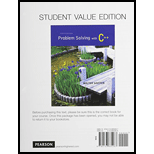
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
9th Edition
ISBN: 9780133862225
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 5PP
Program Plan Intro
Intersection of two sets
Program Plan:
- Include required header file.
- Define main function.
- Declare variable “R” in “set” template class of type “string”.
- Declare iterator variable “iter” in “set” template class of type “string”.
- Initializes the string for set “Q” and set “D”.
- Declare the iterator variable “cIterator” for storing result of intersection of two sets “Q” and “D”.
- Compute the intersection of two sets by using the generic function “set_intersection” function.
- Display the string in resultant string after intersecting two sets.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Add a new public member function to the LinkedList class named reverse() which reverses the items in the list. You must take advantage of the doubly-linked list structure to do this efficiently as discussed in the videos/pdfs, i.e. swap each node’s prev/next pointers, and finally swap headPtr/tailPtr. Demonstrate your function works by creating a sample list of a few entries in main(), printing out the contents of the list, reversing the list, and then printing out the contents of the list again to show that the list has been reversed.
Note: your function must actually reverse the items in the doubly-linked list, not just print them out in reverse order!
Note: we won't use the copy constructor in this assignment, and as such you aren't required to update the copy constructor to work with a doubly-linked list.
This is what I have so far but its not working!
template<class ItemType>void LinkedList<ItemType>::reverse(){ Node<ItemType>*curPtr,*prev,*next;…
The implementation for the priority queue in this chapter starts by modifying theHeap class so that the order of the elements can be determined by the programmer.Earlier the heap kept the highest value on the top of the container, but now it willbe altered to allow for either the highest or the lowest. The changes made to thisclass involve specifying another template parameter, which will be used for thecomparison operator. Altering the push() and pop() functions to make use of thecomparison operator instead of assuming that the largest value element will be ontop.
use c++ to code.
In this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not impact the overall…
Chapter 18 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Ch. 18.1 - If v is a vector, what does v.begin() return? What...Ch. 18.1 - If p is an iterator for a vector object v, what is...Ch. 18.1 - Suppose v is a vector of ints. Write a for loop...Ch. 18.1 - Suppose the vector v contains the letters 'A',...Ch. 18.1 - Suppose the vector v contains the letters 'A',...Ch. 18.1 - Suppose you want to run the following code, where...Ch. 18.2 - Prob. 7STECh. 18.2 - Prob. 8STECh. 18.2 - Prob. 9STECh. 18.2 - Prob. 10STE
Ch. 18.2 - Prob. 11STECh. 18.2 - Prob. 12STECh. 18.2 - Prob. 13STECh. 18.2 - Prob. 14STECh. 18.2 - Prob. 15STECh. 18.2 - Prob. 16STECh. 18.3 - Prob. 17STECh. 18.3 - Prob. 18STECh. 18.3 - Prob. 19STECh. 18.3 - Suppose v is an object of the class vectorint. Use...Ch. 18.3 - Prob. 21STECh. 18.3 - Can you use the copy template function with vector...Ch. 18.3 - Prob. 23STECh. 18 - Prob. 1PCh. 18 - Prob. 2PCh. 18 - Prob. 3PCh. 18 - Prob. 4PCh. 18 - Write a program that allows the user to enter any...Ch. 18 - Prob. 3PPCh. 18 - Prob. 5PPCh. 18 - Solution to Programming Project 18.6 In this...Ch. 18 - Prob. 7PPCh. 18 - You have collected a file of movie ratings where...Ch. 18 - Prob. 9PP
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardWrite a function that gets a linked list of ints, and reverses it. For example - on input 1 -> 2 -> 3 -> 4, after the function finishes the execution, the list becomes 4 -> 3 -> 2 -> 1 - If the list has one element, then it doesn’t change - If the list is empty, then it doesn’t change You may use the data fields in the struct and the functions provided in LL.h and LL.c. // reverses a linked list void LL_reverse(LL_t* list); Test for the Function: void test_q3() { LL_t* lst = LLcreate(); LL_add_to_tail(lst, 1); LL_add_to_tail(lst, 3); LL_add_to_tail(lst, 8); LL_add_to_tail(lst, 4); LL_add_to_tail(lst, 3); LL_reverse(lst); intcorrect[] = {3,4,8,3,1}; inti; node_t* n = lst->head; for(i=0;i<5;i++) { if (n==NULL) { printf("Q3 ERROR: node %d==NULL unexpected\n", i); return; } if (n->data != correct[i]) { printf("Q3 ERROR: node%d->data==%d, expected %d\n", i, n->data, correct[i]); return; } n = n->next; } if (n==NULL) printf("Q3 ok\n"); } Support…arrow_forward
- Write a function that gets a linked list of ints, and reverses it. For example - on input 1 -> 2 -> 3 -> 4, after the function finishes the execution, the list becomes 4 -> 3 -> 2 -> 1 - If the list has one element, then it doesn’t change - If the list is empty, then it doesn’t change You may use the data fields in the struct and the functions provided in LL.h and LL.c. // reverses a linked list void LL_reverse(LL_t* list); Test for the Function; void test_q3() { LL_t* lst = LLcreate(); LL_add_to_tail(lst, 1); LL_add_to_tail(lst, 3); LL_add_to_tail(lst, 8); LL_add_to_tail(lst, 4); LL_add_to_tail(lst, 3); LL_reverse(lst); intcorrect[] = {3,4,8,3,1}; inti; node_t* n = lst->head; for(i=0;i<5;i++) { if (n==NULL) { printf("Q3 ERROR: node %d==NULL unexpected\n", i); return; } if (n->data != correct[i]) { printf("Q3 ERROR: node%d->data==%d, expected %d\n", i, n->data, correct[i]); return; } n = n->next; } if (n==NULL) printf("Q3 ok\n"); } Support File…arrow_forwardI ASK THIS QUESTION HERE AND YOUR EXPERT SOLVE IT THIS WAY BUT CAN YOU WRITE THE main ( ) FOR THIS CODE Consider the following implementation of the node and doubly linked-list: template <class type>class node{public:type info;node<type> * next;// nextnode<type> * prev;//back }; template <class type>class doubly_linked_list{//data membersprivate:node<type> *head, *tail;int length;public:doubly_linked_list(){head = tail = NULL;length = 0;}bool isEmpty(){ // return (head==NULL);if (head == NULL)return true;elsereturn false;} void Append(type e){node<type> *newnode = new node<type>;newnode->info = e;if (isEmpty()){newnode->next = NULL;newnode->prev = NULL;head = newnode;tail = newnode;}else{tail->next = newnode;newnode->prev = tail;newnode->next = NULL;tail = newnode;}++length; } void Display(){if (isEmpty()) { cout << "The linked list is empty !!!!"; return; }cout << "list elements: ";node<type> *…arrow_forwardWrite a function called mergeLists that takes two call-by-reference argu- ments that are pointer variables that point to the heads of linked lists of values of type int. The two linked lists are assumed to be sorted so that the number at the head is the smallest number, the number in the next node is the next smallest, and so forth. The function returns a pointer to the head of a new linked list that contains all of the nodes in the original two lists. The nodes in this longer list are also sorted from smallest to largest values. Note that your function will neither create nor destroy any nodes. When the function call ends, the two pointer variable arguments should have the value NULL.arrow_forward
- What are the properties of sets, lists, vectors, strings and are they mutable or immutable? What type of problems is each one best used for over the other type? E.g if I have a function that solves a problem using a list, why wouldn't I be able to use a vector or set for it? Can I have some code that shows this in Racket?arrow_forwardWrite a void function that takes a linked list of integers and removes all the duplicate elements from the list. The function will have one call-by-reference parameter that is a pointer to the head of the list. After the function is called, this pointer will point to the head of a linked list that has the nodes that have all the distinct integers. Place your function in a suitable test program.arrow_forwardImplements clone which duplicates a list. Pay attention, because if there are sublists, they must be duplicated as well. Understand the implementation of the following function, which recursively displays the ids of each node in a list def show_ids(M, level=0): k = M.first_node while k is not None: print(" "*2*level, id(k)) if (str(k.value.__class__.__name__) == str(M.__class__.__name__)): show_ids(k.value, level+1) k = k.next W=L2(Node(10, Node(L2(Node(14, Node(15, Node(L2(Node(16, Node(17))))))), Node(20, Node(30)))) )show_ids(W) Develop your solution as follows: - First copy the nodes of the current list (self) - Create a new list with the copied nodes - Loop through the nodes of the new list checking the value field - If this field is also a list (use isinstance as in the show_ids function) then it calls clone on that list and substitutes the value. Complete the code: def L4(*args,**kwargs): class L4_class(L):…arrow_forward
- Suppose we have class named NumberList that holds a linked list of double values. In this section you will modify the class by adding recursive member functions. The functions will use recursion to traverse the linked list and perform the following operations: • Count the number of nodes in the list. To count the number of nodes in the list by recursion, we introduce two new member functions: numNodes and countNodes. countNodes is a private member function that uses recursion, and numNodes is the public interface that calls it. • Display the value of the list nodes in reverse order. To display the nodes in the list in reverse order, we introduce two new member functions: displayBackwards and showReverse. showReverse is a private member function that uses recursion, and displayBackwards is the public interface that calls it.arrow_forwardFor this assignment, you need to implement link-based List and derivative ADTs in Java. To complete this, you will need the following: A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1. A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private. The attribute names for the Node and Linked List are the words in bold in #1 and #2. For the Linked List, implement the most common linked-list behaviors as explained in class - getters/setters/constructors/destructors for the attributes of the class, (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the…arrow_forwardImplement a CircularArray class that supports an array-like data structure whichcan be efficiently rotated. If possible, the class should use a generic type (also called a template), and should support iteration via the standard for (Obj o : circularArray) notation.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
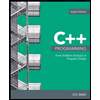
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning