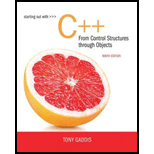
Starting Out with C++ from Control Structures to Objects Plus MyLab Programming with Pearson eText -- Access Card Package (9th Edition)
9th Edition
ISBN: 9780134544847
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 1PC
Program Plan Intro
Linked List Operations
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, destructor, and member functions in the class.
“IntList.cpp”:
- Include the required header files into the program.
- Define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list is empty or not, if the “head” is empty then make a new node into “head” pointer. Otherwise, make a loop to find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Define a function named “display()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Define a function named “insertNode()” to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Use “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Define a function named “deleteNode()” to delete a value from the list.
- Declare the structure pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Use “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Define the destructor to destroy the list values from the memory.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
“Main.cpp”:
- Include the required header files into the program.
- Declare an object named “obj” for the class “IntList”.
- Make a call to functions for insert, append, and delete operations.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Create a function that takes the first Node in a linked list as an input, reverses the list (destructively), and then returns the original Node.
Part2: LinkedList implementation
1. Create a linked list of type String Not Object and name it as StudentName.
2. Add the following values to linked list above Jack Omar Jason.
3. Use addFirst to add the student Mary.
4. Use addLast to add the student Emily.
5. Print linked list using System.out.println().
6. Print the size of the linked list.
7. Use removeLast.
8. Print the linked list using for loop for (String anobject: StudentName){…..}
9. Print the linked list using Iterator class.
10. Does linked list hava capacity function?
11. Create another linked list of type String Not Object and name it as TransferStudent.
12. Add the following values to TransferStudent linked list Sara Walter.
13. Add the content of linked list TransferStudent to the end of the linked list StudentName
14. Print StudentName.
15. Print TransferStudent.
16. What is the shortcoming of using Linked List?
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects Plus MyLab Programming with Pearson eText -- Access Card Package (9th Edition)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- True or FalseA Doubly Linked List has a Header and Trailer sentinels to facilitate a more generic approach when adding and removing the head and tail nodes.arrow_forwardCreate a ToArray function for the LinkedList class that returns an array from a linked list instance.arrow_forwardWrite appropriate comments for the following partial code. Mention, the relation of linked list with the partial code.Partial Code: typedef struct node_s { char current[3]; int volts; struct node_s *linkp; } node_t;arrow_forward
- Think about the following example: A computer program builds and modifies a linked list like follows:Normally, the program would keep tabs on two unique nodes, which are as follows: An explanation of how to use the null reference in the linked list's node in two common circumstancesarrow_forwardTRUE or FALSE? Answer the following question and state the reason why: The delete operation only involves the removing of the node from the list without breaking the links created by the next node. You need an array to represent each node in a linked list. STL lists are also efficient at adding elements at their back because they have a built-in pointer to the last element in the list. A circular linked list has 2 node pointers. cout<<list.back()<<endl; = The back member function returns a reference to the last element in the list. In a Dynamic Stack, the pointer top stays at the head after a push operation. During a Pop operation in Static Stack, the elements are being moved one step up. In a dynamic implementation of stack, the pointer top has an initial value of null. In a dynamic stack, the node that was popped is deleted. In a dynamic stack, the pointer top stays at the head after push operation. STL function top returns a reference to element at the top of the…arrow_forwardIn c++ , write a program to create a structure of a node, create a class Linked List. Implement all operations of a linked list as member function of this class. • create_node(int); • insert_begin(); • insert_pos(); • insert_last(); • delete_pos(); • sort(); • search(); • update(); • reverse(); • display(); ( Drop coding in words with screenshot of output as well )arrow_forward
- True or False? When implementing a queue with a linked list, the front of the queue is also the front of the linked list.arrow_forwardint F(node<int>&p){int c=0; while(p!=0){p=p->next; c++; } return c;} This function is a. return the number of items in the linked list b. return the number of items in a linked list and destroy the linked list c. None of these d. destroy the list and free all allocated nodesarrow_forwardLinked list. Complete the function that takes as a parameter the head of a linked list and prints the linked list in reverse order. If the linked list had the contents: of,the,and,on,a,an,ok. Then the correct output would be: ok,an,a,on,and,the,of. Given: struct node { char word[31]; struct node *prev, next; }; void print_reverse(struct node *head) { }arrow_forward
- What does the following function do for a given Linked List? void fun1(struct node* head){if(head == NULL)return;fun1(head->next);printf("%d ", head->data);}arrow_forwardFunctions that look at a linked list but do not modify it are referred to as______________arrow_forwardWrite your own functions to create, insert nodes (both from the front and the end of the list), delete nodes, count the number of nodes, display all the nodes, display a specific node for the linked list. C++ and Javaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
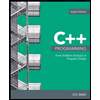
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning