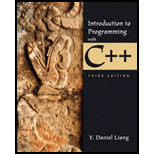
Introduction to Programming with C++
3rd Edition
ISBN: 9780133252811
Author: Y. Daniel Liang
Publisher: Prentice Hall
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 2, Problem 8CP
Translate the following
Step 1: Declare a double variable named miles with initial value 100.
Step 2: Declare a double constant named KILOMETERS PER MILE with value 1.609.
Step 3: Declare a double variable named kilometers, multiply miles and KILOMETERS_PER_MILE, and assign the result to kilometers.
Step 4: Display kilometers to the console.
What is kilometers after Step 4?
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(C PROGRAMMING ONLY)
6. Deal or No Deal?by CodeChum Admin
When I was a kid, I used to watch this TV game show, Deal or No Deal? In the game, there are different brief cases, each labeled with a number. Inside each brief case is an amount in Pesos and the goal is to guess which briefcase contains the smallest amount.
In this program, instead of just finding the smallest amount, your task is to ask the user for an integer input and print the values in the array smaller than the inputted integer.
Instructions:
In the code editor, you are provided with an array with 100 elements.Your task is to ask the user for an integer input and then print all the elements in the array whose value is lesser than the inputted integer.Input
1. Inputted integer
Output
Enter value: 6132450351503022244
Two number systems, octal (base 8) and hexadecimal (base 16), are of interest to computer scientists. In fact, in C++, you can instruct the computer to store a number in octal or hexadecimal.
The digits in the octal number system are 0,1,2,3,4,5,6, and 7. The digits in hexadecimal number systems are 0,1,2,3,4,5,6,7,8,9,A,B,C,D,E, and F. So A in hexadecimal is 10 in decimal, B is 11 in decimal, and so on.
The algorithm to convert a positive decimal number into an equivalent number in an octal (or hexadecimal) is the same for binary numbers. Here, we divide the decimal number by 8(for octal) and by 16(for hexadecimal). Suppose ab represents the number a to the base b. For example, 7510 means 75 to the base 10(that is decimal), and 8316 means 83 to the base 16(that is hexadecimal).
Then 75310 =13618 and 75310= 2F116*
Write a program that uses a recursive function to convert a number in decimal to base 8 or base 16.
Two number systems, octal (base 8) and hexadecimal (base 16), are of interest to computer scientists. In fact, in C++, you can instruct the computer to store a number in octal or hexadecimal.
The digits in the octal number system are 0,1,2,3,4,5,6, and 7. The digits in hexadecimal number systems are 0,1,2,3,4,5,6,7,8,9,A,B,C,D,E, and F. So A in hexadecimal is 10 in decimal, B is 11 in decimal, and so on.
The algorithm to convert a positive decimal number into an equivalent number in an octal (or hexadecimal) is the same for binary numbers. Here, we divide the decimal number by 8(for octal) and by 16(for hexadecimal). Suppose ab represents the number a to the base b. For example, 7510 means 75 to the base 10(that is decimal), and 8316 means 83 to the base 16(that is hexadecimal).
Then 75310 =13618 and 75310= 2F116*
Write a program that uses a recursive function to convert a number in decimal to base 8 or base 16.It is necessary that the user enters a nonnegative integer. Check…
Chapter 2 Solutions
Introduction to Programming with C++
Ch. 2 - Show the output of the following code:
Ch. 2 - How do you write the statements to let the user...Ch. 2 - Prob. 3CPCh. 2 - Which of the following identifiers are valid?...Ch. 2 - Identify and fix the errors in the following...Ch. 2 - Prob. 6CPCh. 2 - Prob. 7CPCh. 2 - Translate the following algorithm into code:
Step...Ch. 2 - Prob. 9CPCh. 2 - Prob. 10CP
Ch. 2 - Prob. 11CPCh. 2 - Prob. 12CPCh. 2 - Prob. 13CPCh. 2 - Prob. 14CPCh. 2 - Prob. 15CPCh. 2 - Are the following statements correct? If so, show...Ch. 2 - Write a statement to display the result of 23.5.Ch. 2 - Suppose m and r are integers. Write a C++...Ch. 2 - How would you write the following arithmetic...Ch. 2 - Prob. 20CPCh. 2 - Prob. 21CPCh. 2 - Which of the following statements are true?
a....Ch. 2 - Show the printout of the following code:
Ch. 2 - Prob. 24CPCh. 2 - Prob. 25CPCh. 2 - Prob. 26CPCh. 2 - Show the following output;
Ch. 2 - If you change in line 12 in Listing 2.10, what...Ch. 2 - Show the printout of the following code:
Ch. 2 - How would you write the following arithmetic...Ch. 2 - (Convert Celsius to Fahrenheit) Write a program...Ch. 2 - (Compute the volume of a cylinder) Write a program...Ch. 2 - (Convert feet into meters) Write a program that...Ch. 2 - (Convert pounds into kilograms) Write a program...Ch. 2 - (Financial application: calculate tips) Write a...Ch. 2 - (Sum the digits in an integer) Write a program...Ch. 2 - (Find the number of years) Write a program that...Ch. 2 - (Current time) Listing 2.9, ShowCurrentTime.cpp,...Ch. 2 - (Physics: acceleration) Average acceleration is...Ch. 2 - (Science: calculating energy) Write a program that...Ch. 2 - (Population projection) Rewrite Programming...Ch. 2 - (Physics: finding runway length) Given an...Ch. 2 - (Financial application: compound value) Suppose...Ch. 2 - (Health application: BMP) Body Mass Index (BMI) is...Ch. 2 - (Geometry: distance of two points) Write a program...Ch. 2 - (Geometry: area of a hexagon) Write a program that...Ch. 2 - (Science: wind-chill temperature) How cold is it...Ch. 2 - (Print a table) Write a program that displays the...Ch. 2 - (Geometry: area of a triangle) Write a program...Ch. 2 - (Slope of a line) Write a program that prompts the...Ch. 2 - (Cost of driving) Write a program that prompts the...Ch. 2 - (Financial application: calculate interest) If you...Ch. 2 - (Financial application: future investment value)...Ch. 2 - Prob. 24PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Data processing) Years that are evenly divisible by 400 or are evenly divisible by 4 but not by 100 are leap years. For example, because 1600 is evenly divisible by 400, 1600 was a leap year. Similarly, because 1988 is evenly divisible by 4 but not by 100, it was also a leap year. Using this information, write a C++ program that accepts the year as user input, determines whether the year is a leap year, and displays a message telling the user whether the entered year is or is not a leap year.arrow_forwardIn this chapter, you learned that although a double and a decimal both hold floating-point numbers, a double can hold a larger value. Write a C# program named DoubleDecimalTest that declares and displays two variables-a double and a decimal. Experiment by assigning the same constant value to each variable so that the assignment to the double is legal but the assignment to the decimal is not. In other words, when you leave the decimal assignment statement in the program, an error message should be generated that indicates the value is outside the range of the type decimal, but when you comment out the decimal assignment and its output statement, the program should compile correctly.arrow_forwardPlease written by computer source 1, 2 C++ Programming. Please answer ASAP. Thank you very much. Question 1: Write a C++ program that reads two marks (a mark for mathematic course and another mark for physics course) and the weighting of the mark of mathematics compared to that of physics. The program then displays the weighted average with two obligatory decimals. Below is the example of how the program should look like. Enter average in math 80.25 Enter the average in physics 100 Enter the weight assigned to mathematics 0.4 The weighted average is then 92.10% 2) Write a C++ program that asks for the different prices for a bus ticket (adult, child, senior). Then, the program asks for the number of adults, elders, and children in the group. The program then displays the total ordered without taxes. Then the program displays the amount of the provincial tax (9.975% of the total Canadian price without tax) and federal tax (5% of the Canadian price total without tax) as well as the total…arrow_forward
- NEED CODE IN C++ ON VISUAL STUDIO AND OUTPUT QUESTION The following table shows telephone area codes in Pakistan Area Code Major City 21 Karachi 42 Lahore 61 Multan 442 Okara 51 Islamabad Write a C++ program with the help of switch statement that input the city area code and prints the corresponding city name.arrow_forwarda. Write a c program that asks a user for an integer. Declare a variable num3. Let num3 = --x; Display the values of x, and num3.b. Write a c program that asks a user for an integer. Declare a variable num4. Let num4 = x--; Display the values of x, and num4.arrow_forwardThe problem is from Introduction to Programming and Data Structures with C++, 4th edition (Count positive and negative numbers and compute the average of numbers)Write a program that reads an unspecified number of integers, determines how many positive and negative values have been read, and computes the total and average of the input values (not counting zeros). Your program ends with the input 0. Display the average as a floating-point number.If you entire input is 0, display 'No numbers are entered except 0.'Sample Run 1Enter an integer, the input ends if it is 0: 1 2 -1 3 0The number of positives is 3The number of negatives is 1The total is 5The average is 1.25Sample Run 2Enter an integer, the input ends if it is 0: 0No numbers are entered except 0arrow_forward
- Question 2: Write a C Program A bank wants a program that determines the yearly interest earned on a guaranteed investment certificate (GIC), given a number of years. If the investment is for five years, the interest is 8.2% per year; if it is for one year, the interest is 5%. Print on the screen "Invalid data. Please try again later.", if the user enters any value other than 1 or 5 for the number of years of investment. Write a complete C program to determine the amount of interest earned per year Hint: Interest - amount * percentagearrow_forwardThe code box below shows a simple C++ program. The program declares and initializes three integers. Then it computes the average of these integers and displays the result. However, there are some mistakes in the code. Find and fix these mistakes such that the code will display the exact output below. Please note that the names of the variables and constants are the same as those in the code in the image and adhere to them accurately. The reason is that the program corrects the code automatically and it must be identicalarrow_forwardC++ Programming Exercise: A real estate office handles, say, 50 apartment units. When the rent is, say, $600 per month, all the units are occupied. However, for each, say, $40 increase in rent, one unit becomes vacant. Moreover, each occupied unit requires an average of $27 per month for maintenance. How many units should be rented to maximize the profit? Write a program that prompts the user to enter: a. The total number of of units. b. The rent to occupy all the units. c. The increase in rent that results in a vacant unit. d. Amount to maintain a rented unit. The program then outputs the number of units to be rented to maximize the profit.arrow_forward
- Write a C++ program called AlphaBravoCharlie which prints the numbers 1 to 110, 11 numbers perline. The program shall print "Alpha" in place of the numbers which are multiples of 3, "Bravo"for multiples of 5, "Charlie" for multiples of 7, "AlphaBravo" for multiples of 3 and 5, and so on. this is complete question answer should be in c++ language.arrow_forwardWrite C program which should print your name and registration number on the screen then do the following:an industry has launched an online shopping ordering system which sells 5 different products. A list of productnumber, product name and their retail price is given below:Product No. Product Name Retail Price in Rs. Quantity Available101 Soap 60.00 10102 Liquid Hand Wash 200.00 20103 Liquid Dish Wash 150.00 30104 Table cleaner 350.00 40105 Hand Sanitizer 100.00 50 Your program must store this information in an array of struct product type. You must declare the struct product_type. (HINT: You can make any other structures or arrays of structures that you think might help you solve this problem.) Your program should…arrow_forwardIn C++, Write a program that asks the user to enter two numbers, obtains the two numbers from the user and prints the sum, product, difference, and quotient of the two numbers.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
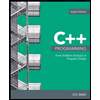
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
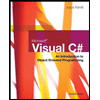
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
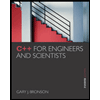
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Algebraic Expressions – Algebra Basics; Author: TabletClass Math;https://www.youtube.com/watch?v=U-7nq7OG18s;License: Standard YouTube License, CC-BY
Python Tutorial for Beginners 3 - Basic Math, Mathematical Operators and Python Expressions; Author: ProgrammingKnowledge;https://www.youtube.com/watch?v=Os4gZUI1ZlM;License: Standard Youtube License