pop(), Push(F) The array will be [A][B][D][F] and the “top” of the stack will be at index 3 where [F] is The next element that will be removed when Pop is called will be A If we Push(G), [G] will be inserted at index 0 Given a queue implemented as an array with a maximum capacity of 5 elements and O(1) insert and remove, and the following functions are performed: Insert(7), Insert(6), Insert(5), Insert(4), Remove(), Remove(), Insert(3), Insert(2), Insert(1), Remove() The removeIndex value will be index 4 The array will be [2][1][ ][4][3] The insertIndex value will be index 2 Given a priority queue implemented as a standard linked list with only a…arrow_forwardStep 1: Inspect the Node.java file Inspect the class declaration for a doubly-linked list node in Node.java. Access Node.java by clicking on the orange arrow next to LabProgram.java at the top of the coding window. The Node class has three fields: a double data value, a reference to the next node, and a reference to the previous node. Each field is protected. So code outside of the class must use the provided getter and setter methods to get or set a field. Node.java is read only, since no changes are required. Step 2: Implement the insert() method A class for a sorted, doubly-linked list is declared in SortedNumberList.java. Implement the SortedNumberList class's insert() method. The method must create a new node with the parameter value, then insert the node into the proper sorted position in the linked list. Ex: Suppose a SortedNumberList's current list is 23 → 47.25 → 86, then insert(33.5) is called. A new node with data value 33.5 is created and inserted between 23 and 47.25,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
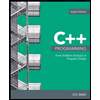
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning