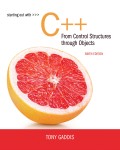
EBK STARTING OUT WITH C++ FROM CONTROL
9th Edition
ISBN: 8220106714379
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 4PC
Program Plan Intro
Height of the Binary Tree
Program Plan:
- Create a template prefix and define the template class BinaryTree to perform the following functions:
- Declare the required variables.
- Declare the function prototypes.
- Define the no-argument generic constructor BinaryTree() to initialize the root value as null.
- Call the functions insertNode(), remove(), displayInOrder(), and treeHeight().
- Define the generic function insert() to insert the node in position pointed by the tree node pointer in a tree.
- Define the generic function insertNode() to create a new node and it should be passed inside the insert() function to insert a new node into the tree.
- Define the generic function remove()which calls deleteNode() to delete the node.
- Define the generic function deleteNode() which deletes the node stored in the variable num and it calls the makeDeletion() function to delete a particular node passed inside the argument.
- Define the generic function makeDeletion()which takes the reference to a pointer to delete the node and the brances of the tree corresponding below the node are reattached.
- Define the generic function displayInOrder()to display the values in the subtree pointed by the node pointer.
- Define the generic function getTreeHeight() to count the height of the tree.
- Define the generic function TreeHeight()which calls getTreeHeight() to display the height of the tree.
- In main() function,
- Create a tree with integer data type to hold the integer values.
- Call the function treeHeight() to print the initial height of the tree.
- Call the function insertNode() to insert the node in a tree.
- Call the function displayInOrder() to display the nodes inserted in the order.
- Call the function remove() to remove the nodes from tree.
- Call the function treeHeight() to print the height of the tree after deleting the nodes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
#include <stdio.h>#include <stdlib.h>#include <time.h>
struct treeNode { struct treeNode* leftPtr; int data; struct treeNode* rightPtr;};
typedef struct treeNode TreeNode;typedef TreeNode* TreeNodePtr;
void insertNode(TreeNodePtr* treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr);
int main(void) { TreeNodePtr rootPtr = NULL;
srand(time(NULL)); puts("The numbers being placed in the tree are:");
for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); }
puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr);
puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr);
puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);}
void insertNode(TreeNodePtr* treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode));
if…
#include <stdio.h>#include <stdlib.h>#include <time.h>
struct treeNode { struct treeNode *leftPtr; int data; struct treeNode *rightPtr;};
typedef struct treeNode TreeNode;typedef TreeNode *TreeNodePtr;
void insertNode(TreeNodePtr *treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr);
int main(void) { TreeNodePtr rootPtr = NULL;
srand(time(NULL)); puts("The numbers being placed in the tree are:");
for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); }
puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr);
puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr);
puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);}
void insertNode(TreeNodePtr *treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode));
if (*treePtr != NULL) { (*treePtr)->data = value;…
A "generic" data structure cannot use a primitive type as its generic type.
O True
False
Chapter 21 Solutions
EBK STARTING OUT WITH C++ FROM CONTROL
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- ArcGis Program Geographic Systems You have been assigned to create a map using a number of feature classes. Each feature class is projected in a different coordinate system. The accurate position of all the features is very important for this assignment. Therefore, you select a feature class and use it's coordinate system for the data frame. Then you should perform a(n) for each of the remaining feature classes.arrow_forwardTask 4. Utility classes Implement a Menu-Driven program of ArrayList class using the above scenario and perform the following operations. Create an object of ArrayList class based on the scenario with explanations of the method Create a method that will allow inserting an element to ArrayList Class based on the scenario. The input field must be validated as Create a method to delete specific elements in the ArrayList with explanations of the methodarrow_forwardCourse: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: Create a class Node having two data members int data; Node next; Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null Create class LinkList having one data members of type Node. Node head Write the following function in the LinkList class publicvoidinsertAtLast(int data);//this function add node at the end of the list publicvoid insertAthead(int data);//this function add node at the head of the list publicvoid deleteNode(int key);//this function find a node containing "key" and delete it publicvoid printLinkList();//this function print all the values in the Linklist public LinkListmergeList(LinkList l1,LinkList l2);// this function…arrow_forward
- T or F -Functions are considered to be objects in JavaScript because they contain three critical attributes: data, methods that act on the data, parameters for passing the data also known as awareness. -A recursive function is a function that calls itself and eventually returns default value to break out of the recursion. -A function that is used to create a new object is called an anonymous function. -Attributes define additional characteristics or properties of the element which is the same as for the start tag. -Javascript is similar to the Java programming languagearrow_forwardUnordered Sets |As explained in this chapter, a set is a collection of distinct elements of the same type. Design the class unorderedSetType, derived from the class unorderedArrayListType, to manipulate sets. Note that you need to redefine only the functions insertAt, insertEnd, and replaceAt. If the item to be inserted is already in the list, the functions insertAt and insertEnd output an appropriate message, such as 13 is already in the set. Similarly, if the item to be replaced is already in the list, the function replaceAt outputs an appropriate message. Also, write a program to test your class.arrow_forwardData structures find_color(colors:set, values:list) -> list The function will have two parameters. The first parameter is a set of strings known as Colors. A second parameter is a list of tuple-2 known as Values. Colors will contain a set of randomly selected colors. Values will contain a list of tuples of size 2. Each tuple will contain color (str) and a number (int). The function should look at each tuple in Values. For each tuple, add the number (the second value in the tuple) to a list of numbers if the color in the tuple (the first value in the tuple) is in Colors. In other words, find all tuples that have a color in the Colors and add the tuples numbers to a list. Finally, the function should return the list of numbers collected in the order they are found in the values list. Example: Colors: {'black', 'pink', 'yellow'} values: [('green', 100), ('yellow', 13), ('red', 6)] Expected: [13] Colors: {'yellow'} values: [('black', 54), ('pink', 5)] Expected: [] Colors: {'black',…arrow_forward
- Define the function number_in_trophic_class(tli3_values, classification) which takes a list of trophic level index values and a trophic classification, returning the number of lakes in that trophic class. For this version of the question you cannot use a list comprehension (or any comprehension) - you will get to use one in the next version of the question. :) Notes: You must include, and use/call your trophic_class function in your submission. Your tropic_class function must work in the same way it did for Question 2. You can assume that classification will always be valid, i.e. it will always be one of the states in the following list: ['Hypertrophic', 'Supertrophic', 'Eutrophic', 'Mesotrophic', 'Oligotrophic', 'Microtrophic', 'Ultra-microtrophic'] For example: Test Result tli3_list_1 = [4.1061, 2.54561, 4.16276, 2.33801, 6.71792, 5.54457, 6.49795, 2.1, 1.2, 1.4, 0.9, 3.8, 3.0] number = number_in_trophic_class(tli3_list_1, 'Microtrophic') print(f'{number} were…arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forwardExercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forward
- #include<stdio.h> #include<stdlib.h> #include<conio.h> struct nodetype { struct nodetype *left ; int info ; struct nodetype *right; }; typedef struct nodetype *NODEPTR; NODEPTR maketree(int); NODEPTR getnode(void); void inordtrav(NODEPTR); int main(void) { NODEPTR root , p , q; printf("%s\n","Enter First number"); scanf("%d",&number); root=maketree(number); /* insert first root item */ while(scanf("%d",&number) !=EOF) { p=q=root; /* find insertion point */ while((number !=p->info) && q!=NULL) {p=q; if (number <p->info) q = p->left; else q = p->right; } q=maketree(number); /* insertion */ if (number==p->info) printf("%d is a duplicate \n",number); else if (number<p->info)…arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the private section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used. #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const { eturn bf; }…arrow_forwardBasic javaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
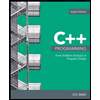
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning