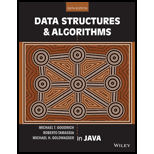
Concept explainers
Explanation of Solution
a.
Simple method to compute p(x):
Let us consider the “p(x)” polynomial of degree “n”.
The method to compute the polynomial of degree is given below:
//Define the simplePolynomial() method
public static double simplePolynomial(double a[], double n)
{
//Declare the variable
double sum = 0;
//Loop executes until the length of array
for(int i=0; i<a.length; i++)
//Compute the polynomial of degree
sum = sum + a[i] * Math.pow(n, a.length - i - 1);
//Return the polynomial of degree
return sum;
}
Running time:
In the above code, the computation of polynomial of degree is given below:
- For each and every array term,
- a[0] is “0” operation.
- a[1]× x is “1” operation.
- a[2]× x × x is “2” operations.
- a[3]× x × x × x is “3” operations
Explanation of Solution
b.
Method to compute p(x) in O(n(logn)) time:
Let us consider the “p(x)” polynomial of degree “n”.
The method to compute the polynomial of degree is given below:
//Define the Polynomial() method
public static double Polynomial(double a[], double n)
{
//Declare the variable
double sum = 0;
int i=0;
//Loop executes until the length of array
while(i<a.length)
{
//Compute the polynomial of degree
sum = sum + a[i] * Math.pow(n, a.length - i - 1);
//Increment "i" by "1"
i = i++;
}
//Return the polynomial of degree
return sum;
}
Running time:
In the above code, the computation of polynomial of degree is given below:
- For each and every array term,
- a[0] is “0” operation.
- a[1]× x is “1” operation
Explanation of Solution
c.
Horner method to compute p(x):
Let us consider the “p(x)” polynomial of degree “n”.
The method to compute the polynomial of degree is given below:
//Define the HornerPolynomial() method
public static double HornerPolynomial(double a[],double n)
{
//Declare the variable
double sum = 0;
//Loop executes until the length of array
for(int i=1;i< a
Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Data Structures and Algorithms in Java
- Please solve and answer the questions correctly please. Thank you!!arrow_forwardConsidering the TM example of binary sum ( see attached)do the step-by-step of execution for the binary numbers 1101 and 11. Feel free to use the Formal Language Editor Tool to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step.arrow_forwardI need help on inculding additonal code where I can can do the opposite code of MatLab, where the function of t that I enter becomes the result of F(t), in other words, turning the time-domain f(t) into the frequency-domain function F(s):arrow_forward
- I need help with the TM computation step-by-step execution for the binary numbers 1101 and 11. Formal Language Editor Tool can be used to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step;arrow_forwardWriting an introduction manual for software designarrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
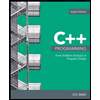
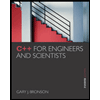
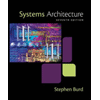
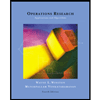
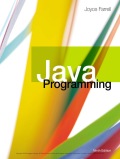