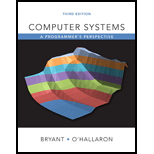
Computer Systems: A Programmer's Perspective (3rd Edition)
3rd Edition
ISBN: 9780134092669
Author: Bryant, Randal E. Bryant, David R. O'Hallaron, David R., Randal E.; O'Hallaron, Bryant/O'hallaron
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 3, Problem 3.73HW
Explanation of Solution
Given assembly code:
x in %xmm0
find_range:
vxorps %xmm1, %xmm1, %xmm1
vucomiss %xmm1, %xmm0
ja .L5
vucomiss %xmm1, %xmm0
jp .L8
movl $1, %eax
je .L3
.L8:
vucomiss %xmm1, %xmm0
setbe %al
movzbl %al, %eax
addl $2, %eax
ret
.L5:
movl $0, %eax
.L3:
Rep;ret
Explanation:
- The assembly function “find_range” illustrates conditional branching in floating point.
- It compares a value “x” with 0.
- If value is less than 0, then result is negative.
- If value is greater than 0, then result is positive.
- If value equals 0, then result is zero.
- The instruction “vxorps %xmm1, %xmm1, %xmm1” sets value of “%xmm1” to 0.
- The instruction “vucomiss %xmm1, %xmm0” compares values.
- The instruction “jp .L8” jumps to label “.L8”.
- The instruction “ja .L5” jumps to label “.L5” if first value is greater than second.
- The instruction “movl $0, %eax” moves immediate value to register “%eax”.
- The instruction “movl $1, %eax” moves immediate value to register “%eax”.
Function find_range:
#Define function
range_t find_range(float x) {
__asm__(
"vxorps %xmm1, %xmm1, %xmm1\n\t"
"vucomiss %xmm1, %xmm0\n\t"
"jp .P\n\t"
"ja .A\n\t"
"jb .B\n\t"
"je .E\n\t"
"...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
[Note: You are allowed to use only instructions implemented by the actual MIPS hardwareprovided in attached photos below. Use assembly language format from the references orthe book. Note, base ten numbers are listed as normal (e.g. 23), binary numbers areprefixed with 0b and hexadecimal numbers are prefixed with 0x.]
Write a C program and corresponding assembly program based on MIPS ISA that reads three edges for a triangle and computes the perimeter if the input is valid. Otherwise, display that the input is invalid. The input is valid if the sum of every pair of two edges is greater than the remaining edge.
[Direction: You can consult any resources such as books, online references, and videosfor this assignment, however, you have to properly cite and paraphrase your answerswhen it is necessary.] solve it any how urgently please.
Compile the following C code snippet with optimization level O0 and O1 using armv7-a clang 11.0.1 compiler.
Which instruction(s) computes the return value of the function in O2? Compare and explain the difference between the three optimization levels.
float foo1() {
float sum = 0.0;
unsigned int i = 0;
for (i = 1; i <= 15; i++) {
sum += i * i * i;
}
return sum;
}
2
– Find C, and Z flags after executing the compare instruction in each of the following codes:
(c) LDI R20, $3F
CPI R20, $3F
Chapter 3 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Ch. 3.4 - Prob. 3.1PPCh. 3.4 - Prob. 3.2PPCh. 3.4 - Prob. 3.3PPCh. 3.4 - Prob. 3.4PPCh. 3.4 - Prob. 3.5PPCh. 3.5 - Prob. 3.6PPCh. 3.5 - Prob. 3.7PPCh. 3.5 - Prob. 3.8PPCh. 3.5 - Prob. 3.9PPCh. 3.5 - Prob. 3.10PP
Ch. 3.5 - Prob. 3.11PPCh. 3.5 - Prob. 3.12PPCh. 3.6 - Prob. 3.13PPCh. 3.6 - Prob. 3.14PPCh. 3.6 - Prob. 3.15PPCh. 3.6 - Prob. 3.16PPCh. 3.6 - Practice Problem 3.17 (solution page 331) An...Ch. 3.6 - Practice Problem 3.18 (solution page 332) Starting...Ch. 3.6 - Prob. 3.19PPCh. 3.6 - Prob. 3.20PPCh. 3.6 - Prob. 3.21PPCh. 3.6 - Prob. 3.22PPCh. 3.6 - Prob. 3.23PPCh. 3.6 - Practice Problem 3.24 (solution page 335) For C...Ch. 3.6 - Prob. 3.25PPCh. 3.6 - Prob. 3.26PPCh. 3.6 - Practice Problem 3.27 (solution page 336) Write...Ch. 3.6 - Prob. 3.28PPCh. 3.6 - Prob. 3.29PPCh. 3.6 - Practice Problem 3.30 (solution page 338) In the C...Ch. 3.6 - Prob. 3.31PPCh. 3.7 - Prob. 3.32PPCh. 3.7 - Prob. 3.33PPCh. 3.7 - Prob. 3.34PPCh. 3.7 - Prob. 3.35PPCh. 3.8 - Prob. 3.36PPCh. 3.8 - Prob. 3.37PPCh. 3.8 - Prob. 3.38PPCh. 3.8 - Prob. 3.39PPCh. 3.8 - Prob. 3.40PPCh. 3.9 - Prob. 3.41PPCh. 3.9 - Prob. 3.42PPCh. 3.9 - Practice Problem 3.43 (solution page 344) Suppose...Ch. 3.9 - Prob. 3.44PPCh. 3.9 - Prob. 3.45PPCh. 3.10 - Prob. 3.46PPCh. 3.10 - Prob. 3.47PPCh. 3.10 - Prob. 3.48PPCh. 3.10 - Prob. 3.49PPCh. 3.11 - Practice Problem 3.50 (solution page 347) For the...Ch. 3.11 - Prob. 3.51PPCh. 3.11 - Prob. 3.52PPCh. 3.11 - Practice Problem 3.52 (solution page 348) For the...Ch. 3.11 - Practice Problem 3.54 (solution page 349) Function...Ch. 3.11 - Prob. 3.55PPCh. 3.11 - Prob. 3.56PPCh. 3.11 - Practice Problem 3.57 (solution page 350) Function...Ch. 3 - For a function with prototype long decoda2(long x,...Ch. 3 - The following code computes the 128-bit product of...Ch. 3 - Prob. 3.60HWCh. 3 - In Section 3.6.6, we examined the following code...Ch. 3 - The code that follows shows an example of...Ch. 3 - This problem will give you a chance to reverb...Ch. 3 - Consider the following source code, where R, S,...Ch. 3 - The following code transposes the elements of an M...Ch. 3 - Prob. 3.66HWCh. 3 - For this exercise, we will examine the code...Ch. 3 - Prob. 3.68HWCh. 3 - Prob. 3.69HWCh. 3 - Consider the following union declaration: This...Ch. 3 - Prob. 3.71HWCh. 3 - Prob. 3.72HWCh. 3 - Prob. 3.73HWCh. 3 - Prob. 3.74HWCh. 3 - Prob. 3.75HW
Knowledge Booster
Similar questions
- For the following C statement, write the correspondingRISC-V assembly code. Assume that the variables f, g, h, i, and j are assigned toregisters x5, x6, x7, x28, and x29, respectively. Assume that the base address ofthe arrays A and B are in registers x10 and x11, respectively. B[8] = A[i−j];arrow_forward[8] A C program containing the function food has been compiled into LC-3 assembly language. The partial translation of the function is:food:ADD R6, R6, #2STR R7, R6, #0ADD R6, R6, #1STR R5, R6, #0 ADD R5, R6, #1 ADD R6, R6, #4...How many local variables does this function have?a. 3b. 4c. 5d. 6arrow_forwardHere is my question from homework: int x; short y, z; cin>>x; y=x; z= y+2; cout<<"First number is: "<<x<<endl; cout<<"Second number is: "<<y<<endl; cout<<"Third number is: "<<z<<endl; End of HW problem My question is how do I utilize short variable type in MIPS assembly code and get it to work with int data type?arrow_forward
- For the following C statement, what is the corresponding MIPS assembly code? Assume that the variables f, g, h, i, and j are assigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays (A and B) are in registers $s6 and $s7, respectively. Also, assume that A and B are arrays of words. B[f-j] = B[i] + A[g]arrow_forwardModify the code below: Add code to display both sum and diff in a hexadecimal format on the screen, all bytes must be stored in a reversed sequence order. code: .386 ; Tells MASM to use Intel 80386 instruction set..MODEL FLAT ; Flat memory modeloption casemap:none ; Treat labels as case-sensitive INCLUDE IO.H ; header file for input/output .STACK 100h ; (default is 1-kilobyte stack) .const ; Constant data segment .DATA ; Begin initialized data segment op1 QWORD 0A2B2A40675981234h ; first 64-bit operand for additionop2 QWORD 08010870001234502h ; second 64-bit operand for addition sum DWORD 3 dup(?) ; 96-bit sum = ????????????????????????h op3 DWORD 2h, 0h, 0h ; 96-bit operand to subtract: 20000000200000002h .CODE ; Begin code segment_main PROC ; Beginning of code;-----------------------------------------------------------------------------; add two 64 bit numbers and store the result as 96 bit sum;-----------------------------------------------------------------------------mov EAX,…arrow_forwardQ2. Refer to datapath design on slide no. 26 with added blocks for jump instructions as shown inslide 33 in Chapter 4 (part 1). Let’s assume a program has 500 instructions. These instructions aredistributed as follows:R-Type Immediatearithmetic(addi)Load Store Branch Jump25% 5% 20% 20% 10% 20%Answer the following questions (show calculations):a) How many instructions will use instruction memory?b) How many instructions will use data memory?c) How many instructions will use the sign extend block?d) In the clock cycles, where the sign extend block is not required, does it remain idle? If yes,how? If not, what happens to the output of the block in that cycle? chapter 4, slide 33 is added as an image needed to solve the questionarrow_forward
- Rearrange the following code to minimize the total number of cycles, assuming that a dependent instruction following the load will need two clock cycles of delay before getting the data. Load r1, 64 (r2)Add r2, r2, r2Sub r3, r4, r1Load r4, 32 (r4)arrow_forwardConvert the following C++ program into an x86 assembly language programYour output must match the expected output given below for full points consideration // Expected output:// <<Bradley Sward>>'s solution to problem one// -13 -4 +21 +64 +7 +8// Press any key to continue... #include <iostream>using namespace std; // Global variablesint a = -5;int b = 2;int c = 7;int d;int e[]{ 5, 4, 1, 9, 8, 7 }; void main(){ // Replace <Bradley Sward> with your name please cout << "<<Bradley Sward>>'s solution to problem one" << endl; b = -c + 6 - c + a; cout << b << " "; a++; cout << a << " "; d = a - 11 + c + 9 - b + c; cout << d << " "; a = 44 - b + c; cout << a << " "; d = c; cout << d << " "; cout << e[4] << endl; system("PAUSE");}arrow_forward1.3 Assemble the following assembly code into machine code. Assume that the machine language op-codes for load, store, mult, add, div, and sub are 18, 19, 13, 14, 15, and 16, respectively. Also assume that the variable x is stored at location M[50]. load R1, x mult R2, R1, #9 store x, R2 sub R0, R1, #8 div R2, R0, #2arrow_forward
- (ASM) For the following C statement, what is the corresponding MIPS assembly code? Assume that the variables f, g, h, i, and j are assigned to registers $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. B[f] = A[(i-h)+j] + g;arrow_forwardPlease look at the entire text below. Please solve and show all work. Thank you. What is the corresponding MIPS assembly code for the following C statement? Assume that the variables f, g, h, i, and j are assigned to register $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. B[8] = A[i−j] Translate the following C code to MIPS. Assume that the variables f, g, h, i, and j are assigned to register $s0, $s1, $s2, $s3, and $s4, respectively. Assume that the base address of the arrays A and B are in registers $s6 and $s7, respectively. Assume that the elements of the arrays A and B are 8-byte words: B[8] = A[i] + A[j] Assume that registers $s0 and $s1 hold the values 0x80000000 and 0xD0000000, respectively. What is the value of $t0 for the following assembly code? add $t0, $s0, $s1 Is the result in $t0 the desired result, or has there been an overflow? For the contents of registers $s0 and $s1 as…arrow_forwardPlease solve and show all work and steps. For the following C statement, write a minimal sequence of MIPS assembly instructions that does the identical operation. Assume $t1 = A, $t2 = B, and $s1 is the base address of C, << is shift left operation. A = C[1] >> 12;arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
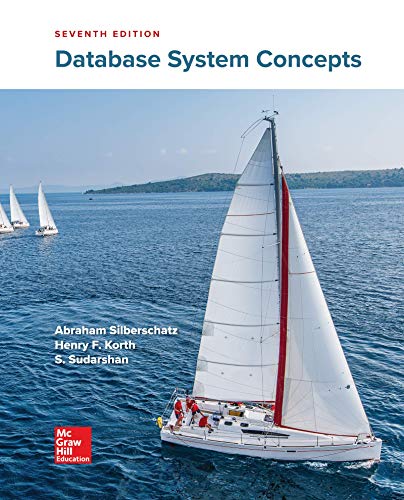
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
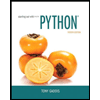
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
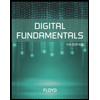
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
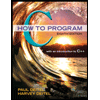
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
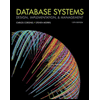
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
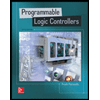
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education