Instructor Solutions Manual For Introduction To Java Programming And Data Structures, Comprehensive Version, 11th Edition
11th Edition
ISBN: 9780134671581
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 28, Problem 28.19PE
Program Plan Intro
Display a DFS/BFS tree in a graph
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Create a text field.
- Create the required buttons.
- Create the label.
- City values vertices gets assigned.
- City value edges get assigned.
- New graph gets created.
- New view gets defined
- Define the start method:
- Create a new border pane.
- New hbox gets created.
- Add the buttons into the pane.
- Set the alignment of the pane.
- New scene gets created
- Set the title of the scene.
- Scene gets placed on the stage.
- The stage gets displayed.
- Action event for the button defined is set.
- Based on the action defined a new tree is defined.
- Define the main method
- Initialize the calls.
- Class “GraphView” gets defined
- Assign the graph.
- Assign the tree
- Define the method “GraphView”
- Assign the graph.
- Define the method “setTree”
- Assign the tree
- Define the method “repaint()”
- Clear the pane.
- Loop that iterates to draw the vertices
- Get the position of x and y coordinates.
- Condition to validate the edge gets defined.
- Loop that iterates to draw the line.
- Define the method “drawArrowLine”
- New lines get created.
- Add the line color.
- Calculate the angle.
- Set the angle.
- Condition to validate the arrow point gets defined.
- Draw the arrow at the required positions.
- Define the class “city”
- Declare the required variables.
- Assign the x and y value.
- Get the name.
- Validate the city name that is entered.
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Displayable.java
- Define the interface.
- Get the x value
- Get the y value.
- Get the name.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Help in C++ please:
Write a program (in main.cpp) that:
Prompts the user for a filename containing node data.
Outputs the minimal spanning tree for a given graph.
You will need to implement the createSpanningGraph method in minimalSpanTreeType.h to create the graph and the weight matrix.
There are a few tabs:
main.cpp, graphType.h, linkedList.h, linkedQueue.h, queueADT.h, minimalSpanTreeType.h, and then two data files labeled: CH20_Ex21Data.txt, CH20Ex4Data.txt
C+
Write a program that outputs the shortest distance from a given node to every other node in the graph.
I NEED THESE HEADER FILES INCLUDED IN THE PROGRAM PLEASE
These are the header files: graphType.h, linkedList.h, linkedQueue.h, queueADT.h, testProgGraph.cpp, unorderedLinkedList.h, weightedGraph.h
Explain this code by putting comments in detail. Language used is c++. Topic is Linkedlist
// void deleteByData(int data) { // if(head->data == data) { // head = head->next; // } // next = head; // Node *prev = next; // while (next != NULL) { // if (next->data == data) { // prev->next = prev->next->next; // } // prev = next; // next = next->next; // } // };
};
Chapter 28 Solutions
Instructor Solutions Manual For Introduction To Java Programming And Data Structures, Comprehensive Version, 11th Edition
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ A program applying the topics involved in data structures (linked list, graph, array etc.) 1. User will be asked to login/signup 2. User will choose for the nearest restaurant (undirected graph?) 3. User will add to cart (from the menu list of the restaurant) 4. User will be asked for order confirmation and mode of payment (cash on delivery, card??) note: new users will have a discount 5. Invoice Using Dev c++arrow_forwardC++ Write a program that outputs the shortest distance from a given node to every other node in the graph.arrow_forwardplease complete the following in JAVA Implement the graph ADT using the adjacency list structure. thanks! also posting a similar question for adjacency matrix. have a good day!arrow_forward
- Write a program in c++ which should create a weighted graph of the values entered by the user andthen write functions that perform the following: 1. Depth first search of source and destination vertex2. Breadth first search of source and destination vertex3. Shortest path search of source and all destination vertices (Dijkstra’s algorithm)4. Shortest path search of source and destination vertex (Dijkstra’s algorithm) The program should present a menu of the options and should run until the user opts to quit.arrow_forwardGraph Theory: Graph theory in computer science uses a graphical matrix with nodes and edges to describe a data structure. These can be used for search trees, game theory, shortest path, and many other applications in math and computer science. An example of an application of a graph in computer science is the data structure used to hole the moves for a checkers game program. Each move can be represented by a node. The edges connecting the nodes are determined by the rules of the game, basically how to get to the node. This is a directed graph, because a player cannot take a move back. So the flow is always in one direction towards the end of the game. Cycles in a graph is when a node can go back to itself. This is not possible in this graph, because a move can only go to another position on the board. The only case where this would be correct is if a player were allowed to skip his turn, or move to the same spot that he is already in. A graph is said to be connected if there is a path…arrow_forwardBreak out the restrictions placed on the stack and its unrestricted counterpart.arrow_forward
- Study the scenario and comple the question(s) thatb follows: Convert Strings to Pig Latin using a linked list PigLatinStrings converts a string into a Pig Latin, but it processes only one word. If a word ends with a panctuation mark in the Pig Latin form,put the punctuation at the end of the string. For example: "Hello!" becomes "ello-Hay!" Write a program that prompts the user to input a string and then outputs the string in Pig Latin. Create the program using a Linked List(C++). a. Build a linked list as template either forwards or backwards.b. The linked list should have a function that prints the linked list. This function should be a recursive function.c. Your program must store the characters of a string into a linked list and use the function Rotate to remove the first node of the linked list and put it at the end of the linked list.arrow_forwardProvide full C++ code for main.cpp, playlist.h and playlist.cpp Building a playlist (of songs) using a linked list and making some operations such as adding songs, removing songs, shuffling them, etc. (Some parts of this lab will closely follow lab 08 - it is imperative that you complete that lab before working on this project). A Node representing a song has the following data members (similar to struct Node of lab 08, except it doesn't have one member for storing data): string uniqueID string songName string artistName int songLength Node* nextNodePtr Your code must have the following three files: Playlist.h - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members: private:Node* first;Node* last;int size; Playlist.cpp - Class definition main.cpp - main() function Checkpoint A For checkpoint A, you need to build a playlist (of songs) by readings data members of several songs…arrow_forward*Please using JAVA only* Objective Program 3: Binary Search Tree Program The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists. Program Description In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose…arrow_forward
- unique please Your task for this assignment is to identify a spanning tree in one connected undirected weighted graph using C++. Implement a spanning tree algorithm using C++. A spanning tree is a subset of the edges of a connected undirected weighted graph that connects all the vertices together, without any cycles. The program is interactive. Graph edges with respective weights (i.e., v1 v2 w) are entered at the command line and results are displayed on the console. Each input transaction represents an undirected edge of a connected weighted graph. The edge consists of two unequal non-negative integers in the range 0 to 9 representing graph vertices that the edge connects. Each edge has an assigned weight. The edge weight is a positive integer in the range 1 to 99. The three integers on each input transaction are separated by space. An input transaction containing the string “end-of-file” signals the end of the graph edge input. After the edge information is read, the process…arrow_forwardgive me a C++ code for the following prompt and explain each step and what it does. The image below is the head of sorted linked lists. Create the code that will delete any repeted/duplicates so each element appears only once. Also Return the linked list sorted as well.arrow_forwardLinked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should haveo Variables you may need for a Node,o (optional) Constructor Your linked list is of an int type. (you may do it as General type as <E>) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods,o Create a 2 linked list and test all your methods. (Including the compare)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
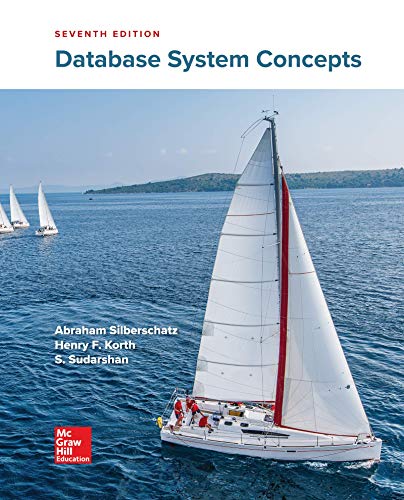
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
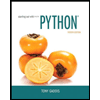
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
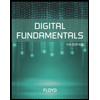
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
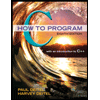
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
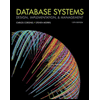
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
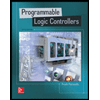
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education