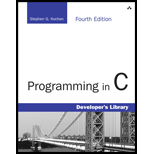
Concept explainers

Explanation of Solution
Modified Program:
The following program will ask the user to enter the total number of triangular they want and calculate the triangular number using nested “for” condition:
//include the header file
#include <stdio.h>
//definition of main method
int main (void)
{
//declare the variables
int n, number, triangularNumber, counter, count;
//get the input from the user
printf("How many triangular would user want?");
scanf("%i", &count);
//check the condition
for (counter = 1; counter <=count; ++counter)
{
//get the input from the user
printf("What triangular number do you want? ");
scanf("%i", &number);
//set the value
triangularNumber = 0;
//check the condition
for (n = 1; n <=number; ++n)
//calculate the "triangularNumber" value
triangularNumber = triangularNumber + n;
//display the result
printf("Triangular number %i is %i\n", number, triangularNumber);
}
//return statement
return 0;
}
Explanation:
In the above program, declare the required header file. Inside the main method, declare the necessary variables and ask the user to enter the total number of triangular they want. The “for” condition is used to get the input from the user then nested “for” condition is used to iterate the “n” value up to the user entered input and inside the loop calculate the triangular of “n” value and display the result on the output screen.
How many triangular would user want? 2
What triangular number do you want? 12
Triangular number 12 is 78
What triangular number do you want? 25
Triangular number 25 is 325
Want to see more full solutions like this?
Chapter 4 Solutions
Programming in C
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- Write a program that displays two chessboards, as shown in Figure 6.13. Your program should define at least the following function:# Draw one chessboard whose upper-left corner is at# (startx, starty) and bottom-right corner is at (endx, endy)def drawChessboard(startx, endx, starty, endy):arrow_forwardwhat sequence of numbers would be printed if the following function were executed with the value of N being 0? def xxx (N): print (N) if (N < 5): xxx (N + 2) print (N)arrow_forwardActivity 2 7.) Write a program that will calculate and print out bills for the city water company. The water rates vary depending on whether the water is for home use, commercial use or industrial use. A code of H means home use, a code of c means commercial use and a code of I means industrial use. The water rates are computed as follows: code H: P250.00 plus P0.002 per gallon used code c: P5,000.00 for the first 4 million gallons and P0.002 for each additional gallon. code I: P8,000 if usage does not exceed 4 million gallons, P14,000 if usage is more than 4 million gallons but not more than 10 million gallons and P18000 if usage exceeds 10 million gallons. Your program should prompt the user for the code and the gallons of water used. Your program should echo your input data and should print the amount due from the user. Your program should use a switch statement for the code (char data type). Use the float data type for the gallons. Sample Output in the images:arrow_forward
- 3. int x =9 ;display the address of the variable x? Answer:arrow_forwardWrite a C++ that Ask user to enter an integer number and then determine whether the number is prime or not? Note: a number is prime that it is only divisible by 1 and itself. such as 3,5,7,13,..arrow_forwardThis Code print the first occurrences of x, Modify the code to print the last occurrences of x and print how many times it exists? ( with explanation for each line if possible) many thanks.arrow_forward
- C++ Question, Write a Computer Code: Let l be a line in the x-y plane. If l is a vertical line, its equation is x = a for some real number a. Suppose l is not a vertical line and its slope is m. Then the equation of l is y = mx + b, where b is the y-intercept. If l passes through the point (x₀, y₀), the equation of l can be written as y - y₀ = m(x - x₀). If (x₁, y₁) and (x₂, y₂) are two points in the x-y plane and x₁ ≠ x₂, the slope of line passing through these points is m = (y₂ - y₁)/(x₂ - x₁).arrow_forwardTrace the following code and give the output int a = 6, b = 8; int c = (a < b) ? a : b; int v = c; while (v%a != 0 || v%b != 0) v += c; cout << v;arrow_forwardWrite a program using function to check if the number is even or odd?Write a program to return the product of digits of given number.Write a program to find the sum of given 2 numbers.Write a program to find the max of two given numbers.arrow_forward
- Hi, I need help with the following assignment. Implement a program that reads in a year and outputs the approximate value of a Ferrari 250 GTO in that year. Use the following table that describes the estimated value of a GTO at different times since 1962. Year Value 1962-1964 $18,500 1965-1968 $6,000 1969-1971 $12,000 1972-1975 $48,000 1976-1980 $200,000 1981-1985 $650,000 1986-2012 $35,000,000 2013-2014 $52,000,000arrow_forwardWrite a program that does temperature converter :\n", "- The user will enter if he wants the result in Celsius or in Fahrenheit. Use the input function to read this option. \n", "- Ask the user the value of the temperature he wants to convert. Use the input function to read this value and convert accordingly.\n", "- If the user wants the temperature in Fahrenheit compute: $ Fahrenheit = Celsius*5/9+32$.\n", "- If the user wants the Celsius compute as follows: $ C = (F-32)*5/9 $.\n"arrow_forwardI). Write a program in c++ with class that will help an elementary school student learn multiplication. Use the rand function to produce two positive one-digit integers. The program should then prompt the user with a question, such as How much is 6 times 7? The student then inputs the answer. Next, the program checks the student’s answer. If it’s correct, display the message "Very good!" and ask another multiplication question. If the answer is wrong, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. A separate function should be used to generate each new question. This function should be called once when the application begins execution and each time the user answers the question correctly.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
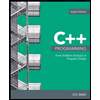