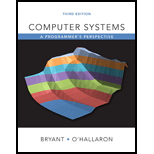
Computer Systems: A Programmer's Perspective (3rd Edition)
3rd Edition
ISBN: 9780134092669
Author: Bryant, Randal E. Bryant, David R. O'Hallaron, David R., Randal E.; O'Hallaron, Bryant/O'hallaron
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 3.60HW
A.
Explanation of Solution
Registers for holding values:
- The parameter “x” is been passed to function in register “%rdi”.
- The parameter “n” is been passed to function in register “%esi”.
- The register “%rax” is initialized to variable “result”...
B.
Explanation of Solution
Initial values of result and mask:
- The instruction “movl $1, %edx” sets value of “mask” to 1...
C.
Explanation of Solution
Test condition for mask:
- The instruction “testq %rdx, %rdx” denotes test condition for “mask”...
D.
Explanation of Solution
Condition to update mask:
- The instruction “salq %cl, %rdx” performs left shift on “mask”...
E.
Explanation of Solution
Condition to update result:
- The instruction “orq %r8, %rax” performs “OR” operation on “result”...
F.
Explanation of Solution
Given assembly code:
long loop(long x, int n)
x in %rdi, n in %esi
loop:
movl %esi, %ecx
movl $1, %edx
movl $0, %eax
jmp .L2
.L3:
movq %rdi, %r8
andq %rdx, %r8
orq %r8, %rax
`salq %cl, %rdx
.L2:
testq %rdx, %rdx
jne .L3
Rep; ret
Load Effective Address:
- The load effective address instruction “leaq” is a variant of “movq” instruction.
- The instruction form reads memory to a register, but memory is not been referenced at all.
- The first operand of instruction is a memory reference; the effective address is been copied to destination.
- The pointers could be generated for later references of memory.
- The common arithmetic operations could be described compactly using this instruction.
- The operand in destination should be a register.
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Comparison Instruction:
- The “CMP” instruction sets condition code according to differences of their two operands.
- The working pattern is same as “SUB” instruction but it sets condition code without updating destinations.
- The zero flag is been set if two operands are equal.
- The ordering relations between operands could be determined using other flags.
- The “cmpl” instruction compares values that are double word.
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please look at the entire text below. Please solve and show all work. Thank you.
What is the corresponding MIPS assembly code for the following C statement? Assume that the variables f, g, h, i, and j are assigned to register $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively.
B[8] = A[i−j]
Translate the following C code to MIPS. Assume that the variables f, g, h, i, and j are assigned to register $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. Assume that the elements of the arrays A and B are 8-byte words:
B[8] = A[i] + A[j]
Assume that registers $s0 and $s1 hold the values 0x80000000 and 0xD0000000, respectively.
What is the value of $t0 for the following assembly code?
add $t0, $s0, $s1
Is the result in $t0 the desired result, or has there been an overflow?
For the contents of registers $s0 and $s1 as…
For the following C statement, what is the corresponding MIPS assembly code? Assume that the variables f, g, h, i, and j are assigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays (A and B) are in registers $s6 and $s7, respectively. Also, assume that A and B are arrays of words.
B[f-j] = B[i] + A[g]
In this exercise we compare the performance of 1-issue and 2-issue processors, taking into account program transformations that can be made to optimize for 2-issue execution. Problems in this exercise refer to the following loop(written in C):for(i=0;i!=j;i+=2)b[i]=a[i]–a[i+1];When writing MIPS code, assume that variables are kept in registers as follows, and that all registers except those indicated as Free are used to keep various variables, so they cannot be used for anything else.
i
j
a
b
c
Free
R5
R6
R1
R2
R3
R10,R11,R12
Translate this C code into MIPS instructions. Your translation should be direct, without rearranging instructions to achieve better performance.
Chapter 3 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Ch. 3.4 - Prob. 3.1PPCh. 3.4 - Prob. 3.2PPCh. 3.4 - Prob. 3.3PPCh. 3.4 - Prob. 3.4PPCh. 3.4 - Prob. 3.5PPCh. 3.5 - Prob. 3.6PPCh. 3.5 - Prob. 3.7PPCh. 3.5 - Prob. 3.8PPCh. 3.5 - Prob. 3.9PPCh. 3.5 - Prob. 3.10PP
Ch. 3.5 - Prob. 3.11PPCh. 3.5 - Prob. 3.12PPCh. 3.6 - Prob. 3.13PPCh. 3.6 - Prob. 3.14PPCh. 3.6 - Prob. 3.15PPCh. 3.6 - Prob. 3.16PPCh. 3.6 - Practice Problem 3.17 (solution page 331) An...Ch. 3.6 - Practice Problem 3.18 (solution page 332) Starting...Ch. 3.6 - Prob. 3.19PPCh. 3.6 - Prob. 3.20PPCh. 3.6 - Prob. 3.21PPCh. 3.6 - Prob. 3.22PPCh. 3.6 - Prob. 3.23PPCh. 3.6 - Practice Problem 3.24 (solution page 335) For C...Ch. 3.6 - Prob. 3.25PPCh. 3.6 - Prob. 3.26PPCh. 3.6 - Practice Problem 3.27 (solution page 336) Write...Ch. 3.6 - Prob. 3.28PPCh. 3.6 - Prob. 3.29PPCh. 3.6 - Practice Problem 3.30 (solution page 338) In the C...Ch. 3.6 - Prob. 3.31PPCh. 3.7 - Prob. 3.32PPCh. 3.7 - Prob. 3.33PPCh. 3.7 - Prob. 3.34PPCh. 3.7 - Prob. 3.35PPCh. 3.8 - Prob. 3.36PPCh. 3.8 - Prob. 3.37PPCh. 3.8 - Prob. 3.38PPCh. 3.8 - Prob. 3.39PPCh. 3.8 - Prob. 3.40PPCh. 3.9 - Prob. 3.41PPCh. 3.9 - Prob. 3.42PPCh. 3.9 - Practice Problem 3.43 (solution page 344) Suppose...Ch. 3.9 - Prob. 3.44PPCh. 3.9 - Prob. 3.45PPCh. 3.10 - Prob. 3.46PPCh. 3.10 - Prob. 3.47PPCh. 3.10 - Prob. 3.48PPCh. 3.10 - Prob. 3.49PPCh. 3.11 - Practice Problem 3.50 (solution page 347) For the...Ch. 3.11 - Prob. 3.51PPCh. 3.11 - Prob. 3.52PPCh. 3.11 - Practice Problem 3.52 (solution page 348) For the...Ch. 3.11 - Practice Problem 3.54 (solution page 349) Function...Ch. 3.11 - Prob. 3.55PPCh. 3.11 - Prob. 3.56PPCh. 3.11 - Practice Problem 3.57 (solution page 350) Function...Ch. 3 - For a function with prototype long decoda2(long x,...Ch. 3 - The following code computes the 128-bit product of...Ch. 3 - Prob. 3.60HWCh. 3 - In Section 3.6.6, we examined the following code...Ch. 3 - The code that follows shows an example of...Ch. 3 - This problem will give you a chance to reverb...Ch. 3 - Consider the following source code, where R, S,...Ch. 3 - The following code transposes the elements of an M...Ch. 3 - Prob. 3.66HWCh. 3 - For this exercise, we will examine the code...Ch. 3 - Prob. 3.68HWCh. 3 - Prob. 3.69HWCh. 3 - Consider the following union declaration: This...Ch. 3 - Prob. 3.71HWCh. 3 - Prob. 3.72HWCh. 3 - Prob. 3.73HWCh. 3 - Prob. 3.74HWCh. 3 - Prob. 3.75HW
Knowledge Booster
Similar questions
- [Note: You are allowed to use only instructions implemented by the actual MIPS hardwareprovided in attached photos below. Use assembly language format from the references orthe book. Note, base ten numbers are listed as normal (e.g. 23), binary numbers areprefixed with 0b and hexadecimal numbers are prefixed with 0x.] Write a C program and corresponding assembly program based on MIPS ISA that reads three edges for a triangle and computes the perimeter if the input is valid. Otherwise, display that the input is invalid. The input is valid if the sum of every pair of two edges is greater than the remaining edge. [Direction: You can consult any resources such as books, online references, and videosfor this assignment, however, you have to properly cite and paraphrase your answerswhen it is necessary.] solve it any how urgently please.arrow_forwardFor the MIPS assembly instructions below, what is thecorresponding C statement? Assume that the variables f, g, h, i, and j areassigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume thatthe base address of the arrays A and B are in registers $s6 and $s7,respectively. Note: for each line of MIPS code below, write the respective Ccode. After that, write the corresponding C code for the MIPS.sll $t0, $s0, 2add $t0, $s6sll $t1, $s1, 2 add $t1, $s7, $t1lw $s0, 0($t0)addi $t2, $t0, 4lw $t0, 0($t2)add $t0, $t0, $s0sw $t0, 0($t1)arrow_forwardPlease solve and show all work. For the following C statement, what is the corresponding MIPS assembly code? Assume that the variables f, g, h, i, and j are assigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. Assume that the elements of the arrays A and B are 8-byte words: f = (g+i+2) + (h − 8); B[8] = A[i-9] + A[j+8] + 7;arrow_forward
- For the contents of registers $s0 and $s1 as specified above, what is the value of $t0 for the following assembly code? sub $t0, $s0, $s1arrow_forward(a) Write an assembly language program for the Intel 8086 microprocessor that divides a 32-bit number by a 16-bit word and stores the results i.e., quotient and reminder, in separate memory locations? (Choose the dividend and divisor by yourself).In the assembly language program, make sure to properly define the different segments using the appropriate assembler directives.(b) Draw an appropriate flow diagram for this program.arrow_forwardNASM CODE, modify the code below Write an assembly program for the processor family x86-32 that reads three signed interger numbers from the standard input and writes the greatest of them on the console. Use the C functions scanf and printf for the management of data input and data output. section .datamsg db "The largest number is: %d", 0xa,0v1 equ -47v2 equ 32v3 equ 21num1 times 4 db 0num2 times 4 db 0num3 times 4 db 0 section .textextern printfglobal main ;must be declared for using gcc main: ;tell linker entry point mov dword [num1], v1mov dword [num2], v2mov dword [num3], v3 mov ecx, [num1]cmp ecx, [num2]jg check_third_nummov ecx, [num2]check_third_num: cmp ecx, [num3]jg _exitmov ecx, [num3]_exit:;Print the greatest numberpush ecxpush msgcall printf ;Exit process mov eax, 1int 80harrow_forward
- For your discussion question response, please provide a response to each of the following questions. Make sure that you include the question followed by your answer to the question in your posting. In the description of the Hack machine language in chapter 4, it is stated that in well-written programs a C-instruction that may cause a jump should not contain a reference to M, and vice versa. Discuss why this should be avoided. Research the concept of interrupts in a computer. You may want to look into how the Z80 microprocessor handles interrupt. A good source of Z80 information is Zaks, R. (1980). Programming the Z80. Sybex. Download the pdf. FYI, The Z80 8 bit microprocessor has been in production for forty years. Knowledge of the Z80 is a great lead-in for further study in more complicated machines.arrow_forward(ASM) For the following C statement, what is the corresponding MIPS assembly code? Assume that the variables f, g, h, i, and j are assigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. B[f] = A[(i-h)+j] + g;arrow_forwardb) Machine cycle defines a loop process with four major components. Explain why machine cycle is important? If the components of machine cycle are inter-swapped, would that cause any problems? Can we add another module to rectify the problem caused by inter-swapping? c) Cache memory and RAM both are based on transistor based then why cache memory is needed if we already have RAM (Random Access Memory) as a volatile memory? Is it possible to deploy any one type of memory in computer for all purposes?arrow_forward
- To demonstrate each of the addressing modes presented in this chapter, translate the following pseudo code into MIPS assembly. Because variables x and y are static and volatile, they should be kept in data memory. You do not need to save the register while utilising registration direct access. variables in memory.main() { static volatile int miles = prompt("Enter the number of miles driven: "); static volatile int gallons = prompt("Enter the number of gallons used: "); static volatile int mpg = miles / gallons; output("Your mpg = " + mpg);}arrow_forwardTo demonstrate each of the addressing modes presented in this chapter, translate the following pseudo code into MIPS assembly. Because variables x and y are static and volatile, they should be kept in data memory. You do not need to keep variables in memory when utilising register direct access. main() { static volatile int miles = prompt("Enter the number of miles driven: "); static volatile int gallons = prompt("Enter the number of gallons used: "); static volatile int mpg = miles / gallons; output("Your mpg = " + mpg);}arrow_forward01 : (a) Write an assembly language program for the Intel 8086 microprocessor that adds two 16-bit words in the memory locations called ADD1 and ADD2, respectively, and stores the result in a memory location SUM? In the assembly language program, make sure to properly define the different segments using the appropriate assembler directives. (b) Draw a diagram showing the data arrangement in memory for the multiply program you wrote in section (a)?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
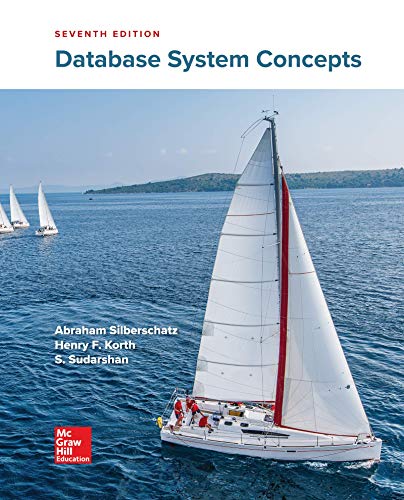
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
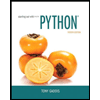
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
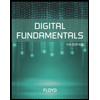
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
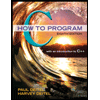
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
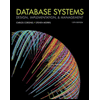
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
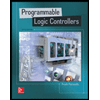
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education